mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-02-07 21:23:31 +01:00
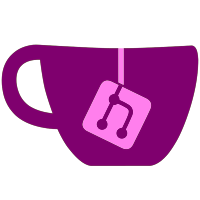
Replaces them with forward declarations of used types, or removes them entirely if they aren't used at all. This also replaces certain Common headers with less inclusive ones (in terms of definitions they pull in).
43 lines
751 B
C++
43 lines
751 B
C++
// Copyright 2013 Dolphin Emulator Project
|
|
// Licensed under GPLv2
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <string>
|
|
#include "InputCommon/InputConfig.h"
|
|
|
|
struct HotkeyStatus
|
|
{
|
|
u32 button[6];
|
|
s8 err;
|
|
};
|
|
|
|
class HotkeyManager : public ControllerEmu
|
|
{
|
|
public:
|
|
HotkeyManager();
|
|
~HotkeyManager();
|
|
|
|
std::string GetName() const;
|
|
void GetInput(HotkeyStatus* const hk);
|
|
void LoadDefaults(const ControllerInterface& ciface);
|
|
|
|
private:
|
|
Buttons* m_keys[3];
|
|
ControlGroup* m_options;
|
|
};
|
|
|
|
namespace HotkeyManagerEmu
|
|
{
|
|
void Initialize(void* const hwnd);
|
|
void Shutdown();
|
|
void LoadConfig();
|
|
|
|
InputConfig* GetConfig();
|
|
void GetStatus();
|
|
bool IsEnabled();
|
|
void Enable(bool enable_toggle);
|
|
bool IsPressed(int Id, bool held);
|
|
}
|