mirror of
https://github.com/Fledge68/WiiFlow_Lite.git
synced 2024-11-01 00:55:06 +01:00
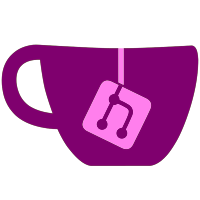
- added sorting by year released for all games. requires 'Reload Cache'. - added playcount and lastplayed for plugin games. B+PLUS to set sorting type (alphabet, playcount, lastplayed, players, and year. wifiplayers for wii games and game ID (1st letter) for VC games). B+up/down to next/prev sorted item. B on next/prev icons to next/prev sorted item.
116 lines
1.7 KiB
C
116 lines
1.7 KiB
C
|
|
#ifndef _DISC_H_
|
|
#define _DISC_H_
|
|
|
|
#define WII_MAGIC 0x5D1C9EA3
|
|
#define GC_MAGIC 0xC2339F3D
|
|
|
|
/* Disc header structure */
|
|
struct discHdr
|
|
{
|
|
/* Game ID */
|
|
u8 id[6];
|
|
|
|
/* Game version */
|
|
u16 version;
|
|
|
|
/* Audio streaming */
|
|
u8 streaming;
|
|
u8 bufsize;
|
|
|
|
/* Padding */
|
|
u64 chantitle; // Used for channels
|
|
|
|
/* Sorting */
|
|
u16 index;
|
|
u8 esrb;
|
|
u8 controllers;
|
|
u8 players;
|
|
u8 wifi;
|
|
|
|
/* Wii Magic word */
|
|
u32 magic;
|
|
|
|
/* GC Magic word */
|
|
u32 gc_magic;
|
|
|
|
/* Game title */
|
|
char title[64];
|
|
|
|
/* Encryption/Hashing */
|
|
u8 encryption;
|
|
u8 h3_verify;
|
|
|
|
/* Padding */
|
|
u32 casecolor;
|
|
u8 unused3[26];
|
|
} ATTRIBUTE_PACKED;
|
|
|
|
struct gc_discHdr
|
|
{
|
|
/* Game ID */
|
|
u8 id[6];
|
|
|
|
/* Game version */
|
|
u16 version;
|
|
|
|
/* Audio streaming */
|
|
u8 streaming;
|
|
u8 bufsize;
|
|
|
|
/* Padding */
|
|
u8 unused1[18];
|
|
|
|
/* Magic word */
|
|
u32 magic;
|
|
|
|
/* Game title */
|
|
char title[64];
|
|
|
|
/* Padding */
|
|
u8 unused2[64];
|
|
} ATTRIBUTE_PACKED;
|
|
|
|
struct dir_discHdr
|
|
{
|
|
char id[7]; //6+1 for null character
|
|
|
|
char path[256]; // full path including partion - path - rom.ext or game.iso or id6.wbfs
|
|
wchar_t title[64]; // wide character title used for displaying title in coverflow
|
|
u32 settings[2]; //chantitle, plugin magic, crc32, gamecube game on sd, etc
|
|
|
|
u8 type;
|
|
|
|
u32 casecolor;
|
|
u16 index;
|
|
u16 year;// year released
|
|
//u8 esrb;
|
|
//u8 controllers;
|
|
u8 players;
|
|
u8 wifi;
|
|
} ATTRIBUTE_PACKED;
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif /* __cplusplus */
|
|
|
|
/* Prototypes */
|
|
s32 Disc_Open(bool);
|
|
s32 Disc_Wait(void);
|
|
s32 Disc_ReadHeader(void *);
|
|
s32 Disc_ReadGCHeader(void *);
|
|
s32 Disc_Type(bool);
|
|
s32 Disc_IsWii(void);
|
|
s32 Disc_IsGC(void);
|
|
|
|
/* Headers for general usage */
|
|
extern struct discHdr wii_hdr;
|
|
extern struct gc_discHdr gc_hdr;
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif /* __cplusplus */
|
|
|
|
#endif
|
|
|