mirror of
https://github.com/cemu-project/vcpkg.git
synced 2025-02-24 11:37:12 +01:00
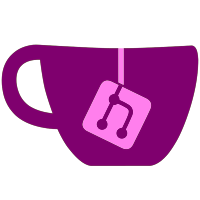
==== Changes Related to manifests ==== * Add the `manifests` feature flag * This only says whether we look for a `vcpkg.json` in the cwd, not whether we support parsing manifests (for ports, for example) * Changes to the manifests RFC * `"authors"` -> `"maintainers"` * `--x-classic-mode` -> `-manifests` \in `vcpkg_feature_flags` * reserve `"core"` in addition to `"default"`, since that's already reserved for features * Add a small helper note about what identifiers must look like * `<license-string>`: SPDX v3.8 -> v3.9 * `"feature"."description"` is allowed to be an array of strings as well * `"version"` -> `"version-string"` for forward-compat with versions RFC * Add the `--feature-flags` option * Add the ability to turn off feature flags via passing `-<feature-flag>` to `VCPKG_FEATURE_FLAGS` or `--feature-flags` * Add CMake toolchain support for manifests * Requires either: * a feature flag of `manifests` in either `Env{VCPKG_FEATURE_FLAGS}` or `VCPKG_FEATURE_FLAGS` * Passing the `VCPKG_ENABLE_MANIFESTS` option * The toolchain will install your packages to `${VCPKG_MANIFEST_DIR}/vcpkg_installed`. * Add MSBuild `vcpkg integrate install` support for manifests * Requires `VcpkgEnableManifest` to be true * `vcpkg create` creates a port that has a `vcpkg.json` instead of a `CONTROL` * argparse, abseil, 3fd, and avisynthplus ports switched to manifest from CONTROL * Add support for `--x-manifest-root`, as well as code for finding it if not passed * Add support for parsing manifests! * Add a filesystem lock! ==== Important Changes which are somewhat unrelated to manifests ==== * Rename `logicexpression.{h,cpp}` to `platform-expression.{h,cpp}` * Add `PlatformExpression` type which takes the place of the old logic expression * Split the parsing of platform expressions from checking whether they're true or not * Eagerly parse PlatformExpressions as opposed to leaving them as strings * Add checking for feature flag consistency * i.e., if `-binarycaching` is passed, you shouldn't be passing `--binarysource` * Add the `Json::Reader` type which, with the help of user-defined visitors, converts JSON to your internal type * VcpkgArgParser: place the switch names into a constant as opposed to using magic constants * In general update the parsing code so that this ^ works * Add `Port-Version` fields to CONTROL files * This replaces the existing practice of `Version: <my-version>-<port-version>` ==== Smaller changes ==== * small drive-by cleanups to some CMake * `${_VCPKG_INSTALLED_DIR}/${VCPKG_TARGET_TRIPLET}` -> `${CURRENT_INSTALLED_DIR}` * Remove `-analyze` when compiling with clang-cl, since that's not a supported flag (vcpkg's build system) * Add a message about which compiler is detected by vcpkg's build system machinery * Fix `Expected::then` * Convert `""` to `{}` for `std::string` and `fs::path`, to avoid a `strlen` (additionally, `.empty()` instead of `== ""`, and `.clear()`) * Add `Strings::strto` which converts strings to numeric types * Support built-in arrays and `StringView` for `Strings::join` * Add `operator<` and friends to `StringView` * Add `substr` to `StringView` * SourceParagraphParser gets some new errors
167 lines
5.7 KiB
C++
167 lines
5.7 KiB
C++
#pragma once
|
|
|
|
#include <vcpkg/base/optional.h>
|
|
#include <vcpkg/base/util.h>
|
|
#include <vcpkg/build.h>
|
|
#include <vcpkg/cmakevars.h>
|
|
#include <vcpkg/packagespec.h>
|
|
#include <vcpkg/portfileprovider.h>
|
|
#include <vcpkg/statusparagraphs.h>
|
|
#include <vcpkg/vcpkgpaths.h>
|
|
|
|
#include <functional>
|
|
#include <map>
|
|
#include <vector>
|
|
|
|
namespace vcpkg::Graphs
|
|
{
|
|
struct Randomizer;
|
|
}
|
|
|
|
namespace vcpkg::Dependencies
|
|
{
|
|
enum class RequestType
|
|
{
|
|
UNKNOWN,
|
|
USER_REQUESTED,
|
|
AUTO_SELECTED
|
|
};
|
|
|
|
std::string to_output_string(RequestType request_type,
|
|
const CStringView s,
|
|
const Build::BuildPackageOptions& options);
|
|
std::string to_output_string(RequestType request_type, const CStringView s);
|
|
|
|
enum class InstallPlanType
|
|
{
|
|
UNKNOWN,
|
|
BUILD_AND_INSTALL,
|
|
ALREADY_INSTALLED,
|
|
EXCLUDED
|
|
};
|
|
|
|
struct InstallPlanAction : Util::MoveOnlyBase
|
|
{
|
|
static bool compare_by_name(const InstallPlanAction* left, const InstallPlanAction* right);
|
|
|
|
InstallPlanAction() noexcept;
|
|
|
|
InstallPlanAction(InstalledPackageView&& spghs, const RequestType& request_type);
|
|
|
|
InstallPlanAction(const PackageSpec& spec,
|
|
const SourceControlFileLocation& scfl,
|
|
const RequestType& request_type,
|
|
std::map<std::string, std::vector<FeatureSpec>>&& dependencies);
|
|
|
|
std::string displayname() const;
|
|
const std::string& public_abi() const;
|
|
const Build::PreBuildInfo& pre_build_info(LineInfo linfo) const;
|
|
|
|
PackageSpec spec;
|
|
|
|
Optional<const SourceControlFileLocation&> source_control_file_location;
|
|
Optional<InstalledPackageView> installed_package;
|
|
|
|
InstallPlanType plan_type;
|
|
RequestType request_type;
|
|
Build::BuildPackageOptions build_options;
|
|
|
|
std::map<std::string, std::vector<FeatureSpec>> feature_dependencies;
|
|
std::vector<PackageSpec> package_dependencies;
|
|
std::vector<std::string> feature_list;
|
|
|
|
Optional<Build::AbiInfo> abi_info;
|
|
};
|
|
|
|
enum class RemovePlanType
|
|
{
|
|
UNKNOWN,
|
|
NOT_INSTALLED,
|
|
REMOVE
|
|
};
|
|
|
|
struct RemovePlanAction : Util::MoveOnlyBase
|
|
{
|
|
static bool compare_by_name(const RemovePlanAction* left, const RemovePlanAction* right);
|
|
|
|
RemovePlanAction() noexcept;
|
|
RemovePlanAction(const PackageSpec& spec, const RemovePlanType& plan_type, const RequestType& request_type);
|
|
|
|
PackageSpec spec;
|
|
RemovePlanType plan_type;
|
|
RequestType request_type;
|
|
};
|
|
|
|
struct ActionPlan
|
|
{
|
|
bool empty() const { return remove_actions.empty() && already_installed.empty() && install_actions.empty(); }
|
|
size_t size() const { return remove_actions.size() + already_installed.size() + install_actions.size(); }
|
|
|
|
std::vector<RemovePlanAction> remove_actions;
|
|
std::vector<InstallPlanAction> already_installed;
|
|
std::vector<InstallPlanAction> install_actions;
|
|
};
|
|
|
|
enum class ExportPlanType
|
|
{
|
|
UNKNOWN,
|
|
NOT_BUILT,
|
|
ALREADY_BUILT
|
|
};
|
|
|
|
struct ExportPlanAction : Util::MoveOnlyBase
|
|
{
|
|
static bool compare_by_name(const ExportPlanAction* left, const ExportPlanAction* right);
|
|
|
|
ExportPlanAction() noexcept;
|
|
ExportPlanAction(const PackageSpec& spec,
|
|
InstalledPackageView&& installed_package,
|
|
const RequestType& request_type);
|
|
|
|
ExportPlanAction(const PackageSpec& spec, const RequestType& request_type);
|
|
|
|
PackageSpec spec;
|
|
ExportPlanType plan_type;
|
|
RequestType request_type;
|
|
|
|
Optional<const BinaryParagraph&> core_paragraph() const;
|
|
std::vector<PackageSpec> dependencies(Triplet triplet) const;
|
|
|
|
private:
|
|
Optional<InstalledPackageView> m_installed_package;
|
|
};
|
|
|
|
struct ClusterGraph;
|
|
|
|
struct CreateInstallPlanOptions
|
|
{
|
|
Graphs::Randomizer* randomizer = nullptr;
|
|
};
|
|
|
|
std::vector<RemovePlanAction> create_remove_plan(const std::vector<PackageSpec>& specs,
|
|
const StatusParagraphs& status_db);
|
|
|
|
std::vector<ExportPlanAction> create_export_plan(const std::vector<PackageSpec>& specs,
|
|
const StatusParagraphs& status_db);
|
|
|
|
/// <summary>Figure out which actions are required to install features specifications in `specs`.</summary>
|
|
/// <param name="provider">Contains the ports of the current environment.</param>
|
|
/// <param name="specs">Feature specifications to resolve dependencies for.</param>
|
|
/// <param name="status_db">Status of installed packages in the current environment.</param>
|
|
ActionPlan create_feature_install_plan(const PortFileProvider::PortFileProvider& provider,
|
|
const CMakeVars::CMakeVarProvider& var_provider,
|
|
const std::vector<FullPackageSpec>& specs,
|
|
const StatusParagraphs& status_db,
|
|
const CreateInstallPlanOptions& options = {});
|
|
|
|
ActionPlan create_upgrade_plan(const PortFileProvider::PortFileProvider& provider,
|
|
const CMakeVars::CMakeVarProvider& var_provider,
|
|
const std::vector<PackageSpec>& specs,
|
|
const StatusParagraphs& status_db,
|
|
const CreateInstallPlanOptions& options = {});
|
|
|
|
void print_plan(const ActionPlan& action_plan,
|
|
const bool is_recursive = true,
|
|
const fs::path& default_ports_dir = {});
|
|
}
|