mirror of
https://github.com/wiiu-env/WiiUPluginLoaderBackend.git
synced 2024-11-06 13:05:07 +01:00
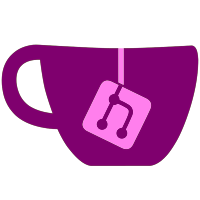
- avoid using const std::unique_ptr& and const std::shared_ptr& - avoid wrapping results in std::optional - prefer std::string_view over const std::string& - update FSUtils::LoadFileToMem to write into std::vector<uint8_t> - use std::span when possible - Avoid unnessecary copies in PluginDataFactory - allocate plugins as HeapMemoryFixedSize which bascially is a std::unique_ptr with fixed size
62 lines
1.3 KiB
C++
62 lines
1.3 KiB
C++
#include "fs/FSUtils.h"
|
|
#include "utils/logger.h"
|
|
#include "utils/utils.h"
|
|
#include <cstdio>
|
|
#include <cstring>
|
|
#include <fcntl.h>
|
|
#include <filesystem>
|
|
#include <unistd.h>
|
|
#include <vector>
|
|
|
|
int32_t FSUtils::LoadFileToMem(std::string_view filepath, std::vector<uint8_t> &buffer) {
|
|
//! always initialize input
|
|
buffer.clear();
|
|
|
|
int32_t iFd = open(filepath.data(), O_RDONLY);
|
|
if (iFd < 0) {
|
|
return -1;
|
|
}
|
|
|
|
struct stat file_stat {};
|
|
int rc = fstat(iFd, &file_stat);
|
|
if (rc < 0) {
|
|
close(iFd);
|
|
return -4;
|
|
}
|
|
uint32_t filesize = file_stat.st_size;
|
|
|
|
buffer.resize(filesize);
|
|
|
|
uint32_t blocksize = 0x80000;
|
|
uint32_t done = 0;
|
|
int32_t readBytes;
|
|
|
|
while (done < filesize) {
|
|
if (done + blocksize > filesize) {
|
|
blocksize = filesize - done;
|
|
}
|
|
readBytes = read(iFd, buffer.data() + done, blocksize);
|
|
if (readBytes <= 0) {
|
|
break;
|
|
}
|
|
done += readBytes;
|
|
}
|
|
|
|
::close(iFd);
|
|
|
|
if (done != filesize) {
|
|
buffer.clear();
|
|
return -3;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
bool FSUtils::CreateSubfolder(std::string_view fullpath) {
|
|
std::error_code err;
|
|
if (!std::filesystem::create_directories(fullpath, err)) {
|
|
return std::filesystem::exists(fullpath, err);
|
|
}
|
|
return true;
|
|
}
|