mirror of
https://github.com/cemu-project/DS4Windows.git
synced 2024-11-23 01:39:17 +01:00
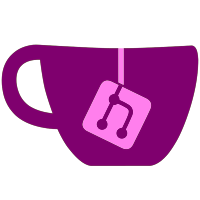
Improved mapping support: can better handle the switching of controls: ie triangle and circle/dpad and left stick/L1+R1 and L2+R2. If you have a profile with swap buttons, they may not work until you open the profile in settings and resave. Servers moved to ds4winsdows.com Remove DS4Windows from Alt+tab menu if minimized to tray (thanks youturnjason) Basic support for command line arguments (right now just "-stop" is supported: starts program up without starting the ds4)
65 lines
2.2 KiB
C#
65 lines
2.2 KiB
C#
using System;
|
|
using System.Windows.Forms;
|
|
using System.Threading;
|
|
using System.Runtime.InteropServices;
|
|
using System.Diagnostics;
|
|
|
|
|
|
namespace ScpServer
|
|
{
|
|
static class Program
|
|
{
|
|
[DllImport("user32.dll")]
|
|
[return: MarshalAs(UnmanagedType.Bool)]
|
|
static extern bool SetForegroundWindow(IntPtr hWnd);
|
|
|
|
[DllImport("user32.dll")]
|
|
private static extern IntPtr GetForegroundWindow();
|
|
|
|
/// <summary>
|
|
/// The main entry point for the application.
|
|
/// </summary>
|
|
[STAThread]
|
|
static void Main(string [] args)
|
|
{
|
|
System.Runtime.GCSettings.LatencyMode = System.Runtime.GCLatencyMode.LowLatency;
|
|
try
|
|
{
|
|
System.Diagnostics.Process.GetCurrentProcess().PriorityClass = System.Diagnostics.ProcessPriorityClass.High;
|
|
}
|
|
catch
|
|
{
|
|
// Ignore problems raising the priority.
|
|
}
|
|
bool createdNew = true;
|
|
using (Mutex mutex = new Mutex(true, "MyApplicationName", out createdNew))
|
|
{
|
|
if (createdNew)
|
|
{
|
|
Application.EnableVisualStyles();
|
|
Application.SetCompatibleTextRenderingDefault(false);
|
|
Application.Run(new ScpForm(args));
|
|
}
|
|
else
|
|
{
|
|
Process current = Process.GetCurrentProcess();
|
|
foreach (Process process in Process.GetProcessesByName("DS4Windows"))
|
|
{
|
|
if (process.Id != current.Id)
|
|
{
|
|
SetForegroundWindow(process.MainWindowHandle);
|
|
if (GetForegroundWindow() != process.MainWindowHandle) //if tool is minimized to tray
|
|
{
|
|
Application.EnableVisualStyles();
|
|
Application.SetCompatibleTextRenderingDefault(false);
|
|
Application.Run(new Alreadyrunning());
|
|
}
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|