mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-01-10 08:09:26 +01:00
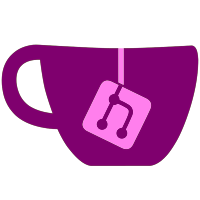
The default async api allow us to set some latency options. The old one (simple API) was the lazy way to go for usual audio where latency doesn't matter. This also streams audio, so it should be a bit faster then the old one.
64 lines
1.3 KiB
C++
64 lines
1.3 KiB
C++
// Copyright 2013 Dolphin Emulator Project
|
|
// Licensed under GPLv2
|
|
// Refer to the license.txt file included.
|
|
|
|
#ifndef _PULSE_AUDIO_STREAM_H
|
|
#define _PULSE_AUDIO_STREAM_H
|
|
|
|
#if defined(HAVE_PULSEAUDIO) && HAVE_PULSEAUDIO
|
|
#include <pulse/pulseaudio.h>
|
|
#endif
|
|
|
|
#include "Common.h"
|
|
#include "SoundStream.h"
|
|
|
|
#include "Thread.h"
|
|
|
|
class PulseAudio : public SoundStream
|
|
{
|
|
#if defined(HAVE_PULSEAUDIO) && HAVE_PULSEAUDIO
|
|
public:
|
|
PulseAudio(CMixer *mixer);
|
|
|
|
virtual bool Start();
|
|
virtual void Stop();
|
|
|
|
static bool isValid() {return true;}
|
|
|
|
virtual bool usesMixer() const {return true;}
|
|
|
|
virtual void Update();
|
|
|
|
void StateCallback(pa_context *c);
|
|
void WriteCallback(pa_stream *s, size_t length);
|
|
void UnderflowCallback(pa_stream *s);
|
|
|
|
private:
|
|
virtual void SoundLoop();
|
|
|
|
bool PulseInit();
|
|
void PulseShutdown();
|
|
|
|
// wrapper callback functions, last parameter _must_ be PulseAudio*
|
|
static void StateCallback(pa_context *c, void *userdata);
|
|
static void WriteCallback(pa_stream *s, size_t length, void *userdata);
|
|
static void UnderflowCallback(pa_stream *s, void *userdata);
|
|
|
|
std::thread m_thread;
|
|
bool m_run_thread;
|
|
|
|
int m_pa_error;
|
|
int m_pa_connected;
|
|
pa_mainloop *m_pa_ml;
|
|
pa_mainloop_api *m_pa_mlapi;
|
|
pa_context *m_pa_ctx;
|
|
pa_stream *m_pa_s;
|
|
pa_buffer_attr m_pa_ba;
|
|
#else
|
|
public:
|
|
PulseAudio(CMixer *mixer) : SoundStream(mixer) {}
|
|
#endif
|
|
};
|
|
|
|
#endif
|