mirror of
https://github.com/cemu-project/cemu_graphic_packs.git
synced 2024-11-22 17:49:17 +01:00
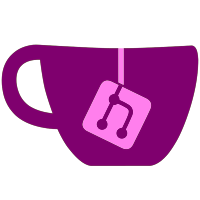
Since it's not possible to update 300+ shaders manually and automation was possible, I thought that I'd take the honor and create a script that's able to automatically convert all of the shaders to be cross-compatible with Vulkan. And change the graphic pack versions to version 4 of course. Also, the script has some nifty testing code which compiled every shader as OpenGL and Vulkan, but for that see the details that I've written below. **Here's the script that I've made to do all of this. No manual edits were needed:** https://gist.github.com/Crementif/8d98a855b95f219d95298fb3db99deae
52 lines
2.1 KiB
Plaintext
52 lines
2.1 KiB
Plaintext
#version 420
|
|
#extension GL_ARB_texture_gather : enable
|
|
#extension GL_ARB_arrays_of_arrays : enable
|
|
#ifdef VULKAN
|
|
#define ATTR_LAYOUT(__vkSet, __location) layout(set = __vkSet, location = __location)
|
|
#define UNIFORM_BUFFER_LAYOUT(__glLocation, __vkSet, __vkLocation) layout(set = __vkSet, binding = __vkLocation, std140)
|
|
#define TEXTURE_LAYOUT(__glLocation, __vkSet, __vkLocation) layout(set = __vkSet, binding = __vkLocation)
|
|
#define SET_POSITION(_v) gl_Position = _v; gl_Position.z = (gl_Position.z + gl_Position.w) / 2.0
|
|
#define GET_FRAGCOORD() vec4(gl_FragCoord.xy*uf_fragCoordScale.xy,gl_FragCoord.zw)
|
|
#define gl_VertexID gl_VertexIndex
|
|
#define gl_InstanceID gl_InstanceIndex
|
|
#else
|
|
#define ATTR_LAYOUT(__vkSet, __location) layout(location = __location)
|
|
#define UNIFORM_BUFFER_LAYOUT(__glLocation, __vkSet, __vkLocation) layout(binding = __glLocation, std140)
|
|
#define TEXTURE_LAYOUT(__glLocation, __vkSet, __vkLocation) layout(binding = __glLocation)
|
|
#define SET_POSITION(_v) gl_Position = _v
|
|
#define GET_FRAGCOORD() vec4(gl_FragCoord.xy*uf_fragCoordScale,gl_FragCoord.zw)
|
|
#endif
|
|
// This shaders was auto-converted from OpenGL to Cemu so expect weird code and possible errors.
|
|
|
|
// shader cb0e6e8cbec4502a
|
|
// DoF blur effect - Battle, Camera, Scope
|
|
TEXTURE_LAYOUT(0, 1, 0) uniform sampler2D textureUnitPS0;// Tex0 addr 0xf5c7b800 res 1280x720x1 dim 1 tm: 4 format 0816 compSel: 0 1 2 5 mipView: 0x0 (num 0x1
|
|
layout(location = 0) in vec4 passParameterSem3;
|
|
layout(location = 0) out vec4 passPixelColor0;
|
|
#ifdef VULKAN
|
|
layout(set = 1, binding = 1) uniform ufBlock
|
|
{
|
|
uniform vec4 uf_fragCoordScale;
|
|
};
|
|
#else
|
|
uniform vec2 uf_fragCoordScale;
|
|
#endif
|
|
|
|
const float resScale = 0;
|
|
const int radius = int(2*resScale);
|
|
|
|
void main() {
|
|
vec2 center = (passParameterSem3.xy + passParameterSem3.zw) / 2;
|
|
vec2 step = passParameterSem3.xw - passParameterSem3.zy;
|
|
vec4 result = vec4(0.0);
|
|
float count = 0.0;
|
|
for (int x = -radius; x <= radius; x++) {
|
|
for (int y = -radius; y <= radius; y++) {
|
|
if (length(vec2(x, y)) <= radius) {
|
|
result += texture(textureUnitPS0, center + vec2(x, y)*step);
|
|
count += 1.0;
|
|
}
|
|
}
|
|
}
|
|
passPixelColor0 = result / count;
|
|
} |