mirror of
https://github.com/Polprzewodnikowy/SummerCart64.git
synced 2025-01-25 02:41:10 +01:00
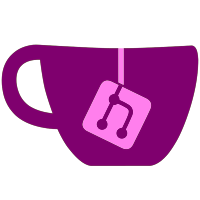
* isv support + usb/dd improvements * make room for saves * update offset * fixed debug address * idk * exception * ironed out all broken stuff * cleanup * return epc fix * better * more cleanup * even more cleanup * mooore cleanup * fixed printf * no assert * improved docker build, pyft232 instead of pyserial * fixed displaying long message strings description test * just straight cleanup * smallest cleanup * PAL * cpu buffer * n64 bootloader done * super slow usb storage reading implemented * reduced buffer size * usb gets fast * little cleanup * double buffered reads * removed separate event id * ISV in hardware finally * small exception changes * mac testing * py spacing * fsd write, rtc, isv and reset fixes * fixxx * good stopping point * usb fixed? * pretend we have 128 MB sdram * backup * chmod * test * test done * more tests * user rm * help * final fix * updated component values * nice asset names * cic 64dd support * ddipl enable separation * pre DMA rewrite, created dedicated buffer memory space, simplified code * dma rewrite, needs testing * moved xml * dd basics * timing * 64dd working yet again, isv brought back, dma fixes, usb path rewrite, pc code rewrite * added usb read functionality, general cleanup * changed mem addressing * added fpga flash update access * added mcu update * chmod * little cleanup * update format and stuff * fixes * uninitialized fix * small fixes * update fixes * update stuff done * fpga update tested * build time fix * boot fix * test timing * readme test * test 2 * reports * testseet * final * build test * forgot * button and naming * General cleanup And multiline commit message test * Exception screen UI touch ups * display separation and tests beginning * pc software update * pc software done * timing test * delete launch.json * sw fixes * fixed button hole diameter in shell * small cleanup, rpi testing * shell fillet fix, pc rtc printing * added cfg lock mechanism * moved lock to cfg address space * extended ROM and ISV fixes * preliminary sd card support * little sd card cleanup * sd menu fixes * 5 second limit * reduced shell thickness * basic led act blinking * faster sd menu loading * inst cache invalidate * sd card writing is working * SD card CSD and CID registers * wait for previous command * led error codes * fixed cfg_translate_address use * 64dd from sd card working * 64dd speedup and button handling * delayed address latching cycle - might break other builds, needs testing * bootloader improvements * small fixes * return previous cfg when setting new * cache stuff * unfloader debug protocol support * UNFLoader style debug command line support * requirements.txt * shell groove fillet * reset state inside controller * fixed fast PI read, added PI R/W fifo debug info * PI access prioritize * SD clock stop when RX FIFO is more than half full * flash erase method change * CFG error handling, TLOZ MM debug ISV support * CIC5167 support * general fixes * USB unplugged cable handling * turn off led when changing between error/act modes * rtc 2 bit clock stop support * line endings * Revert "line endings" This reverts commit d0ddfe5ec716d2db7c72561703f51a94bf34e6bb. * PI address debug * readme test * diagram update * diagram background * diagram background * diagram background * updated readme
84 lines
3.7 KiB
C
84 lines
3.7 KiB
C
#include <stdarg.h>
|
|
#include "display.h"
|
|
#include "exception_regs.h"
|
|
#include "exception.h"
|
|
#include "io.h"
|
|
#include "version.h"
|
|
#include "vr4300.h"
|
|
#include "../assets/assets.h"
|
|
|
|
|
|
#define EXCEPTION_INTERRUPT (0)
|
|
#define EXCEPTION_SYSCALL (8)
|
|
|
|
#define INTERRUPT_MASK_TIMER (1 << 7)
|
|
|
|
#define SYSCALL_CODE_MASK (0x03FFFFC0UL)
|
|
#define SYSCALL_CODE_BIT (6)
|
|
|
|
|
|
static const char *exception_get_description (uint8_t exception_code) {
|
|
switch (exception_code) {
|
|
case 0: return "Interrupt";
|
|
case 1: return "TLB Modification exception";
|
|
case 2: return "TLB Miss exception (load or instruction fetch)";
|
|
case 3: return "TLB Miss exception (store)";
|
|
case 4: return "Address Error exception (load or instruction fetch)";
|
|
case 5: return "Address Error exception (store)";
|
|
case 6: return "Bus Error exception (instruction fetch)";
|
|
case 7: return "Bus Error exception (data reference: load or store)";
|
|
case 8: return "Syscall exception";
|
|
case 9: return "Breakpoint exception";
|
|
case 10: return "Reserved Instruction exception";
|
|
case 11: return "Coprocessor Unusable exception";
|
|
case 12: return "Arithmetic Overflow exception";
|
|
case 13: return "Trap exception";
|
|
case 15: return "Floating-Point exception";
|
|
case 23: return "Watch exception";
|
|
}
|
|
|
|
return "Unknown exception";
|
|
}
|
|
|
|
|
|
void exception_fatal_handler (uint32_t exception_code, uint32_t interrupt_mask, exception_t *e) {
|
|
version_t *version = version_get();
|
|
uint32_t *instruction_address = (((uint32_t *) (e->epc.u32)) + ((e->cr & C0_CR_BD) ? 1 : 0));
|
|
|
|
display_init((uint32_t *) (&assets_sc64_logo_640_240_dimmed));
|
|
|
|
display_printf("branch: %s | tag: %s\n", version->git_branch, version->git_tag);
|
|
display_printf("sha: %s\n", version->git_sha);
|
|
display_printf("%s\n\n", version->git_message);
|
|
|
|
display_printf("%s\n", exception_get_description(exception_code));
|
|
display_printf(" pc: 0x%08lX sr: 0x%08lX cr: 0x%08lX va: 0x%08lX\n", e->epc.u32, e->sr, e->cr, e->badvaddr.u32);
|
|
display_printf(" zr: 0x%08lX at: 0x%08lX v0: 0x%08lX v1: 0x%08lX\n", e->zr.u32, e->at.u32, e->v0.u32, e->v1.u32);
|
|
display_printf(" a0: 0x%08lX a1: 0x%08lX a2: 0x%08lX a3: 0x%08lX\n", e->a0.u32, e->a1.u32, e->a2.u32, e->a3.u32);
|
|
display_printf(" t0: 0x%08lX t1: 0x%08lX t2: 0x%08lX t3: 0x%08lX\n", e->t0.u32, e->t1.u32, e->t2.u32, e->t3.u32);
|
|
display_printf(" t4: 0x%08lX t5: 0x%08lX t6: 0x%08lX t7: 0x%08lX\n", e->t4.u32, e->t5.u32, e->t6.u32, e->t7.u32);
|
|
display_printf(" s0: 0x%08lX s1: 0x%08lX s2: 0x%08lX s3: 0x%08lX\n", e->s0.u32, e->s1.u32, e->s2.u32, e->s3.u32);
|
|
display_printf(" s4: 0x%08lX s5: 0x%08lX s6: 0x%08lX s7: 0x%08lX\n", e->s4.u32, e->s5.u32, e->s6.u32, e->s7.u32);
|
|
display_printf(" t8: 0x%08lX t9: 0x%08lX k0: 0x%08lX k1: 0x%08lX\n", e->t8.u32, e->t9.u32, e->k0.u32, e->k1.u32);
|
|
display_printf(" gp: 0x%08lX sp: 0x%08lX s8: 0x%08lX ra: 0x%08lX\n\n", e->gp.u32, e->sp.u32, e->s8.u32, e->ra.u32);
|
|
|
|
if (exception_code == EXCEPTION_INTERRUPT) {
|
|
if (interrupt_mask & INTERRUPT_MASK_TIMER) {
|
|
exception_disable_watchdog();
|
|
display_printf("Still loading after 5 second limit...\n\n");
|
|
return;
|
|
}
|
|
} else if (exception_code == EXCEPTION_SYSCALL) {
|
|
uint32_t code = (((*instruction_address) & SYSCALL_CODE_MASK) >> SYSCALL_CODE_BIT);
|
|
|
|
if (code == TRIGGER_CODE_ERROR) {
|
|
const char *fmt = (const char *) (e->a0.u32);
|
|
va_list args = *((va_list *) (e->sp.u32));
|
|
display_vprintf(fmt, args);
|
|
display_printf("\n");
|
|
}
|
|
}
|
|
|
|
while (1);
|
|
}
|