mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-01-15 18:49:11 +01:00
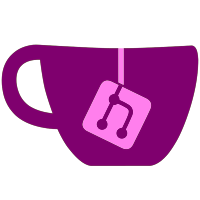
Main Issues: DX11 is functional with a ~2MB/s mem leak. OpenGL/DirectX9 have a black display while game runs. (DirectX 9 flashes good display on emulation stop) Too many virtual function calls. (once everything is working, I will work on removing them) Won't build on non-Windows in its current state. (mainly EmuWindow will need changes for Linux/OS X) Probably other stuff. git-svn-id: https://dolphin-emu.googlecode.com/svn/trunk@6219 8ced0084-cf51-0410-be5f-012b33b47a6e
81 lines
2.2 KiB
C++
81 lines
2.2 KiB
C++
|
|
#ifndef _TEXTURECACHE_H_
|
|
#define _TEXTURECACHE_H_
|
|
|
|
#include <map>
|
|
|
|
#include "CommonTypes.h"
|
|
#include "VideoCommon.h"
|
|
#include "TextureDecoder.h"
|
|
|
|
unsigned int GetPow2(unsigned int val);
|
|
|
|
class TextureCacheBase
|
|
{
|
|
public:
|
|
struct TCacheEntryBase
|
|
{
|
|
u32 addr;
|
|
u32 size_in_bytes;
|
|
u64 hash;
|
|
u32 paletteHash;
|
|
u32 oldpixel;
|
|
|
|
int frameCount;
|
|
unsigned int w, h, fmt, MipLevels;
|
|
int Scaledw, Scaledh;
|
|
|
|
bool isRenderTarget;
|
|
bool isNonPow2;
|
|
|
|
TCacheEntryBase() : addr(0), size_in_bytes(0), hash(0), paletteHash(0), oldpixel(0),
|
|
frameCount(0), w(0), h(0), fmt(0), MipLevels(0), Scaledw(0), Scaledh(0),
|
|
isRenderTarget(false), isNonPow2(true) {}
|
|
|
|
virtual ~TCacheEntryBase();
|
|
|
|
virtual void Load(unsigned int width, unsigned int height,
|
|
unsigned int expanded_width, unsigned int levels) = 0;
|
|
|
|
virtual void FromRenderTarget(bool bFromZBuffer, bool bScaleByHalf,
|
|
unsigned int cbufid, const float colmat[], const EFBRectangle &source_rect) = 0;
|
|
|
|
virtual void Bind(unsigned int stage) = 0;
|
|
|
|
bool IntersectsMemoryRange(u32 range_address, u32 range_size) const;
|
|
};
|
|
|
|
friend struct TCacheEntryBase;
|
|
|
|
TextureCacheBase();
|
|
virtual ~TextureCacheBase();
|
|
|
|
static void Cleanup();
|
|
|
|
static void Invalidate(bool shutdown);
|
|
static void InvalidateRange(u32 start_address, u32 size);
|
|
|
|
// TODO: make these 2 static?
|
|
TCacheEntryBase* Load(unsigned int stage, u32 address, unsigned int width,
|
|
unsigned int height, unsigned int tex_format, unsigned int tlutaddr,
|
|
unsigned int tlutfmt, bool UseNativeMips, unsigned int maxlevel);
|
|
|
|
void CopyRenderTargetToTexture(u32 address, bool bFromZBuffer, bool bIsIntensityFmt,
|
|
u32 copyfmt, bool bScaleByHalf, const EFBRectangle &source_rect);
|
|
|
|
virtual TCacheEntryBase* CreateTexture(unsigned int width, unsigned int height,
|
|
unsigned int expanded_width, unsigned int tex_levels, PC_TexFormat pcfmt) = 0;
|
|
|
|
virtual TCacheEntryBase* CreateRenderTargetTexture(int scaled_tex_w, int scaled_tex_h) = 0;
|
|
|
|
static void ClearRenderTargets();
|
|
static void MakeRangeDynamic(u32 start_address, u32 size);
|
|
|
|
protected:
|
|
typedef std::map<u32, TCacheEntryBase*> TexCache;
|
|
static TexCache textures;
|
|
static u8 *temp;
|
|
};
|
|
|
|
#endif
|