mirror of
https://github.com/cemu-project/vcpkg.git
synced 2025-02-25 03:53:32 +01:00
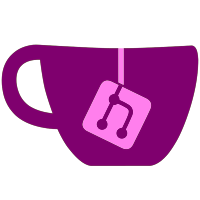
This changes our PR builds to treat 'fail' in the ci.baseline.txt as 'skip' instead of using tombstones. We currently have large numbers of spurious failures that get enshrined in PRs through no fault of a PR author, removing the tombstones concept will fix those by allowing the user to retry. This does mean we accept some risk of not detecting when a port is 'fixed', but that failure is reasonable for us to handle after we see it in CI, but that seems worth it given that it lets us get rid of the tombstone concept. This also helps out the binary caching feature, because we don't have to figure out how to productize tombstones.
134 lines
4.0 KiB
C++
134 lines
4.0 KiB
C++
#pragma once
|
|
|
|
#include <vcpkg/binaryparagraph.h>
|
|
#include <vcpkg/packagespec.h>
|
|
#include <vcpkg/tools.h>
|
|
#include <vcpkg/vcpkgcmdarguments.h>
|
|
|
|
#include <vcpkg/base/cache.h>
|
|
#include <vcpkg/base/files.h>
|
|
#include <vcpkg/base/lazy.h>
|
|
#include <vcpkg/base/optional.h>
|
|
#include <vcpkg/base/util.h>
|
|
|
|
namespace vcpkg
|
|
{
|
|
namespace Tools
|
|
{
|
|
static const std::string SEVEN_ZIP = "7zip";
|
|
static const std::string SEVEN_ZIP_ALT = "7z";
|
|
static const std::string MAVEN = "mvn";
|
|
static const std::string CMAKE = "cmake";
|
|
static const std::string GIT = "git";
|
|
static const std::string MONO = "mono";
|
|
static const std::string NINJA = "ninja";
|
|
static const std::string NUGET = "nuget";
|
|
static const std::string IFW_INSTALLER_BASE = "ifw_installerbase";
|
|
static const std::string IFW_BINARYCREATOR = "ifw_binarycreator";
|
|
static const std::string IFW_REPOGEN = "ifw_repogen";
|
|
}
|
|
|
|
struct ToolsetArchOption
|
|
{
|
|
CStringView name;
|
|
System::CPUArchitecture host_arch;
|
|
System::CPUArchitecture target_arch;
|
|
};
|
|
|
|
struct Toolset
|
|
{
|
|
fs::path visual_studio_root_path;
|
|
fs::path dumpbin;
|
|
fs::path vcvarsall;
|
|
std::vector<std::string> vcvarsall_options;
|
|
CStringView version;
|
|
std::vector<ToolsetArchOption> supported_architectures;
|
|
};
|
|
|
|
namespace Build
|
|
{
|
|
struct PreBuildInfo;
|
|
struct AbiInfo;
|
|
}
|
|
|
|
namespace System
|
|
{
|
|
struct Environment;
|
|
}
|
|
|
|
namespace details
|
|
{
|
|
struct VcpkgPathsImpl;
|
|
}
|
|
|
|
struct VcpkgPaths : Util::MoveOnlyBase
|
|
{
|
|
struct TripletFile
|
|
{
|
|
std::string name;
|
|
fs::path location;
|
|
|
|
TripletFile(const std::string& name, const fs::path& location) : name(name), location(location) { }
|
|
};
|
|
|
|
VcpkgPaths(Files::Filesystem& filesystem, const VcpkgCmdArguments& args);
|
|
~VcpkgPaths();
|
|
|
|
fs::path package_dir(const PackageSpec& spec) const;
|
|
fs::path build_dir(const PackageSpec& spec) const;
|
|
fs::path build_dir(const std::string& package_name) const;
|
|
fs::path build_info_file_path(const PackageSpec& spec) const;
|
|
fs::path listfile_path(const BinaryParagraph& pgh) const;
|
|
|
|
bool is_valid_triplet(Triplet t) const;
|
|
const std::vector<std::string> get_available_triplets_names() const;
|
|
const std::vector<TripletFile>& get_available_triplets() const;
|
|
const fs::path get_triplet_file_path(Triplet triplet) const;
|
|
|
|
fs::path original_cwd;
|
|
fs::path root;
|
|
fs::path manifest_root_dir;
|
|
fs::path buildtrees;
|
|
fs::path downloads;
|
|
fs::path packages;
|
|
fs::path ports;
|
|
fs::path installed;
|
|
fs::path triplets;
|
|
fs::path community_triplets;
|
|
fs::path scripts;
|
|
fs::path prefab;
|
|
|
|
fs::path tools;
|
|
fs::path buildsystems;
|
|
fs::path buildsystems_msbuild_targets;
|
|
fs::path buildsystems_msbuild_props;
|
|
|
|
fs::path vcpkg_dir;
|
|
fs::path vcpkg_dir_status_file;
|
|
fs::path vcpkg_dir_info;
|
|
fs::path vcpkg_dir_updates;
|
|
|
|
fs::path ports_cmake;
|
|
|
|
const fs::path& get_tool_exe(const std::string& tool) const;
|
|
const std::string& get_tool_version(const std::string& tool) const;
|
|
|
|
/// <summary>Retrieve a toolset matching a VS version</summary>
|
|
/// <remarks>
|
|
/// Valid version strings are "v120", "v140", "v141", and "". Empty string gets the latest.
|
|
/// </remarks>
|
|
const Toolset& get_toolset(const Build::PreBuildInfo& prebuildinfo) const;
|
|
|
|
Files::Filesystem& get_filesystem() const;
|
|
|
|
const System::Environment& get_action_env(const Build::AbiInfo& abi_info) const;
|
|
const std::string& get_triplet_info(const Build::AbiInfo& abi_info) const;
|
|
bool manifest_mode_enabled() const { return !manifest_root_dir.empty(); }
|
|
|
|
void track_feature_flag_metrics() const;
|
|
|
|
private:
|
|
std::unique_ptr<details::VcpkgPathsImpl> m_pimpl;
|
|
};
|
|
}
|