mirror of
https://github.com/Fledge68/WiiFlow_Lite.git
synced 2024-11-01 17:15:06 +01:00
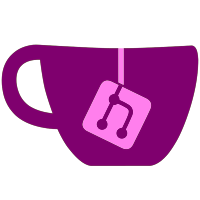
Note: The All Plugins source option has been removed and choosing either nand button on mutiple selection loads the nand you last used (real or emu) The categories_v4.ini had to also be slightly modified to work with this. It will now contain a single 'GENERAL' section which contains the number of categories and the name of each. A suggestion, I would use the first 20 for bostonbc's genres and ratings and the next ten or so for movie types(mplayerce) and the next ten or so for misc. When using more than one source the partition selection and emu nand settings in config are disabled for now. The source button on the main screen still works the same including the d-pad option. Most of the credit for this goes to Fix94 for setting wiiflow up for this a long time ago. I just took the 80% of work he'd already did and finished by getting it to work with the source menu. Note there may be some issues.
86 lines
2.4 KiB
C++
86 lines
2.4 KiB
C++
/****************************************************************************
|
|
* Copyright (C) 2012 FIX94
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
****************************************************************************/
|
|
#ifndef _PLUGIN_HPP_
|
|
#define _PLUGIN_HPP_
|
|
|
|
#include <fstream>
|
|
#include <iostream>
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
#include "config/config.hpp"
|
|
#include "loader/disc.h"
|
|
|
|
using namespace std;
|
|
|
|
#define TAG_GAME_ID "{gameid}"
|
|
#define TAG_LOC "{loc}"
|
|
#define TAG_CONSOLE "{console}"
|
|
|
|
#define PLUGIN_INI_DEF "PLUGIN"
|
|
#define PLUGIN_DEV "{device}"
|
|
#define PLUGIN_PATH "{path}"
|
|
#define PLUGIN_NAME "{name}"
|
|
#define PLUGIN_LDR "{loader}"
|
|
|
|
struct PluginOptions
|
|
{
|
|
u32 magicWord;
|
|
u32 caseColor;
|
|
string DolName;
|
|
string coverFolder;
|
|
string consoleCoverID;
|
|
string BannerSound;
|
|
u32 BannerSoundSize;
|
|
vector<string> Args;
|
|
wstringEx DisplayName;
|
|
};
|
|
|
|
class Plugin
|
|
{
|
|
public:
|
|
bool AddPlugin(Config &plugin);
|
|
u8 *GetBannerSound(u32 magic);
|
|
u32 GetBannerSoundSize();
|
|
const char *GetDolName(u32 magic);
|
|
const char *GetCoverFolderName(u32 magic);
|
|
string GenerateCoverLink(dir_discHdr gameHeader, const string& constURL, Config &Checksums);
|
|
wstringEx GetPluginName(u8 pos);
|
|
u32 getPluginMagic(u8 pos);
|
|
bool PluginExist(u8 pos);
|
|
void SetEnablePlugin(Config &cfg, u8 pos, u8 ForceMode = 0);
|
|
const vector<bool> &GetEnabledPlugins(Config &cfg);
|
|
vector<string> CreateArgs(const char *device, const char *path,
|
|
const char *title, const char *loader, u32 magic);
|
|
void init(const string& m_pluginsDir);
|
|
void Cleanup();
|
|
void EndAdd();
|
|
vector<dir_discHdr> ParseScummvmINI(Config &ini, const char *Device, u32 MagicWord);
|
|
s8 GetPluginPosition(u32 magic);
|
|
private:
|
|
vector<PluginOptions> Plugins;
|
|
vector<bool> enabledPlugins;
|
|
char PluginMagicWord[9];
|
|
s8 Plugin_Pos;
|
|
string pluginsDir;
|
|
bool adding;
|
|
};
|
|
|
|
extern Plugin m_plugin;
|
|
|
|
#endif
|