mirror of
https://github.com/Polprzewodnikowy/SummerCart64.git
synced 2024-11-25 23:24:15 +01:00
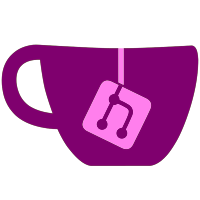
This PR completely removes `task.c / task.h` from `sw/controller` STM32 code. Additionally, these changes were implemented: - Updated IPL3 - Added new diagnostic data (voltage and temperature) readout commands for USB and N64 - Fixed some issues with FlashRAM save type - Joybus timings were relaxed to accommodate communication with unsynchronized master controller (like _Datel Game Killer_, thanks @RWeick) - N64 embedded test program now waits for release of button press to proceed - Fixed issue where, in rare circumstances, I2C peripheral in STM32 would get locked-up on power-up - LED blinking behavior on SD card access was changed - LED blink duration on save writeback has been extended - Minor fixes through the entire of hardware abstraction layer for STM32 code - Primer now correctly detects issues with I2C bus during first time programming - `primer.py` script gives more meaningful error messages - Fixed bug where RTC time was always written on N64FlashcartMenu boot - sc64deployer now displays "Diagnostic data" instead of "MCU stack usage"
100 lines
2.1 KiB
C
100 lines
2.1 KiB
C
#include <stdint.h>
|
|
#include "button.h"
|
|
#include "dd.h"
|
|
#include "fpga.h"
|
|
#include "usb.h"
|
|
|
|
|
|
#define BUTTON_COUNTER_TRIGGER_ON (64)
|
|
#define BUTTON_COUNTER_TRIGGER_OFF (0)
|
|
|
|
|
|
struct process {
|
|
uint8_t counter;
|
|
bool state;
|
|
button_mode_t mode;
|
|
bool trigger;
|
|
};
|
|
|
|
|
|
static struct process p;
|
|
|
|
|
|
bool button_get_state (void) {
|
|
return p.state;
|
|
}
|
|
|
|
bool button_set_mode (button_mode_t mode) {
|
|
if (mode >= __BUTTON_MODE_COUNT) {
|
|
return true;
|
|
}
|
|
p.mode = mode;
|
|
if (p.mode == BUTTON_MODE_NONE) {
|
|
p.trigger = false;
|
|
}
|
|
return false;
|
|
}
|
|
|
|
button_mode_t button_get_mode (void) {
|
|
return p.mode;
|
|
}
|
|
|
|
|
|
void button_init (void) {
|
|
p.counter = 0;
|
|
p.state = false;
|
|
p.mode = BUTTON_MODE_NONE;
|
|
p.trigger = false;
|
|
}
|
|
|
|
|
|
void button_process (void) {
|
|
usb_tx_info_t packet_info;
|
|
|
|
uint32_t status = fpga_reg_get(REG_CFG_SCR);
|
|
|
|
if (status & CFG_SCR_BUTTON_STATE) {
|
|
if (p.counter < BUTTON_COUNTER_TRIGGER_ON) {
|
|
p.counter += 1;
|
|
}
|
|
} else {
|
|
if (p.counter > BUTTON_COUNTER_TRIGGER_OFF) {
|
|
p.counter -= 1;
|
|
}
|
|
}
|
|
|
|
if (!p.state && p.counter == BUTTON_COUNTER_TRIGGER_ON) {
|
|
p.state = true;
|
|
p.trigger = true;
|
|
}
|
|
|
|
if (p.state && p.counter == BUTTON_COUNTER_TRIGGER_OFF) {
|
|
p.state = false;
|
|
}
|
|
|
|
if (p.trigger) {
|
|
switch (p.mode) {
|
|
case BUTTON_MODE_N64_IRQ:
|
|
fpga_reg_set(REG_CFG_CMD, CFG_CMD_IRQ);
|
|
p.trigger = false;
|
|
break;
|
|
|
|
case BUTTON_MODE_USB_PACKET:
|
|
usb_create_packet(&packet_info, PACKET_CMD_BUTTON_TRIGGER);
|
|
if (usb_enqueue_packet(&packet_info)) {
|
|
p.trigger = false;
|
|
}
|
|
break;
|
|
|
|
case BUTTON_MODE_DD_DISK_SWAP:
|
|
dd_handle_button();
|
|
p.trigger = false;
|
|
break;
|
|
|
|
default:
|
|
p.trigger = false;
|
|
break;
|
|
}
|
|
}
|
|
}
|