mirror of
https://github.com/cemu-project/idapython.git
synced 2024-11-24 18:16:55 +01:00
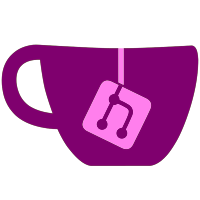
- IDA Pro 6.2 support - added set_idc_func_ex(): it is now possible to add new IDC functions using Python - added visit_patched_bytes() (see ex_patch.py) - added support for the multiline text input control in the Form class - added support for the editable/readonly dropdown list control in the Form class - added execute_sync() to register a function call into the UI message queue - added execute_ui_requests() / check ex_uirequests.py - added add_hotkey() / del_hotkey() to bind Python methods to hotkeys - added register_timer()/unregister_timer(). Check ex_timer.py - added the IDC (Arrays) netnode manipulation layer into idc.py - added idautils.Structs() and StructMembers() generator functions - removed the "Run Python Statement" menu item. IDA now has a unified dialog. Use RunPlugin("python", 0) to invoke it manually. - better error messages for script plugins, loaders and processor modules - bugfix: Dbg_Hooks.dbg_run_to() was receiving wrong input - bugfix: A few Enum related functions were not properly working in idc.py - bugfix: GetIdaDirectory() and GetProcessName() were broken in idc.py - bugfix: idaapi.get_item_head() / idc.ItemHead() were not working
38 lines
1.2 KiB
Python
38 lines
1.2 KiB
Python
# -------------------------------------------------------------------------
|
|
# This is an example illustrating how to use timers
|
|
# (c) Hex-Rays
|
|
|
|
import idaapi
|
|
|
|
# -------------------------------------------------------------------------
|
|
class timercallback_t(object):
|
|
def __init__(self):
|
|
self.interval = 1000
|
|
self.obj = idaapi.register_timer(self.interval, self)
|
|
if self.obj is None:
|
|
raise RuntimeError, "Failed to register timer"
|
|
self.times = 5
|
|
|
|
def __call__(self):
|
|
print("Timer invoked. %d time(s) left" % self.times)
|
|
self.times -= 1
|
|
# Unregister the timer when the counter reaches zero
|
|
return -1 if self.times == 0 else self.interval
|
|
|
|
def __del__(self):
|
|
print("Timer object disposed %s" % id(self))
|
|
|
|
|
|
# -------------------------------------------------------------------------
|
|
def main():
|
|
try:
|
|
t = timercallback_t()
|
|
# No need to unregister the timer.
|
|
# It will unregister itself in the callback when it returns -1
|
|
except Exception as e:
|
|
print "Error: %s" % e
|
|
|
|
|
|
# -------------------------------------------------------------------------
|
|
if __name__ == '__main__':
|
|
main() |