mirror of
https://github.com/wiiu-env/WiiUPluginLoaderBackend.git
synced 2024-11-06 04:55:07 +01:00
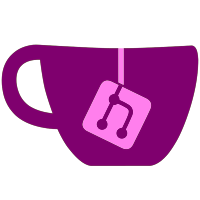
- avoid using const std::unique_ptr& and const std::shared_ptr& - avoid wrapping results in std::optional - prefer std::string_view over const std::string& - update FSUtils::LoadFileToMem to write into std::vector<uint8_t> - use std::span when possible - Avoid unnessecary copies in PluginDataFactory - allocate plugins as HeapMemoryFixedSize which bascially is a std::unique_ptr with fixed size
45 lines
1.2 KiB
C++
45 lines
1.2 KiB
C++
#pragma once
|
|
#include "utils.h"
|
|
#include <cstdint>
|
|
#include <memory>
|
|
|
|
class HeapMemoryFixedSize {
|
|
public:
|
|
HeapMemoryFixedSize() = default;
|
|
|
|
explicit HeapMemoryFixedSize(std::size_t size) : mData(make_unique_nothrow<uint8_t[]>(size)), mSize(mData ? size : 0) {}
|
|
|
|
// Delete the copy constructor and copy assignment operator
|
|
HeapMemoryFixedSize(const HeapMemoryFixedSize &) = delete;
|
|
HeapMemoryFixedSize &operator=(const HeapMemoryFixedSize &) = delete;
|
|
|
|
HeapMemoryFixedSize(HeapMemoryFixedSize &&other) noexcept
|
|
: mData(std::move(other.mData)), mSize(other.mSize) {
|
|
other.mSize = 0;
|
|
}
|
|
|
|
HeapMemoryFixedSize &operator=(HeapMemoryFixedSize &&other) noexcept {
|
|
if (this != &other) {
|
|
mData = std::move(other.mData);
|
|
mSize = other.mSize;
|
|
other.mSize = 0;
|
|
}
|
|
return *this;
|
|
}
|
|
|
|
explicit operator bool() const {
|
|
return mData != nullptr;
|
|
}
|
|
|
|
[[nodiscard]] const void *data() const {
|
|
return mData.get();
|
|
}
|
|
|
|
[[nodiscard]] std::size_t size() const {
|
|
return mSize;
|
|
}
|
|
|
|
private:
|
|
std::unique_ptr<uint8_t[]> mData{};
|
|
std::size_t mSize{};
|
|
}; |