mirror of
https://github.com/Polprzewodnikowy/SummerCart64.git
synced 2024-11-22 14:09:16 +01:00
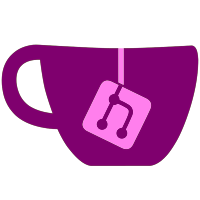
This PR completely removes `task.c / task.h` from `sw/controller` STM32 code. Additionally, these changes were implemented: - Updated IPL3 - Added new diagnostic data (voltage and temperature) readout commands for USB and N64 - Fixed some issues with FlashRAM save type - Joybus timings were relaxed to accommodate communication with unsynchronized master controller (like _Datel Game Killer_, thanks @RWeick) - N64 embedded test program now waits for release of button press to proceed - Fixed issue where, in rare circumstances, I2C peripheral in STM32 would get locked-up on power-up - LED blinking behavior on SD card access was changed - LED blink duration on save writeback has been extended - Minor fixes through the entire of hardware abstraction layer for STM32 code - Primer now correctly detects issues with I2C bus during first time programming - `primer.py` script gives more meaningful error messages - Fixed bug where RTC time was always written on N64FlashcartMenu boot - sc64deployer now displays "Diagnostic data" instead of "MCU stack usage"
91 lines
1.9 KiB
C
91 lines
1.9 KiB
C
#include "cic.h"
|
|
#include "fpga.h"
|
|
#include "hw.h"
|
|
#include "led.h"
|
|
#include "rtc.h"
|
|
|
|
|
|
typedef enum {
|
|
REGION_NTSC,
|
|
REGION_PAL,
|
|
__REGION_MAX
|
|
} cic_region_t;
|
|
|
|
|
|
static bool cic_error_active = false;
|
|
|
|
|
|
void cic_reset_parameters (void) {
|
|
cic_region_t region = rtc_get_region();
|
|
|
|
const uint8_t default_seed = 0x3F;
|
|
const uint64_t default_checksum = 0xA536C0F1D859ULL;
|
|
|
|
uint32_t cfg[2] = {
|
|
(default_seed << CIC_SEED_BIT) | ((default_checksum >> 32) & 0xFFFF),
|
|
(default_checksum & 0xFFFFFFFFUL)
|
|
};
|
|
|
|
if (region == REGION_PAL) {
|
|
cfg[0] |= CIC_REGION;
|
|
}
|
|
|
|
fpga_reg_set(REG_CIC_0, cfg[0]);
|
|
fpga_reg_set(REG_CIC_1, cfg[1]);
|
|
}
|
|
|
|
void cic_set_parameters (uint32_t *args) {
|
|
uint32_t cfg[2] = {
|
|
args[0] & (0x00FFFFFF),
|
|
args[1]
|
|
};
|
|
|
|
cfg[0] |= fpga_reg_get(REG_CIC_0) & (CIC_64DD_MODE | CIC_REGION);
|
|
|
|
if (args[0] & (1 << 24)) {
|
|
cfg[0] |= CIC_DISABLED;
|
|
}
|
|
|
|
fpga_reg_set(REG_CIC_0, cfg[0]);
|
|
fpga_reg_set(REG_CIC_1, cfg[1]);
|
|
}
|
|
|
|
void cic_set_dd_mode (bool enabled) {
|
|
uint32_t cfg = fpga_reg_get(REG_CIC_0);
|
|
|
|
if (enabled) {
|
|
cfg |= CIC_64DD_MODE;
|
|
} else {
|
|
cfg &= ~(CIC_64DD_MODE);
|
|
}
|
|
|
|
fpga_reg_set(REG_CIC_0, cfg);
|
|
}
|
|
|
|
|
|
void cic_init (void) {
|
|
cic_reset_parameters();
|
|
}
|
|
|
|
|
|
void cic_process (void) {
|
|
uint32_t cfg = fpga_reg_get(REG_CIC_0);
|
|
|
|
if (cfg & CIC_INVALID_REGION_DETECTED) {
|
|
cfg ^= CIC_REGION;
|
|
cfg |= CIC_INVALID_REGION_RESET;
|
|
fpga_reg_set(REG_CIC_0, cfg);
|
|
|
|
cic_region_t region = (cfg & CIC_REGION) ? REGION_PAL : REGION_NTSC;
|
|
rtc_set_region(region);
|
|
|
|
cic_error_active = true;
|
|
led_blink_error(LED_ERROR_CIC);
|
|
}
|
|
|
|
if (cic_error_active && (!hw_gpio_get(GPIO_ID_N64_RESET))) {
|
|
cic_error_active = false;
|
|
led_clear_error(LED_ERROR_CIC);
|
|
}
|
|
}
|