mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-01-11 00:29:11 +01:00
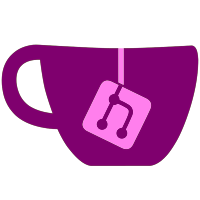
Before, it used a fallback where it returned a default object, where the width and height were set to 0. Presenter::Initialize() used GetSurfaceInfo to set the backbuffer size, then used that size when initializing the on-screen UI (even for the software renderer, where the on-screen UI isn't currently present), which meant that ImGui got a window size of 0 and thus resulted in a failed assertion. Although BindBackbuffer checks for size changes, it doesn't help because ImGui has already been initialized, and the size hasn't actually changed since initialization occured. Fixes one aspect of https://bugs.dolphin-emu.org/issues/13172.
59 lines
2.3 KiB
C++
59 lines
2.3 KiB
C++
// Copyright 2023 Dolphin Emulator Project
|
|
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
|
|
#pragma once
|
|
|
|
#include "VideoCommon/AbstractGfx.h"
|
|
|
|
class SWOGLWindow;
|
|
|
|
namespace SW
|
|
{
|
|
class SWGfx final : public AbstractGfx
|
|
{
|
|
public:
|
|
SWGfx(std::unique_ptr<SWOGLWindow> window);
|
|
|
|
bool IsHeadless() const override;
|
|
virtual bool SupportsUtilityDrawing() const override;
|
|
|
|
std::unique_ptr<AbstractTexture> CreateTexture(const TextureConfig& config,
|
|
std::string_view name) override;
|
|
std::unique_ptr<AbstractStagingTexture>
|
|
CreateStagingTexture(StagingTextureType type, const TextureConfig& config) override;
|
|
std::unique_ptr<AbstractFramebuffer>
|
|
CreateFramebuffer(AbstractTexture* color_attachment, AbstractTexture* depth_attachment) override;
|
|
|
|
void BindBackbuffer(const ClearColor& clear_color = {}) override;
|
|
|
|
std::unique_ptr<AbstractShader> CreateShaderFromSource(ShaderStage stage, std::string_view source,
|
|
std::string_view name) override;
|
|
std::unique_ptr<AbstractShader> CreateShaderFromBinary(ShaderStage stage, const void* data,
|
|
size_t length,
|
|
std::string_view name) override;
|
|
std::unique_ptr<NativeVertexFormat>
|
|
CreateNativeVertexFormat(const PortableVertexDeclaration& vtx_decl) override;
|
|
std::unique_ptr<AbstractPipeline> CreatePipeline(const AbstractPipelineConfig& config,
|
|
const void* cache_data = nullptr,
|
|
size_t cache_data_length = 0) override;
|
|
|
|
void ShowImage(const AbstractTexture* source_texture,
|
|
const MathUtil::Rectangle<int>& source_rc) override;
|
|
|
|
void ScaleTexture(AbstractFramebuffer* dst_framebuffer, const MathUtil::Rectangle<int>& dst_rect,
|
|
const AbstractTexture* src_texture,
|
|
const MathUtil::Rectangle<int>& src_rect) override;
|
|
|
|
void SetScissorRect(const MathUtil::Rectangle<int>& rc) override;
|
|
|
|
void ClearRegion(const MathUtil::Rectangle<int>& target_rc, bool colorEnable, bool alphaEnable,
|
|
bool zEnable, u32 color, u32 z) override;
|
|
|
|
SurfaceInfo GetSurfaceInfo() const override;
|
|
|
|
private:
|
|
std::unique_ptr<SWOGLWindow> m_window;
|
|
};
|
|
|
|
} // namespace SW
|