mirror of
https://github.com/cemu-project/idapython.git
synced 2024-11-24 18:16:55 +01:00
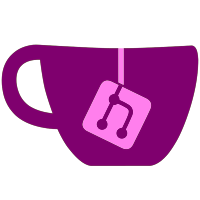
What's new: - Proper multi-threaded support - Better PyObject reference counting with ref_t and newref_t helper classes - Improved the pywraps/deployment script - Added IDAViewWrapper class and example - Added idc.GetDisasmEx() - Added idc.AddSegEx() - Added idc.GetLocalTinfo() - Added idc.ApplyType() - Updated type information implementation - Introduced the idaapi.require() - see http://www.hexblog.com/?p=749 - set REMOVE_CWD_SYS_PATH=1 by default in python.cfg (remove current directory from the import search path). Various bugfixes: - fixed various memory leaks - asklong/askaddr/asksel (and corresponding idc.py functions) were returning results truncated to 32 bits in IDA64 - fix wrong documentation for idc.SizeOf - GetFloat/GetDouble functions did not take into account endianness of the processor - idaapi.NO_PROCESS was not defined, and was causing GetProcessPid() to fail - idc.py: insert escape characters to string parameter when call Eval() - idc.SaveFile/savefile were always overwriting an existing file instead of writing only the new data - PluginForm.Close() wasn't passing its arguments to the delegate function, resulting in an error.
99 lines
2.9 KiB
Python
99 lines
2.9 KiB
Python
"""
|
|
Deploy code snips into swig interface files
|
|
|
|
(c) Hex-Rays
|
|
"""
|
|
|
|
import sys
|
|
import re
|
|
import os
|
|
|
|
# creates a regular expression
|
|
def make_re(tag, mod_name, prefix):
|
|
s = '%(p)s<%(tag)s\(%(m)s\)>(.+?)%(p)s</%(tag)s\(%(m)s\)>' % {'m': mod_name, 'tag': tag, 'p': prefix}
|
|
return (s, re.compile(s, re.DOTALL))
|
|
|
|
def convert_path(path_in):
|
|
parts = path_in.split('/')
|
|
return os.sep.join(parts)
|
|
|
|
def deploy(mod_name, src_files, dest_file, silent = True):
|
|
dest_file = convert_path(dest_file)
|
|
src_files = map(convert_path, src_files)
|
|
# create regular expressions
|
|
templates = (
|
|
('pycode', make_re('pycode', mod_name, '#')),
|
|
('code', make_re('code', mod_name, '//')),
|
|
('inline', make_re('inline', mod_name, '//'))
|
|
)
|
|
|
|
if not os.path.exists(dest_file):
|
|
print "File", dest_file, "does not exist and will be skipped"
|
|
return
|
|
|
|
if not os.access(dest_file, os.W_OK):
|
|
print "File", dest_file, "is not writable and will be skipped"
|
|
return
|
|
|
|
# read dest file
|
|
dest_lines = "".join(file(dest_file, "r").readlines())
|
|
|
|
# read all source files into one buffer
|
|
src_lines = "".join(["".join(file(x, "r").readlines()) for x in src_files])
|
|
|
|
pcount = 0
|
|
for desc, (expr_str, expr) in templates:
|
|
# find source pattern
|
|
matches = expr.findall(src_lines)
|
|
if not matches:
|
|
if not silent:
|
|
print "Failed to match <%s> source expression against '%s', skipping...!" % (desc, expr_str)
|
|
continue
|
|
|
|
# find pattern in destination
|
|
dest = expr.search(dest_lines)
|
|
if not dest:
|
|
if not silent:
|
|
print "Failed to match <%s> destination expression against '%s', skipping..." % (desc, expr_str)
|
|
print dest_lines
|
|
sys.exit(0)
|
|
continue
|
|
|
|
# accumulate all the strings to be replaced
|
|
replaces = []
|
|
for src in matches:
|
|
replaces.append(src)
|
|
|
|
dest_lines = dest_lines[:dest.start(1)] + "\n".join(replaces) + dest_lines[dest.end(1):]
|
|
pcount += 1
|
|
|
|
|
|
f = file(dest_file, 'w')
|
|
if not f:
|
|
print "Failed to open destination file:", dest_file
|
|
return
|
|
f.write(dest_lines)
|
|
f.close()
|
|
|
|
if pcount:
|
|
print "Deployed successfully: %s (%d)" % (dest_file, pcount)
|
|
else:
|
|
print "Nothing was deployed in: %s" % dest_file
|
|
|
|
|
|
def main(argv = None):
|
|
if not argv:
|
|
argv = sys.argv
|
|
if len(argv) != 4:
|
|
print "Usage deploy.py modname src_file1,src_file2,... dest_file"
|
|
return
|
|
|
|
mod_name = argv[1]
|
|
src_files = argv[2].split(',')
|
|
dest_file = argv[3]
|
|
deploy(mod_name, src_files, dest_file)
|
|
|
|
#main(['', 'py_graph', 'py_graph.hpp,py_graph.py', 'graph.i'])
|
|
if __name__ == '__main__':
|
|
main()
|