mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-01-25 15:31:17 +01:00
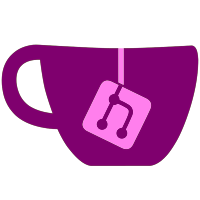
This class loads all the common PP shader configuration options and passes those options through to a inherited class that OpenGL or D3D will have. Makes it so all the common code for PP shaders is in VideoCommon instead of duplicating the code across each backend.
42 lines
848 B
C++
42 lines
848 B
C++
// Copyright 2013 Dolphin Emulator Project
|
|
// Licensed under GPLv2
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <string>
|
|
#include <unordered_map>
|
|
|
|
#include "VideoBackends/OGL/GLUtil.h"
|
|
#include "VideoCommon/VideoCommon.h"
|
|
|
|
namespace OGL
|
|
{
|
|
|
|
class OpenGLPostProcessing : public PostProcessingShaderImplementation
|
|
{
|
|
public:
|
|
OpenGLPostProcessing();
|
|
~OpenGLPostProcessing();
|
|
|
|
void BindTargetFramebuffer() override;
|
|
void BlitToScreen() override;
|
|
void Update(u32 width, u32 height) override;
|
|
void ApplyShader() override;
|
|
|
|
private:
|
|
GLuint m_fbo;
|
|
GLuint m_texture;
|
|
SHADER m_shader;
|
|
GLuint m_uniform_resolution;
|
|
GLuint m_uniform_time;
|
|
std::string m_glsl_header;
|
|
|
|
std::unordered_map<std::string, GLuint> m_uniform_bindings;
|
|
|
|
void CreateHeader();
|
|
std::string LoadShaderOptions(const std::string& code);
|
|
};
|
|
|
|
} // namespace
|