mirror of
https://github.com/cemu-project/vcpkg.git
synced 2025-02-24 11:37:12 +01:00
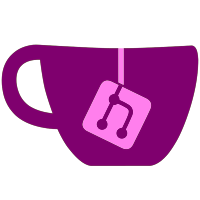
* [vcpkg] Add initial JSON support This adds a JSON parser, as well as the amount of unicode support required for JSON parsing to work according to the specification. In the future, I hope to rewrite our existing XML files into JSON. Additionally, as a drive-by, we've added the following: * add /wd4800 to pragmas.h -- this is a "performance warning", for when you implicitly convert pointers or integers to bool, and shouldn't be an issue for us. * Switched Parse::ParserBase to read unicode (as utf-8), as opposed to ASCII * Building again under VCPKG_DEVELOPMENT_WARNINGS, yay!
52 lines
2.1 KiB
C++
52 lines
2.1 KiB
C++
#pragma once
|
|
|
|
#include <vcpkg/base/expected.h>
|
|
#include <vcpkg/packagespec.h>
|
|
#include <vcpkg/textrowcol.h>
|
|
|
|
#include <memory>
|
|
#include <string>
|
|
#include <unordered_map>
|
|
#include <vector>
|
|
|
|
namespace vcpkg::Parse
|
|
{
|
|
struct ParseControlErrorInfo
|
|
{
|
|
std::string name;
|
|
std::vector<std::string> missing_fields;
|
|
std::vector<std::string> extra_fields;
|
|
std::string error;
|
|
};
|
|
|
|
template<class P>
|
|
using ParseExpected = vcpkg::ExpectedT<std::unique_ptr<P>, std::unique_ptr<ParseControlErrorInfo>>;
|
|
|
|
using Paragraph = std::unordered_map<std::string, std::pair<std::string, TextRowCol>>;
|
|
|
|
struct ParagraphParser
|
|
{
|
|
ParagraphParser(Paragraph&& fields) : fields(std::move(fields)) {}
|
|
|
|
void required_field(const std::string& fieldname, std::string& out);
|
|
std::string optional_field(const std::string& fieldname);
|
|
void required_field(const std::string& fieldname, std::pair<std::string&, TextRowCol&> out);
|
|
void optional_field(const std::string& fieldname, std::pair<std::string&, TextRowCol&> out);
|
|
std::unique_ptr<ParseControlErrorInfo> error_info(const std::string& name) const;
|
|
|
|
private:
|
|
Paragraph&& fields;
|
|
std::vector<std::string> missing_fields;
|
|
};
|
|
|
|
ExpectedS<std::vector<std::string>> parse_default_features_list(const std::string& str,
|
|
StringView origin = "<unknown>",
|
|
TextRowCol textrowcol = {});
|
|
ExpectedS<std::vector<ParsedQualifiedSpecifier>> parse_qualified_specifier_list(const std::string& str,
|
|
StringView origin = "<unknown>",
|
|
TextRowCol textrowcol = {});
|
|
ExpectedS<std::vector<Dependency>> parse_dependencies_list(const std::string& str,
|
|
StringView origin = "<unknown>",
|
|
TextRowCol textrowcol = {});
|
|
}
|