mirror of
https://github.com/Fledge68/WiiFlow_Lite.git
synced 2024-11-01 09:05:06 +01:00
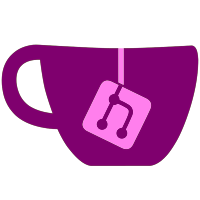
is found, it will just replace the filename extension with .jpg and try it again, the jpg file width and height need to be divisable with 4, otherwise wiiflow will just ignore the cover
35 lines
1.5 KiB
C++
35 lines
1.5 KiB
C++
|
|
#ifndef __TEXTURE_HPP
|
|
#define __TEXTURE_HPP
|
|
|
|
#include <gccore.h>
|
|
|
|
#include "smartptr.hpp"
|
|
|
|
struct STexture
|
|
{
|
|
SmartBuf data;
|
|
u32 width;
|
|
u32 height;
|
|
u8 format;
|
|
u8 maxLOD;
|
|
STexture(void) : data(), width(0), height(0), format(-1), maxLOD(0) { }
|
|
// Utility funcs
|
|
enum TexErr { TE_OK, TE_ERROR, TE_NOMEM };
|
|
// Get from PNG, if not found from JPG
|
|
TexErr fromImageFile(const char *filename, u8 f = -1, Alloc alloc = ALLOC_MEM2, u32 minMipSize = 0, u32 maxMipSize = 0);
|
|
// This function doesn't use MEM2 if the PNG is loaded from memory and there's no mip mapping
|
|
TexErr fromPNG(const u8 *buffer, u8 f = -1, Alloc alloc = ALLOC_MEM2, u32 minMipSize = 0, u32 maxMipSize = 0);
|
|
TexErr fromRAW(const u8 *buffer, u32 w, u32 h, u8 f = -1, Alloc alloc = ALLOC_MEM2, u32 minMipSize = 0, u32 maxMipSize = 0);
|
|
TexErr fromJPG(const u8 *buffer, const u32 buffer_size, u8 f = -1, Alloc alloc = ALLOC_MEM2, u32 minMipSize = 0, u32 maxMipSize = 0);
|
|
private:
|
|
static void _resize(u8 *dst, u32 dstWidth, u32 dstHeight, const u8 *src, u32 srcWidth, u32 srcHeight);
|
|
static void _resizeD2x2(u8 *dst, const u8 *src, u32 srcWidth, u32 srcHeight);
|
|
static SmartBuf _genMipMaps(const u8 *src, u32 width, u32 height, u8 maxLOD, u32 lod0Width, u32 lod0Height);
|
|
static void _calcMipMaps(u8 &maxLOD, u8 &minLOD, u32 &lod0Width, u32 &lod0Height, u32 width, u32 height, u32 minSize, u32 maxSize);
|
|
};
|
|
|
|
u32 fixGX_GetTexBufferSize(u16 wd, u16 ht, u32 fmt, u8 mipmap, u8 maxlod);
|
|
|
|
#endif //!defined(__TEXTURE_HPP)
|