mirror of
https://github.com/cemu-project/cemu_graphic_packs.git
synced 2024-11-21 17:19:18 +01:00
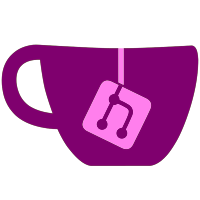
Didn't update the docs (will do that tomorrow), but I manually checked (didn't verify things, but I basically checked if it contained "uf_windowSpaceToClipSpaceTransform" and if the shader was made after a certain Cemu change was made due to how they're left out) to see if any graphic pack in here was *probably* safe. I also didn't convert 5 graphic packs since they contained signs that needed to be manually checked or at least examined more: - \Enhancements\TwilightPrincessHD_Bicubic - \Resolutions\DevilsThird_Resolution - \Resolutions\TwilightPrincessHD_Resolution (this one just needs to be fully verified since it's popular enough and has like 27 shaders) - \Resolutions\LegoStarWars_Resolution - \Resolutions\TokyoMirage_Resolution (this one could also be manually verified) I hope I didn't make too many mistakes with this one.
370 lines
16 KiB
Plaintext
370 lines
16 KiB
Plaintext
#version 420
|
|
#extension GL_ARB_texture_gather : enable
|
|
#extension GL_ARB_separate_shader_objects : enable
|
|
#ifdef VULKAN
|
|
#define ATTR_LAYOUT(__vkSet, __location) layout(set = __vkSet, location = __location)
|
|
#define UNIFORM_BUFFER_LAYOUT(__glLocation, __vkSet, __vkLocation) layout(set = __vkSet, binding = __vkLocation, std140)
|
|
#define TEXTURE_LAYOUT(__glLocation, __vkSet, __vkLocation) layout(set = __vkSet, binding = __vkLocation)
|
|
#define SET_POSITION(_v) gl_Position = _v; gl_Position.z = (gl_Position.z + gl_Position.w) / 2.0
|
|
#define GET_FRAGCOORD() vec4(gl_FragCoord.xy*uf_fragCoordScale.xy,gl_FragCoord.zw)
|
|
#define gl_VertexID gl_VertexIndex
|
|
#define gl_InstanceID gl_InstanceIndex
|
|
#else
|
|
#define ATTR_LAYOUT(__vkSet, __location) layout(location = __location)
|
|
#define UNIFORM_BUFFER_LAYOUT(__glLocation, __vkSet, __vkLocation) layout(binding = __glLocation, std140)
|
|
#define TEXTURE_LAYOUT(__glLocation, __vkSet, __vkLocation) layout(binding = __glLocation)
|
|
#define SET_POSITION(_v) gl_Position = _v
|
|
#define GET_FRAGCOORD() vec4(gl_FragCoord.xy*uf_fragCoordScale,gl_FragCoord.zw)
|
|
#endif
|
|
// This shader was automatically converted to be cross-compatible with Vulkan and OpenGL.
|
|
|
|
// shader 6ea8b1aa69c0b6f7
|
|
//cutscene contrasty
|
|
|
|
#ifdef VULKAN
|
|
layout(set = 1, binding = 7) uniform ufBlock
|
|
{
|
|
uniform ivec4 uf_remappedPS[5];
|
|
uniform vec4 uf_fragCoordScale;
|
|
};
|
|
#else
|
|
uniform ivec4 uf_remappedPS[5];
|
|
uniform vec2 uf_fragCoordScale;
|
|
#endif
|
|
|
|
const float hazeFactor = 0.1;
|
|
|
|
const float gamma = $gamma; // 1.0 is neutral Botw is already colour graded at this stage
|
|
const float exposure = $exposure; // 1.0 is neutral
|
|
const float vibrance = $vibrance; // 0.0 is neutral
|
|
const float crushContrast = $crushContrast; // 0.0 is neutral. Use small increments, loss of shadow detail
|
|
const float contrastCurve = $contrastCurve;
|
|
|
|
|
|
vec3 RGB_Lift = vec3($redShadows, $greenShadows , $blueShadows); // [0.000 to 2.000] Adjust shadows for Red, Green and Blue.
|
|
vec3 RGB_Gamma = vec3($redMid ,$greenMid, $blueMid); // [0.000 to 2.000] Adjust midtones for Red, Green and Blue
|
|
vec3 RGB_Gain = vec3($redHilight, $greenHilight, $blueHilight); // [0.000 to 2.000] Adjust highlights for Red, Green and Blue
|
|
//lumasharpen
|
|
const float sharp_mix = $sharp_mix;
|
|
const float sharp_strength = 2.0;
|
|
const float sharp_clamp = 0.75;
|
|
const float offset_bias = 1.0;
|
|
float Sigmoid (float x) {
|
|
|
|
return 1.0 / (1.0 + (exp(-(x - 0.5) * 5.5)));
|
|
}
|
|
|
|
|
|
#define px (1.0/1920.0*uf_fragCoordScale.x)
|
|
#define py (1.0/1080.0*uf_fragCoordScale.y)
|
|
#define CoefLuma vec3(0.2126, 0.7152, 0.0722)
|
|
|
|
float lumasharping(sampler2D tex, vec2 pos) {
|
|
vec4 colorInput = texture(tex, pos);
|
|
|
|
vec3 ori = colorInput.rgb;
|
|
|
|
// -- Combining the strength and luma multipliers --
|
|
vec3 sharp_strength_luma = (CoefLuma * sharp_strength);
|
|
|
|
// -- Gaussian filter --
|
|
// [ .25, .50, .25] [ 1 , 2 , 1 ]
|
|
// [ .50, 1, .50] = [ 2 , 4 , 2 ]
|
|
// [ .25, .50, .25] [ 1 , 2 , 1 ]
|
|
|
|
vec3 blur_ori = texture(tex, pos + vec2(px, -py) * 0.5 * offset_bias).rgb; // South East
|
|
blur_ori += texture(tex, pos + vec2(-px, -py) * 0.5 * offset_bias).rgb; // South West
|
|
blur_ori += texture(tex, pos + vec2(px, py) * 0.5 * offset_bias).rgb; // North East
|
|
blur_ori += texture(tex, pos + vec2(-px, py) * 0.5 * offset_bias).rgb; // North West
|
|
|
|
blur_ori *= 0.25; // ( /= 4) Divide by the number of texture fetches
|
|
|
|
// -- Calculate the sharpening --
|
|
vec3 sharp = ori - blur_ori; //Subtracting the blurred image from the original image
|
|
|
|
// -- Adjust strength of the sharpening and clamp it--
|
|
vec4 sharp_strength_luma_clamp = vec4(sharp_strength_luma * (0.5 / sharp_clamp), 0.5); //Roll part of the clamp into the dot
|
|
|
|
float sharp_luma = clamp((dot(vec4(sharp, 1.0), sharp_strength_luma_clamp)), 0.0, 1.0); //Calculate the luma, adjust the strength, scale up and clamp
|
|
sharp_luma = (sharp_clamp * 2.0) * sharp_luma - sharp_clamp; //scale down
|
|
|
|
return sharp_luma;
|
|
}
|
|
|
|
vec3 LiftGammaGainPass(vec3 colorInput)
|
|
{ //reshade BSD https://reshade.me , Alexkiri port
|
|
vec3 color = colorInput;
|
|
color = color * (1.5 - 0.5 * RGB_Lift) + 0.5 * RGB_Lift - 0.5;
|
|
color = clamp(color, 0.0, 1.0);
|
|
color *= RGB_Gain;
|
|
color = pow(color, 1.0 / RGB_Gamma);
|
|
return clamp(color, 0.0, 1.0);
|
|
}
|
|
|
|
vec3 contrasty(vec3 colour){
|
|
vec3 fColour = (colour.xyz);
|
|
//fColour = LiftGammaGainPass(fColour);
|
|
|
|
fColour = clamp(exposure * fColour, 0.0, 1.0);
|
|
fColour = pow(fColour, vec3(1.0 / gamma));
|
|
float luminance = fColour.r*0.299 + fColour.g*0.587 + fColour.b*0.114;
|
|
float mn = min(min(fColour.r, fColour.g), fColour.b);
|
|
float mx = max(max(fColour.r, fColour.g), fColour.b);
|
|
float sat = (1.0 - (mx - mn)) * (1.0 - mx) * luminance * 5.0;
|
|
vec3 lightness = vec3((mn + mx) / 2.0);
|
|
fColour = LiftGammaGainPass(fColour);
|
|
// vibrance
|
|
fColour = mix(fColour, mix(fColour, lightness, -vibrance), sat);
|
|
fColour = max(vec3(0.0), fColour - vec3(crushContrast));
|
|
return fColour;
|
|
}
|
|
|
|
|
|
// uf_remappedPS[5] was moved to the ufBlock
|
|
TEXTURE_LAYOUT(0, 1, 0) uniform sampler2D textureUnitPS0;
|
|
TEXTURE_LAYOUT(2, 1, 1) uniform sampler2D textureUnitPS2;
|
|
TEXTURE_LAYOUT(3, 1, 2) uniform sampler2D textureUnitPS3;
|
|
TEXTURE_LAYOUT(4, 1, 3) uniform sampler2D textureUnitPS4;
|
|
TEXTURE_LAYOUT(5, 1, 4) uniform sampler2D textureUnitPS5;
|
|
TEXTURE_LAYOUT(6, 1, 5) uniform sampler2D textureUnitPS6;
|
|
TEXTURE_LAYOUT(7, 1, 6) uniform sampler2D textureUnitPS7;
|
|
layout(location = 0) in vec4 passParameterSem128;
|
|
layout(location = 0) out vec4 passPixelColor0;
|
|
//uniform vec2 uf_fragCoordScale;
|
|
int clampFI32(int v)
|
|
{
|
|
if( v == 0x7FFFFFFF )
|
|
return floatBitsToInt(1.0);
|
|
else if( v == 0xFFFFFFFF )
|
|
return floatBitsToInt(0.0);
|
|
return floatBitsToInt(clamp(intBitsToFloat(v), 0.0, 1.0));
|
|
}
|
|
float mul_nonIEEE(float a, float b){return mix(0.0, a*b, (a != 0.0) && (b != 0.0));}
|
|
void main()
|
|
{
|
|
ivec4 R0i = ivec4(0);
|
|
ivec4 R1i = ivec4(0);
|
|
ivec4 R2i = ivec4(0);
|
|
ivec4 R3i = ivec4(0);
|
|
ivec4 R4i = ivec4(0);
|
|
ivec4 R5i = ivec4(0);
|
|
ivec4 R122i = ivec4(0);
|
|
ivec4 R123i = ivec4(0);
|
|
ivec4 R125i = ivec4(0);
|
|
ivec4 R126i = ivec4(0);
|
|
ivec4 R127i = ivec4(0);
|
|
int backupReg0i, backupReg1i, backupReg2i, backupReg3i, backupReg4i;
|
|
ivec4 PV0i = ivec4(0), PV1i = ivec4(0);
|
|
int PS0i = 0, PS1i = 0;
|
|
ivec4 tempi = ivec4(0);
|
|
float tempResultf;
|
|
int tempResulti;
|
|
ivec4 ARi = ivec4(0);
|
|
bool predResult = true;
|
|
vec3 cubeMapSTM;
|
|
int cubeMapFaceId;
|
|
R0i = floatBitsToInt(passParameterSem128);
|
|
R1i.x = floatBitsToInt(texture(textureUnitPS3, intBitsToFloat(R0i.xy)).x);
|
|
R4i.xyzw = floatBitsToInt(texture(textureUnitPS4, intBitsToFloat(R0i.xy)).xyzw);
|
|
R3i.xyzw = floatBitsToInt(texture(textureUnitPS0, intBitsToFloat(R0i.xy)).xyzw);
|
|
R2i.xyzw = floatBitsToInt(texture(textureUnitPS5, intBitsToFloat(R0i.xy)).xyzw);
|
|
R5i.xyz = floatBitsToInt(texture(textureUnitPS7, intBitsToFloat(R0i.xy)).xyz);
|
|
// 0
|
|
R0i.x = 0x3f000000;
|
|
R1i.y = 0x3f000000;
|
|
R0i.w = floatBitsToInt(intBitsToFloat(R4i.w) + -(0.5));
|
|
R1i.x = floatBitsToInt(texture(textureUnitPS2, intBitsToFloat(R1i.xy)).w);
|
|
R0i.x = floatBitsToInt(texture(textureUnitPS6, intBitsToFloat(R0i.xx)).x);
|
|
// 0
|
|
PV0i.x = floatBitsToInt(intBitsToFloat(R1i.x) + -(0.5));
|
|
R127i.y = ((0.5 > intBitsToFloat(R1i.x))?int(0xFFFFFFFF):int(0x0));
|
|
PV0i.y = R127i.y;
|
|
PV0i.z = floatBitsToInt(max(intBitsToFloat(R0i.w), -(intBitsToFloat(R0i.w))));
|
|
PV0i.z = floatBitsToInt(intBitsToFloat(PV0i.z) * 2.0);
|
|
// 1
|
|
R123i.x = ((PV0i.y == 0)?(R2i.x):(R3i.x));
|
|
PV1i.x = R123i.x;
|
|
PV1i.y = floatBitsToInt(-(intBitsToFloat(PV0i.z)) + 1.0);
|
|
PV1i.y = clampFI32(PV1i.y);
|
|
R123i.z = ((PV0i.y == 0)?(R2i.y):(R3i.y));
|
|
PV1i.z = R123i.z;
|
|
PV1i.w = floatBitsToInt(max(intBitsToFloat(PV0i.x), -(intBitsToFloat(PV0i.x))));
|
|
PV1i.w = floatBitsToInt(intBitsToFloat(PV1i.w) * 2.0);
|
|
R122i.x = ((PV0i.y == 0)?(R2i.z):(R3i.z));
|
|
PS1i = R122i.x;
|
|
// 2
|
|
PV0i.x = floatBitsToInt(intBitsToFloat(PV1i.y) + intBitsToFloat(0x3727c5ac));
|
|
R126i.y = floatBitsToInt(-(intBitsToFloat(R3i.y)) + intBitsToFloat(PV1i.z));
|
|
PV0i.z = floatBitsToInt(-(intBitsToFloat(PV1i.w)) + 1.0);
|
|
PV0i.z = clampFI32(PV0i.z);
|
|
R127i.w = floatBitsToInt(-(intBitsToFloat(R3i.x)) + intBitsToFloat(PV1i.x));
|
|
R125i.y = floatBitsToInt(-(intBitsToFloat(R3i.z)) + intBitsToFloat(PS1i));
|
|
PS0i = R125i.y;
|
|
// 3
|
|
R123i.x = ((R127i.y == 0)?(R2i.w):(R3i.w));
|
|
PV1i.x = R123i.x;
|
|
PV1i.y = floatBitsToInt(intBitsToFloat(PV0i.z) + intBitsToFloat(0x3727c5ac));
|
|
PS1i = floatBitsToInt(1.0 / intBitsToFloat(PV0i.x));
|
|
// 4
|
|
R127i.x = floatBitsToInt(-(intBitsToFloat(R3i.w)) + intBitsToFloat(PV1i.x));
|
|
PV0i.z = floatBitsToInt(intBitsToFloat(PS1i) + -(1.0));
|
|
PS0i = floatBitsToInt(1.0 / intBitsToFloat(PV1i.y));
|
|
// 5
|
|
PV1i.y = floatBitsToInt(intBitsToFloat(PV0i.z) + 4.0);
|
|
PV1i.w = floatBitsToInt(intBitsToFloat(PS0i) + -(1.0));
|
|
// 6
|
|
PV0i.x = floatBitsToInt(intBitsToFloat(PV1i.y) + -(4.0));
|
|
PV0i.z = floatBitsToInt(intBitsToFloat(PV1i.w) + 4.0);
|
|
// 7
|
|
PV1i.y = floatBitsToInt(intBitsToFloat(PV0i.z) + -(4.0));
|
|
R126i.w = floatBitsToInt(intBitsToFloat(PV0i.x) * 0.25);
|
|
R126i.w = clampFI32(R126i.w);
|
|
// 8
|
|
PV0i.x = floatBitsToInt(intBitsToFloat(PV1i.y) * 0.25);
|
|
PV0i.x = clampFI32(PV0i.x);
|
|
// 9
|
|
backupReg0i = R127i.x;
|
|
backupReg1i = R126i.y;
|
|
backupReg2i = R127i.w;
|
|
R127i.x = floatBitsToInt((mul_nonIEEE(intBitsToFloat(backupReg0i),intBitsToFloat(PV0i.x)) + intBitsToFloat(R3i.w)));
|
|
PV1i.x = R127i.x;
|
|
R126i.y = floatBitsToInt((mul_nonIEEE(intBitsToFloat(R125i.y),intBitsToFloat(PV0i.x)) + intBitsToFloat(R3i.z)));
|
|
PV1i.y = R126i.y;
|
|
R127i.z = floatBitsToInt((mul_nonIEEE(intBitsToFloat(backupReg1i),intBitsToFloat(PV0i.x)) + intBitsToFloat(R3i.y)));
|
|
PV1i.z = R127i.z;
|
|
R127i.w = floatBitsToInt((mul_nonIEEE(intBitsToFloat(backupReg2i),intBitsToFloat(PV0i.x)) + intBitsToFloat(R3i.x)));
|
|
PV1i.w = R127i.w;
|
|
// 10
|
|
PV0i.x = floatBitsToInt(intBitsToFloat(R4i.z) + -(intBitsToFloat(PV1i.y)));
|
|
PV0i.y = floatBitsToInt(intBitsToFloat(R4i.y) + -(intBitsToFloat(PV1i.z)));
|
|
PV0i.z = floatBitsToInt(intBitsToFloat(R4i.x) + -(intBitsToFloat(PV1i.w)));
|
|
PV0i.w = floatBitsToInt(intBitsToFloat(R4i.w) + -(intBitsToFloat(PV1i.x)));
|
|
// 11
|
|
R123i.x = floatBitsToInt((mul_nonIEEE(intBitsToFloat(PV0i.w),intBitsToFloat(R126i.w)) + intBitsToFloat(R127i.x)));
|
|
PV1i.x = R123i.x;
|
|
R123i.y = floatBitsToInt((mul_nonIEEE(intBitsToFloat(PV0i.y),intBitsToFloat(R126i.w)) + intBitsToFloat(R127i.z)));
|
|
PV1i.y = R123i.y;
|
|
R123i.z = floatBitsToInt((mul_nonIEEE(intBitsToFloat(PV0i.z),intBitsToFloat(R126i.w)) + intBitsToFloat(R127i.w)));
|
|
PV1i.z = R123i.z;
|
|
R123i.w = floatBitsToInt((mul_nonIEEE(intBitsToFloat(PV0i.x),intBitsToFloat(R126i.w)) + intBitsToFloat(R126i.y)));
|
|
PV1i.w = R123i.w;
|
|
// 12
|
|
R127i.x = floatBitsToInt(mul_nonIEEE(intBitsToFloat(PV1i.z), intBitsToFloat(R0i.x)));
|
|
PV0i.x = R127i.x;
|
|
PV0i.y = floatBitsToInt(mul_nonIEEE(intBitsToFloat(PV1i.x), intBitsToFloat(R0i.x)));
|
|
R126i.z = floatBitsToInt(mul_nonIEEE(intBitsToFloat(PV1i.w), intBitsToFloat(R0i.x)));
|
|
PV0i.z = R126i.z;
|
|
R127i.w = floatBitsToInt(mul_nonIEEE(intBitsToFloat(PV1i.y), intBitsToFloat(R0i.x)));
|
|
PV0i.w = R127i.w;
|
|
// 13
|
|
PV1i.x = floatBitsToInt(intBitsToFloat(PV0i.x) * intBitsToFloat(0x3e6147ae));
|
|
R127i.z = floatBitsToInt(intBitsToFloat(PV0i.z) * intBitsToFloat(0x3e6147ae));
|
|
PV1i.z = R127i.z;
|
|
PV1i.w = floatBitsToInt(intBitsToFloat(PV0i.w) * intBitsToFloat(0x3e6147ae));
|
|
R0i.w = floatBitsToInt(mul_nonIEEE(intBitsToFloat(PV0i.y), intBitsToFloat(uf_remappedPS[0].x)));
|
|
PS1i = R0i.w;
|
|
// 14
|
|
PV0i.x = floatBitsToInt(intBitsToFloat(PV1i.w) + intBitsToFloat(0x3e99999a));
|
|
PV0i.y = floatBitsToInt(intBitsToFloat(PV1i.x) + intBitsToFloat(0x3e99999a));
|
|
PV0i.z = floatBitsToInt(intBitsToFloat(PV1i.x) + intBitsToFloat(0x3cf5c28f));
|
|
PV0i.w = floatBitsToInt(intBitsToFloat(PV1i.z) + intBitsToFloat(0x3e99999a));
|
|
R125i.z = floatBitsToInt(intBitsToFloat(PV1i.w) + intBitsToFloat(0x3cf5c28f));
|
|
PS0i = R125i.z;
|
|
// 15
|
|
backupReg0i = R127i.x;
|
|
R126i.x = floatBitsToInt((mul_nonIEEE(intBitsToFloat(R126i.z),intBitsToFloat(PV0i.w)) + intBitsToFloat(0x3d75c28f)));
|
|
PV1i.y = floatBitsToInt(intBitsToFloat(R127i.z) + intBitsToFloat(0x3cf5c28f));
|
|
R123i.z = floatBitsToInt((mul_nonIEEE(intBitsToFloat(R127i.x),intBitsToFloat(PV0i.y)) + intBitsToFloat(0x3d75c28f)));
|
|
PV1i.z = R123i.z;
|
|
R126i.w = floatBitsToInt((mul_nonIEEE(intBitsToFloat(R127i.w),intBitsToFloat(PV0i.x)) + intBitsToFloat(0x3d75c28f)));
|
|
R127i.x = floatBitsToInt((mul_nonIEEE(intBitsToFloat(backupReg0i),intBitsToFloat(PV0i.z)) + intBitsToFloat(0x3b03126f)));
|
|
PS1i = R127i.x;
|
|
// 16
|
|
backupReg0i = R126i.z;
|
|
backupReg1i = R127i.w;
|
|
R126i.z = floatBitsToInt((mul_nonIEEE(intBitsToFloat(backupReg0i),intBitsToFloat(PV1i.y)) + intBitsToFloat(0x3b03126f)));
|
|
R127i.w = floatBitsToInt((mul_nonIEEE(intBitsToFloat(backupReg1i),intBitsToFloat(R125i.z)) + intBitsToFloat(0x3b03126f)));
|
|
PS0i = floatBitsToInt(1.0 / intBitsToFloat(PV1i.z));
|
|
// 17
|
|
PV1i.z = floatBitsToInt(intBitsToFloat(R127i.x) * intBitsToFloat(PS0i));
|
|
PS1i = floatBitsToInt(1.0 / intBitsToFloat(R126i.w));
|
|
// 18
|
|
PV0i.y = floatBitsToInt(intBitsToFloat(R127i.w) * intBitsToFloat(PS1i));
|
|
PV0i.w = floatBitsToInt(intBitsToFloat(PV1i.z) + -(intBitsToFloat(0x3d086595)));
|
|
PS0i = floatBitsToInt(1.0 / intBitsToFloat(R126i.x));
|
|
// 19
|
|
PV1i.x = floatBitsToInt(intBitsToFloat(R126i.z) * intBitsToFloat(PS0i));
|
|
PV1i.y = floatBitsToInt(mul_nonIEEE(intBitsToFloat(PV0i.w), intBitsToFloat(uf_remappedPS[0].x)));
|
|
PV1i.z = floatBitsToInt(intBitsToFloat(PV0i.y) + -(intBitsToFloat(0x3d086595)));
|
|
// 20
|
|
R126i.x = floatBitsToInt((mul_nonIEEE(intBitsToFloat(R5i.x),intBitsToFloat(uf_remappedPS[1].x)) + intBitsToFloat(PV1i.y)));
|
|
PV0i.y = floatBitsToInt(intBitsToFloat(PV1i.x) + -(intBitsToFloat(0x3d086595)));
|
|
PV0i.w = floatBitsToInt(mul_nonIEEE(intBitsToFloat(PV1i.z), intBitsToFloat(uf_remappedPS[0].x)));
|
|
// 21
|
|
R123i.x = floatBitsToInt((mul_nonIEEE(intBitsToFloat(R5i.y),intBitsToFloat(uf_remappedPS[1].x)) + intBitsToFloat(PV0i.w)));
|
|
PV1i.x = R123i.x;
|
|
PV1i.z = floatBitsToInt(mul_nonIEEE(intBitsToFloat(PV0i.y), intBitsToFloat(uf_remappedPS[0].x)));
|
|
// 22
|
|
backupReg0i = R126i.x;
|
|
R126i.x = floatBitsToInt(mul_nonIEEE(intBitsToFloat(backupReg0i), intBitsToFloat(uf_remappedPS[2].x)));
|
|
R126i.y = floatBitsToInt(mul_nonIEEE(intBitsToFloat(PV1i.x), intBitsToFloat(uf_remappedPS[2].y)));
|
|
R123i.w = floatBitsToInt((mul_nonIEEE(intBitsToFloat(R5i.z),intBitsToFloat(uf_remappedPS[1].x)) + intBitsToFloat(PV1i.z)));
|
|
PV0i.w = R123i.w;
|
|
// 23
|
|
R126i.z = floatBitsToInt(mul_nonIEEE(intBitsToFloat(PV0i.w), intBitsToFloat(uf_remappedPS[2].z)));
|
|
PV1i.z = R126i.z;
|
|
// 24
|
|
tempi.x = floatBitsToInt(dot(vec4(intBitsToFloat(R126i.x),intBitsToFloat(R126i.y),intBitsToFloat(PV1i.z),-0.0),vec4(intBitsToFloat(0x3e990abb),intBitsToFloat(0x3f162c13),intBitsToFloat(0x3dea747e),0.0)));
|
|
PV0i.x = tempi.x;
|
|
PV0i.y = tempi.x;
|
|
PV0i.z = tempi.x;
|
|
PV0i.w = tempi.x;
|
|
R125i.z = tempi.x;
|
|
// 25
|
|
PV1i.x = floatBitsToInt(intBitsToFloat(R126i.y) + -(intBitsToFloat(PV0i.x)));
|
|
PV1i.y = floatBitsToInt(intBitsToFloat(R126i.x) + -(intBitsToFloat(PV0i.x)));
|
|
PV1i.w = floatBitsToInt(intBitsToFloat(R126i.z) + -(intBitsToFloat(PV0i.x)));
|
|
// 26
|
|
R126i.x = floatBitsToInt((mul_nonIEEE(intBitsToFloat(PV1i.w),intBitsToFloat(uf_remappedPS[3].z)) + intBitsToFloat(R125i.z)));
|
|
R126i.y = floatBitsToInt((mul_nonIEEE(intBitsToFloat(PV1i.x),intBitsToFloat(uf_remappedPS[3].y)) + intBitsToFloat(R125i.z)));
|
|
R123i.z = floatBitsToInt((mul_nonIEEE(intBitsToFloat(PV1i.y),intBitsToFloat(uf_remappedPS[3].x)) + intBitsToFloat(R125i.z)));
|
|
PV0i.z = R123i.z;
|
|
// 27
|
|
tempResultf = log2(intBitsToFloat(PV0i.z));
|
|
if( isinf(tempResultf) == true ) tempResultf = -3.40282347E+38F;
|
|
PS1i = floatBitsToInt(tempResultf);
|
|
// 28
|
|
R125i.z = floatBitsToInt(mul_nonIEEE(intBitsToFloat(PS1i), intBitsToFloat(uf_remappedPS[4].x)));
|
|
tempResultf = log2(intBitsToFloat(R126i.y));
|
|
if( isinf(tempResultf) == true ) tempResultf = -3.40282347E+38F;
|
|
PS0i = floatBitsToInt(tempResultf);
|
|
// 29
|
|
R127i.w = floatBitsToInt(mul_nonIEEE(intBitsToFloat(PS0i), intBitsToFloat(uf_remappedPS[4].x)));
|
|
tempResultf = log2(intBitsToFloat(R126i.x));
|
|
if( isinf(tempResultf) == true ) tempResultf = -3.40282347E+38F;
|
|
PS1i = floatBitsToInt(tempResultf);
|
|
// 30
|
|
R126i.x = floatBitsToInt(mul_nonIEEE(intBitsToFloat(PS1i), intBitsToFloat(uf_remappedPS[4].x)));
|
|
PS0i = floatBitsToInt(exp2(intBitsToFloat(R125i.z)));
|
|
// 31
|
|
R0i.x = PS0i;
|
|
PS1i = floatBitsToInt(exp2(intBitsToFloat(R127i.w)));
|
|
// 32
|
|
R0i.y = PS1i;
|
|
R4i.w = floatBitsToInt(exp2(intBitsToFloat(R126i.x)));
|
|
PS0i = R4i.w;
|
|
// 33
|
|
R0i.z = R4i.w;
|
|
|
|
|
|
// export
|
|
passPixelColor0 = vec4(intBitsToFloat(R0i.x), intBitsToFloat(R0i.y), intBitsToFloat(R0i.z), intBitsToFloat(R0i.w));
|
|
|
|
passPixelColor0.xyz = contrasty(passPixelColor0.xyz);
|
|
passPixelColor0.xyz = mix(passPixelColor0.xyz, smoothstep(0.0, 1.0, passPixelColor0.xyz), contrastCurve);
|
|
float smask = lumasharping(textureUnitPS0, passParameterSem128.xy);
|
|
vec3 temp3 = passPixelColor0.xyz;
|
|
passPixelColor0.xyz = mix(passPixelColor0.xyz, (temp3.xyz += (smask)), sharp_mix);
|
|
|
|
}
|