mirror of
https://github.com/cemu-project/vcpkg.git
synced 2025-02-24 11:37:12 +01:00
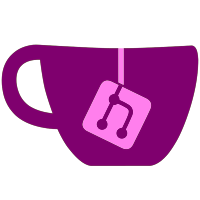
* Ports Overlay feature spec * Ports Overlay implementation * [--overlay-ports] Refactor handling of additional paths * Code cleanup * [--overlay-ports] Add help * [depend-info] Support --overlay-ports * Add method to load all ports using PathsPortFileProvider * Make PortFileProvider::load_all_control_files() const * Remove unused code * [vcpkg] Avoid double-load of source control file between Build::perform_and_exit and Build::perform_and_exit_ex * [vcpkg] Clang format * [vcpkg] Fixup build failure introduced in b069ceb2f231 * Report errors from Paragraphs::try_load_port()
108 lines
2.9 KiB
C++
108 lines
2.9 KiB
C++
#pragma once
|
|
|
|
#include <vcpkg/base/optional.h>
|
|
#include <vcpkg/base/span.h>
|
|
#include <vcpkg/base/stringliteral.h>
|
|
|
|
#include <memory>
|
|
#include <unordered_map>
|
|
#include <unordered_set>
|
|
#include <vector>
|
|
|
|
namespace vcpkg
|
|
{
|
|
struct ParsedArguments
|
|
{
|
|
std::unordered_set<std::string> switches;
|
|
std::unordered_map<std::string, std::string> settings;
|
|
std::unordered_map<std::string, std::vector<std::string>> multisettings;
|
|
};
|
|
|
|
struct VcpkgPaths;
|
|
|
|
struct CommandSwitch
|
|
{
|
|
constexpr CommandSwitch(const StringLiteral& name, const StringLiteral& short_help_text)
|
|
: name(name), short_help_text(short_help_text)
|
|
{
|
|
}
|
|
|
|
StringLiteral name;
|
|
StringLiteral short_help_text;
|
|
};
|
|
|
|
struct CommandSetting
|
|
{
|
|
constexpr CommandSetting(const StringLiteral& name, const StringLiteral& short_help_text)
|
|
: name(name), short_help_text(short_help_text)
|
|
{
|
|
}
|
|
|
|
StringLiteral name;
|
|
StringLiteral short_help_text;
|
|
};
|
|
|
|
struct CommandMultiSetting
|
|
{
|
|
constexpr CommandMultiSetting(const StringLiteral& name, const StringLiteral& short_help_text)
|
|
: name(name), short_help_text(short_help_text)
|
|
{
|
|
}
|
|
|
|
StringLiteral name;
|
|
StringLiteral short_help_text;
|
|
};
|
|
|
|
struct CommandOptionsStructure
|
|
{
|
|
Span<const CommandSwitch> switches;
|
|
Span<const CommandSetting> settings;
|
|
Span<const CommandMultiSetting> multisettings;
|
|
};
|
|
|
|
struct CommandStructure
|
|
{
|
|
std::string example_text;
|
|
|
|
size_t minimum_arity;
|
|
size_t maximum_arity;
|
|
|
|
CommandOptionsStructure options;
|
|
|
|
std::vector<std::string> (*valid_arguments)(const VcpkgPaths& paths);
|
|
};
|
|
|
|
void display_usage(const CommandStructure& command_structure);
|
|
|
|
#if defined(_WIN32)
|
|
using CommandLineCharType = wchar_t;
|
|
#else
|
|
using CommandLineCharType = char;
|
|
#endif
|
|
|
|
struct VcpkgCmdArguments
|
|
{
|
|
static VcpkgCmdArguments create_from_command_line(const int argc, const CommandLineCharType* const* const argv);
|
|
static VcpkgCmdArguments create_from_arg_sequence(const std::string* arg_begin, const std::string* arg_end);
|
|
|
|
std::unique_ptr<std::string> vcpkg_root_dir;
|
|
std::unique_ptr<std::string> triplet;
|
|
std::unique_ptr<std::vector<std::string>> overlay_ports;
|
|
Optional<bool> debug = nullopt;
|
|
Optional<bool> sendmetrics = nullopt;
|
|
Optional<bool> printmetrics = nullopt;
|
|
|
|
// feature flags
|
|
Optional<bool> featurepackages = nullopt;
|
|
Optional<bool> binarycaching = nullopt;
|
|
|
|
std::string command;
|
|
std::vector<std::string> command_arguments;
|
|
|
|
ParsedArguments parse_arguments(const CommandStructure& command_structure) const;
|
|
|
|
private:
|
|
std::unordered_map<std::string, Optional<std::vector<std::string>>> optional_command_arguments;
|
|
};
|
|
}
|