mirror of
https://github.com/Fledge68/WiiFlow_Lite.git
synced 2024-11-01 09:05:06 +01:00
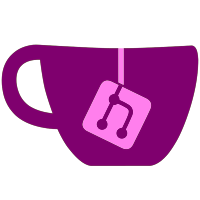
arcade rom clones are not included in the plugins database files to keep the files short. Any arcade rom clones you have will either not be found or will aquire the same ID and title as the parent rom when the CRC of the clone rom is included in the parent roms list of CRC's. you will need to manually edit both the CRC list file and xml database file to include the rom info on any clone you have in order for them to display the correct title and game info. i would think only a small handfull of your roms would be clones. reload cache may take longer now to create the cached list. I found zipped roms for snes, nes, and others are no problem because even if the filename can't be found the crc does not need to be calculated. it is merely taken from the zip file which already holds the crc. on the other hand, half of my gba roms are unzipped with a gba extension. so my gba list takes 10 seconds or so to make because the crc of the unzipped roms does need to be calculated. mame roms use the filename but if it's a clone then it will calculate the crc of the rom zip file. thus any clones will slow things down unless you add them to the database files mentioned above. I imagine megaCD and PS1 cd games will be slow to reload cache. but at least you only need to do it once or whenever you add or delete a game. wiimpathy's database files use the no intro naming convention. i'm not crazy about it because it moves any leading 'the' to the end preceded by a comma. in this case i use custom titles ini to put the title the way i want.
59 lines
2.1 KiB
C++
59 lines
2.1 KiB
C++
/****************************************************************************
|
|
* Copyright (C) 2012 FIX94
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
****************************************************************************/
|
|
#ifndef _LISTGENERATOR_HPP_
|
|
#define _LISTGENERATOR_HPP_
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
#include <stdio.h>
|
|
|
|
#include "defines.h"
|
|
#include "types.h"
|
|
#include "config/config.hpp"
|
|
#include "loader/wbfs.h"
|
|
#include "loader/disc.h"
|
|
#include "gui/GameTDB.hpp"
|
|
#include "plugin/plugin.hpp"
|
|
|
|
using namespace std;
|
|
|
|
class ListGenerator : public vector<dir_discHdr>
|
|
{
|
|
public:
|
|
void createSFList(u8 maxBtns, Config &m_sourceMenuCfg, const string& sourceDir);
|
|
void Init(const char *settingsDir, const char *Language, const char *plgnsDataDir);
|
|
void Clear();
|
|
void CreateRomList(Config &platform_cfg, const string& romsDir, const vector<string>& FileTypes, const string& DBName, bool UpdateCache);
|
|
void CreateList(u32 Flow, u32 Device, const string& Path, const vector<string>& FileTypes,
|
|
const string& DBName, bool UpdateCache);
|
|
u32 Color;
|
|
u32 Magic;
|
|
private:
|
|
void OpenConfigs();
|
|
void CloseConfigs();
|
|
string gameTDB_Path;
|
|
string CustomTitlesPath;
|
|
string gameTDB_Language;
|
|
};
|
|
|
|
typedef void (*FileAdder)(char *Path);
|
|
void GetFiles(const char *Path, const vector<string>& FileTypes,
|
|
FileAdder AddFile, bool CompareFolders, u32 max_depth = 2, u32 depth = 1);
|
|
extern ListGenerator m_cacheList;
|
|
|
|
#endif /*_LISTGENERATOR_HPP_*/
|