mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-03-06 01:37:49 +01:00
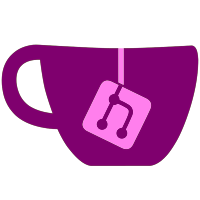
While setting up a proper NAND for Wii emulation has become much easier now that disc and online system updates work, they still require users to have a recent disc game, certificates extracted from IOS or a NAND dump for online updates to work and to really get all system titles. This commit adds the ability to do an online update right from Dolphin itself, which solves that usability issue.
41 lines
1.1 KiB
C++
41 lines
1.1 KiB
C++
// Copyright 2017 Dolphin Emulator Project
|
|
// Licensed under GPLv2+
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <cstddef>
|
|
#include <functional>
|
|
#include <string>
|
|
|
|
#include "Common/CommonTypes.h"
|
|
|
|
// Small utility functions for common Wii related tasks.
|
|
|
|
namespace WiiUtils
|
|
{
|
|
bool InstallWAD(const std::string& wad_path);
|
|
|
|
enum class UpdateResult
|
|
{
|
|
Succeeded,
|
|
AlreadyUpToDate,
|
|
|
|
// NUS errors and failures.
|
|
ServerFailed,
|
|
// General download failures.
|
|
DownloadFailed,
|
|
// Import failures.
|
|
ImportFailed,
|
|
// Update was cancelled.
|
|
Cancelled,
|
|
};
|
|
|
|
// Return false to cancel the update as soon as the current title has finished updating.
|
|
using UpdateCallback = std::function<bool(size_t processed, size_t total, u64 title_id)>;
|
|
// Download and install the latest version of all titles (if missing) from NUS.
|
|
// If no region is specified, the region of the installed System Menu will be used.
|
|
// If no region is specified and no system menu is installed, the update will fail.
|
|
UpdateResult DoOnlineUpdate(UpdateCallback update_callback, const std::string& region);
|
|
}
|