mirror of
https://github.com/cemu-project/idapython.git
synced 2024-11-24 18:16:55 +01:00
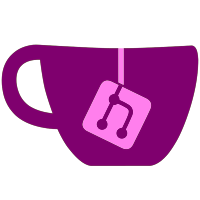
What's new: - Proper multi-threaded support - Better PyObject reference counting with ref_t and newref_t helper classes - Improved the pywraps/deployment script - Added IDAViewWrapper class and example - Added idc.GetDisasmEx() - Added idc.AddSegEx() - Added idc.GetLocalTinfo() - Added idc.ApplyType() - Updated type information implementation - Introduced the idaapi.require() - see http://www.hexblog.com/?p=749 - set REMOVE_CWD_SYS_PATH=1 by default in python.cfg (remove current directory from the import search path). Various bugfixes: - fixed various memory leaks - asklong/askaddr/asksel (and corresponding idc.py functions) were returning results truncated to 32 bits in IDA64 - fix wrong documentation for idc.SizeOf - GetFloat/GetDouble functions did not take into account endianness of the processor - idaapi.NO_PROCESS was not defined, and was causing GetProcessPid() to fail - idc.py: insert escape characters to string parameter when call Eval() - idc.SaveFile/savefile were always overwriting an existing file instead of writing only the new data - PluginForm.Close() wasn't passing its arguments to the delegate function, resulting in an error.
91 lines
1.8 KiB
Python
91 lines
1.8 KiB
Python
import sys
|
|
import os
|
|
|
|
def __sys(cmd, fmt=None, echo=True):
|
|
"""Executes a string of OS commands and returns the a list of tuples (return code,command executed)"""
|
|
if not fmt:
|
|
fmt = {}
|
|
r = []
|
|
for cmd in [x for x in (cmd % fmt).split("\n") if len(x)]:
|
|
if echo:
|
|
print ">>>", cmd
|
|
r.append((os.system(cmd), cmd))
|
|
return r
|
|
|
|
body = r"""/// Autogenerated file
|
|
#include <stdio.h>
|
|
#include <conio.h>
|
|
#include <ctype.h>
|
|
#include <windows.h>
|
|
|
|
void want_break(int n)
|
|
{
|
|
printf("do you want to DebugBreak in func%d()?", n);
|
|
char ch = _toupper(_getch());
|
|
printf("\n");
|
|
if (ch == 'Y')
|
|
DebugBreak();
|
|
else if (ch == 'X')
|
|
ExitProcess(n);
|
|
}
|
|
%FUNCS%
|
|
int main(int /*argc*/, char * /*argv[]*/)
|
|
{
|
|
func1();
|
|
return 0;
|
|
}
|
|
"""
|
|
|
|
funcs_body = []
|
|
|
|
func_body = r"""
|
|
void func%(n)d()
|
|
{
|
|
printf("%(ident)senter %(n)d\n");%(pause)s
|
|
func%(n1)d();
|
|
printf("%(ident)sleave %(n)d\n");
|
|
}
|
|
"""
|
|
|
|
if len(sys.argv) < 2:
|
|
print "usage: gen nb_calls pause_frequency"
|
|
sys.exit(0)
|
|
|
|
n = int(sys.argv[1])
|
|
if n < 1:
|
|
print "at least one call should be passed!"
|
|
sys.exit(1)
|
|
|
|
m = int(sys.argv[2])
|
|
|
|
func_params = {'n': 0, 'n1': 0, 'ident': '', 'pause' : ''}
|
|
|
|
for i in xrange(1, n + 1):
|
|
func_params['n'] = i
|
|
func_params['n1'] = i+1
|
|
func_params['ident'] = " " * i
|
|
func_params['pause'] = ("\n want_break(%d);" % i) if (i % m) == 0 else ''
|
|
|
|
funcs_body.append(func_body % func_params)
|
|
funcs_body.append(r"""
|
|
void func%(n)d()
|
|
{
|
|
printf("that's it #%(n)d!\n");
|
|
}
|
|
""" % {'n':i+1})
|
|
funcs_body.reverse()
|
|
|
|
# write the file
|
|
body = body.replace('%FUNCS%', ''.join(funcs_body))
|
|
f = file('src.cpp', 'w')
|
|
f.write(body)
|
|
f.close()
|
|
|
|
|
|
__sys("""
|
|
if exist src.exe del src.exe
|
|
bcc32 src
|
|
if exist src.exe move src.exe src_bcc.exe
|
|
if exist src.obj del src.obj
|
|
cl32 src.cpp /Zi /Od
|
|
""") |