mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-01-18 20:11:16 +01:00
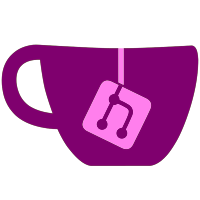
This saves us from having to update the GameFileCache when the TitleDatabase changes (for instance when the user changes language).
42 lines
1.1 KiB
C++
42 lines
1.1 KiB
C++
// Copyright 2017 Dolphin Emulator Project
|
|
// Licensed under GPLv2+
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <string>
|
|
#include <unordered_map>
|
|
|
|
#include "Common/CommonTypes.h"
|
|
|
|
namespace Core
|
|
{
|
|
// Reader for title database files.
|
|
class TitleDatabase final
|
|
{
|
|
public:
|
|
TitleDatabase();
|
|
~TitleDatabase();
|
|
|
|
enum class TitleType
|
|
{
|
|
Channel,
|
|
Other,
|
|
};
|
|
|
|
// Get a user friendly title name for a game ID.
|
|
// This falls back to returning an empty string if none could be found.
|
|
const std::string& GetTitleName(const std::string& game_id, TitleType = TitleType::Other) const;
|
|
|
|
// Same as above, but takes a title ID instead of a game ID, and can only find names of channels.
|
|
const std::string& GetChannelName(u64 title_id) const;
|
|
|
|
// Get a description for a game ID (title name if available + game ID).
|
|
std::string Describe(const std::string& game_id, TitleType = TitleType::Other) const;
|
|
|
|
private:
|
|
std::unordered_map<std::string, std::string> m_wii_title_map;
|
|
std::unordered_map<std::string, std::string> m_gc_title_map;
|
|
};
|
|
} // namespace Core
|