mirror of
https://github.com/cemu-project/vcpkg.git
synced 2025-02-24 19:43:33 +01:00
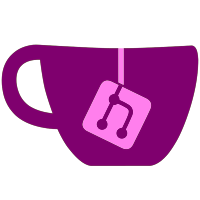
* [vcpkg] Correct UInt128 code 😇
`UInt128::operator<<(x, y)` should clear the bottom 64 bits of `x` if
`y >= 64`; however, we don't do this, and so we duplicate `x`'s bottom
bits into `x.top` instead of moving them. Similarly, we have the
opposite problem for `UInt128::operator>>`. This commit fixes these
latent bugs, which we weren't hitting because the thing we use them for
never actually shifts more than 64 bits.
27 lines
487 B
C++
27 lines
487 B
C++
#pragma once
|
|
|
|
#include <stdint.h>
|
|
|
|
namespace vcpkg {
|
|
|
|
struct UInt128 {
|
|
UInt128() = default;
|
|
UInt128(uint64_t value) : bottom(value), top(0) {}
|
|
|
|
UInt128& operator<<=(int by) noexcept;
|
|
UInt128& operator>>=(int by) noexcept;
|
|
UInt128& operator+=(uint64_t lhs) noexcept;
|
|
|
|
uint64_t bottom_64_bits() const noexcept {
|
|
return bottom;
|
|
}
|
|
uint64_t top_64_bits() const noexcept {
|
|
return top;
|
|
}
|
|
private:
|
|
uint64_t bottom;
|
|
uint64_t top;
|
|
};
|
|
|
|
}
|