mirror of
https://github.com/cemu-project/cemu_graphic_packs.git
synced 2024-11-22 09:39:17 +01:00
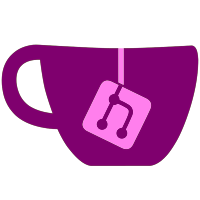
TPHD QL tweaks aka, sunday gaming delayed Resolution * Sharper, unscaled blur fixed. See contrasty note * Resolutions 4k and above scale sub viewports 75-> 50% of the main res. 7680x4320 is now 30 fps stable on a 1070, SSAA looks sweet. * Added some off-aspect custom res with horizontal res x2. Good for SSAA fixing shimmering as it's mostly horizontal in TPHD Contrasty * Sharper, unscaled blur fixed. Note, contrasty must be pre-selected at start to override same shader in resolution folder * Minor tweaks to cold setting Fancy FX *Added option to disable blur, just keeping other tweaks active. Realized that half scaling sub-view port mostly replaces fancy fX :/ *Tweaked to somewhat scale, tries to emulate original look by default. Ie 4x res = 4x blur "qualitative look" (not the same as nr of passes) *Added "defocus" looks blurrier by scaling incorrectly, masked by more draw passes. This is similar to the old default Not happy about the results of moving blur from res folder, too many drawbacks as quality of scaling really needs to be per-setting. Will probably deprecate if things start to break again. Maybe global variables in the future?
92 lines
2.9 KiB
Plaintext
92 lines
2.9 KiB
Plaintext
#version 420
|
|
#extension GL_ARB_texture_gather : enable
|
|
#extension GL_ARB_separate_shader_objects : enable
|
|
// shader e334517825fdd599
|
|
//basline dof blur..
|
|
|
|
const float scaleBlur = $scaleBlur; //0.125 4k
|
|
const int sampleScaling = $presetPass;
|
|
|
|
#define EnableBlur $enableBlur
|
|
|
|
layout(binding = 0) uniform sampler2D textureUnitPS0;// Tex0 addr 0xf4001000 res 1920x1080x1 dim 1 tm: 4 format 001a compSel: 0 1 2 3 mipView: 0x0 (num 0x1) sliceView: 0x0 (num 0x1) Sampler0 ClampX/Y/Z: 2 2 2 border: 0
|
|
layout(location = 0) in vec4 passParameterSem0;
|
|
layout(location = 0) out vec4 passPixelColor0;
|
|
uniform vec2 uf_fragCoordScale;
|
|
|
|
/* Nr of pass hack since we don't know what the res pack set to after moving shader from main pack :(
|
|
* Would have been better to manually tweak per res setting , and then add static scaler on top of that.
|
|
* ~1080 -> 2 pass, 1440->3 , 4k -> 5
|
|
*/
|
|
|
|
const int sampleScale = 2 + int(1.0/uf_fragCoordScale.x) + sampleScaling;
|
|
|
|
//const int sampleScale = 2 + sampleScaling;
|
|
// FabriceNeyret2 CC, single shader gaussian by intermediate MIPmap level. www.shadertoy.com/view/ltScRG
|
|
const int samples = 4 * sampleScale, //8 or 4 balances xy position + brightness hack
|
|
LOD = 2, // gaussian done on MIPmap at scale LOD
|
|
sLOD = 1 << LOD; // tile size = 2^LOD
|
|
const float sigma = float(samples) * .25;
|
|
|
|
float gaussian(vec2 i) {
|
|
return exp(-.5* dot(i /= sigma, i)) / (6.28 * sigma*sigma);
|
|
}
|
|
|
|
vec4 blur(sampler2D sp, vec2 U, vec2 scale) {
|
|
vec4 O = vec4(0.0);
|
|
int s = samples / sLOD;
|
|
|
|
for (int i = 0; i < s*s; i++) {
|
|
vec2 d = vec2(i%s, i / s)*float(sLOD) - float(samples) / 2.;
|
|
O += gaussian(d) * textureLod(sp, U + scale * d, float(LOD));
|
|
}
|
|
|
|
//return O / O.a;
|
|
return vec4(O.x, O.y, O.z, 0.0)*20.0; // balance loss of brightness
|
|
}
|
|
|
|
int clampFI32(int v)
|
|
{
|
|
if( v == 0x7FFFFFFF )
|
|
return floatBitsToInt(1.0);
|
|
else if( v == 0xFFFFFFFF )
|
|
return floatBitsToInt(0.0);
|
|
return floatBitsToInt(clamp(intBitsToFloat(v), 0.0, 1.0));
|
|
}
|
|
float mul_nonIEEE(float a, float b){return mix(0.0, a*b, (a != 0.0) && (b != 0.0));}
|
|
void main()
|
|
{
|
|
vec4 R0f = vec4(0.0);
|
|
vec4 R9f = vec4(0.0);
|
|
float backupReg0f, backupReg1f, backupReg2f, backupReg3f, backupReg4f;
|
|
vec4 PV0f = vec4(0.0), PV1f = vec4(0.0);
|
|
float PS0f = 0.0, PS1f = 0.0;
|
|
vec4 tempf = vec4(0.0);
|
|
float tempResultf;
|
|
int tempResulti;
|
|
ivec4 ARi = ivec4(0);
|
|
bool predResult = true;
|
|
vec3 cubeMapSTM;
|
|
int cubeMapFaceId;
|
|
R0f = passParameterSem0;
|
|
|
|
|
|
#if (EnableBlur == 1)
|
|
vec2 scaler = uf_fragCoordScale.xy;
|
|
vec2 coord = R0f.xy*textureSize(textureUnitPS0, 0); //
|
|
vec2 ps = vec2(1.0) / textureSize(textureUnitPS0, 0);
|
|
vec2 uv = coord * ps;
|
|
|
|
R9f.xyz = blur(textureUnitPS0, R0f.xy, ps*0.5*scaleBlur*scaler).xyz;
|
|
R0f.w = (textureLod(textureUnitPS0, R0f.xy,0.0).w);
|
|
// export
|
|
passPixelColor0 = vec4(R9f.x, R9f.y, R9f.z, R0f.w);
|
|
|
|
#elif (EnableBlur == 0)
|
|
R0f.xyzw = (textureLod(textureUnitPS0, R0f.xy,0.0).xyzw);
|
|
// export
|
|
passPixelColor0 = vec4(R0f.x, R0f.y, R0f.z, R0f.w);
|
|
#endif
|
|
|
|
}
|