mirror of
https://github.com/Fledge68/WiiFlow_Lite.git
synced 2024-11-01 00:55:06 +01:00
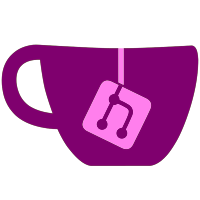
which has enough space left instead of fixed memory in most parts -fixed alot of things about banner playing, now shouldnt randomly codedump anymore or things like this, also some banner sounds which did not play before should work fine now -removed unused code and added better debug prints -using some fixed voice for game banner and not always a new one per banner -fixed DIOS-MIOS cheats for sure now :P -fixed possible memory allocation bug in wbfs alloc (thanks to cfg-loader) -removed MEM2_memalign since it did not work correctly -fixed a few wrong memset sizes
60 lines
1.6 KiB
C
60 lines
1.6 KiB
C
#include <string.h>
|
|
#include <malloc.h>
|
|
|
|
#include "gcard.h"
|
|
#include "http.h"
|
|
#include "utils.h"
|
|
#include "gecko/gecko.h"
|
|
|
|
#define MAX_URL_SIZE 178 // 128 + 48 + 6
|
|
|
|
struct provider
|
|
{
|
|
char url[128];
|
|
char key[48];
|
|
};
|
|
|
|
struct provider *providers = NULL;
|
|
int amount_of_providers = 0;
|
|
|
|
u8 register_card_provider(const char *url, const char *key)
|
|
{
|
|
if (strlen(url) > 0 && strlen(key) > 0 && strstr(url, "{KEY}") != NULL && strstr(url, "{ID6}") != NULL)
|
|
{
|
|
providers = (struct provider *) realloc(providers, (amount_of_providers + 1) * sizeof(struct provider));
|
|
memset(&providers[amount_of_providers], 0, sizeof(struct provider));
|
|
strncpy((char *) providers[amount_of_providers].url, url, 128);
|
|
strncpy((char *) providers[amount_of_providers].key, key, 48);
|
|
amount_of_providers++;
|
|
gprintf("Gamercard provider is valid!\n");
|
|
return 0;
|
|
}
|
|
gprintf("Gamertag provider is NOT valid!\n");
|
|
return -1;
|
|
}
|
|
|
|
u8 has_enabled_providers()
|
|
{
|
|
if (amount_of_providers != 0 && providers != NULL)
|
|
return 1;
|
|
return 0;
|
|
}
|
|
|
|
void add_game_to_card(const char *gameid)
|
|
{
|
|
int i;
|
|
|
|
char *url = (char *)malloc(MAX_URL_SIZE); // Too much memory, but only like 10 bytes
|
|
memset(url, 0, sizeof(url));
|
|
|
|
for (i = 0; i < amount_of_providers && providers != NULL; i++)
|
|
{
|
|
strcpy(url, (char *) providers[i].url);
|
|
str_replace(url, (char *) "{KEY}", (char *) providers[i].key, MAX_URL_SIZE);
|
|
str_replace(url, (char *) "{ID6}", (char *) gameid, MAX_URL_SIZE);
|
|
|
|
gprintf("Gamertag URL:\n%s\n",(char*)url);
|
|
downloadfile(NULL, 0, (char *) url, NULL, NULL);
|
|
}
|
|
free(url);
|
|
} |