mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-02-11 15:09:00 +01:00
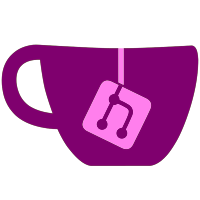
When rendering to the main window, the wxGLCanvas should really be owned by the DolphinWX code for it to be safely freed. Hack around the problem by just hiding the canvas for now. git-svn-id: https://dolphin-emu.googlecode.com/svn/trunk@6890 8ced0084-cf51-0410-be5f-012b33b47a6e
136 lines
3.5 KiB
Python
136 lines
3.5 KiB
Python
# -*- python -*-
|
|
|
|
Import('env')
|
|
import os
|
|
import sys
|
|
from SconsTests import utils
|
|
|
|
files = [
|
|
'BootManager.cpp',
|
|
]
|
|
|
|
libs = [
|
|
'core', 'lzo2', 'discio', 'bdisasm',
|
|
'inputcommon', 'common', 'lua', 'z', 'sfml-network',
|
|
]
|
|
|
|
wxlibs = [ ]
|
|
|
|
ldflags = [ ]
|
|
|
|
if env['HAVE_WX']:
|
|
files += [
|
|
'AboutDolphin.cpp',
|
|
'ARCodeAddEdit.cpp',
|
|
'GeckoCodeDiag.cpp',
|
|
'ConfigMain.cpp',
|
|
'Frame.cpp',
|
|
'FrameAui.cpp',
|
|
'FrameTools.cpp',
|
|
'LuaWindow.cpp',
|
|
'LogWindow.cpp',
|
|
'GameListCtrl.cpp',
|
|
'HotkeyDlg.cpp',
|
|
'InputConfigDiag.cpp',
|
|
'InputConfigDiagBitmaps.cpp',
|
|
'ISOFile.cpp',
|
|
'ISOProperties.cpp',
|
|
'PatchAddEdit.cpp',
|
|
'CheatsWindow.cpp',
|
|
'Main.cpp',
|
|
'MemcardManager.cpp',
|
|
'MemoryCards/GCMemcard.cpp',
|
|
'MemoryCards/WiiSaveCrypted.cpp',
|
|
'NetPlay.cpp',
|
|
'NetPlayClient.cpp',
|
|
'NetPlayServer.cpp',
|
|
'NetWindow.cpp',
|
|
'UDPConfigDiag.cpp',
|
|
'WiimoteConfigDiag.cpp',
|
|
'WXInputBase.cpp',
|
|
'WxUtils.cpp',
|
|
]
|
|
|
|
wxlibs += [ 'debwx', 'debugger_ui_util' ]
|
|
else:
|
|
files += [
|
|
'MainNoGUI.cpp',
|
|
]
|
|
|
|
if sys.platform == 'win32':
|
|
files += [ "stdafx.cpp" ]
|
|
elif sys.platform == 'darwin':
|
|
ldflags += [ '-Wl,-pagezero_size,0x1000' ]
|
|
libs += [ 'iconv' ]
|
|
exe = '#' + env['prefix'] + '/Dolphin.app/Contents/MacOS/Dolphin'
|
|
|
|
if env['HAVE_WX']:
|
|
wxlibs += env['wxconfiglibs']
|
|
else:
|
|
exe += 'NoGUI'
|
|
|
|
env.Install('#' + env['prefix'] + '/Dolphin.app/Contents/' +
|
|
'Library/Frameworks/Cg.framework', source = [
|
|
'#Externals/Cg/Cg.framework/Cg',
|
|
'#Externals/Cg/Cg.framework/Resources'
|
|
])
|
|
|
|
env.Install(env['data_dir'], '#Data/Sys')
|
|
env.Install(env['data_dir'], '#Data/User')
|
|
env.Install(env['data_dir'],
|
|
'#Source/Core/DolphinWX/resources/Dolphin.icns')
|
|
|
|
msgfmt = env.WhereIs('msgfmt')
|
|
if not msgfmt == None:
|
|
po_files = Glob('#Languages/*.po', strings = True)
|
|
for po in po_files:
|
|
index_lo = po.find('Languages/') + len('Languages/')
|
|
index_hi = po.find('.po')
|
|
lproj = os.sep + po[index_lo:index_hi] + '.lproj'
|
|
mo_dir = env['build_dir'] + '/Languages' + lproj
|
|
mo_file = mo_dir + '/dolphin-emu.mo'
|
|
env.Command('#' + mo_file, po, 'mkdir -p ' + mo_dir +
|
|
' && ' + msgfmt + ' -o ' + mo_file + ' ' + po)
|
|
env.Install(env['data_dir'] + lproj, '#' + mo_file)
|
|
|
|
from plistlib import writePlist
|
|
def createPlist(target, source, env):
|
|
for srcNode in source:
|
|
writePlist(srcNode.value, str(target[0]))
|
|
env.Append(BUILDERS = {'Plist' : Builder(action = createPlist)})
|
|
env.Plist('#' + env['prefix'] + '/Dolphin.app/Contents/Info.plist',
|
|
Value(dict(
|
|
CFBundleExecutable = 'Dolphin',
|
|
CFBundleIconFile = 'Dolphin.icns',
|
|
CFBundleIdentifier = 'com.dolphin-emu.dolphin',
|
|
CFBundlePackageType = 'APPL',
|
|
CFBundleShortVersionString =
|
|
utils.GenerateRevFile('', Dir('#None').abspath, None),
|
|
CFBundleVersion = '3.0',
|
|
LSMinimumSystemVersion = '10.5.4',
|
|
LSRequiresCarbon = True,
|
|
CFBundleDocumentTypes = [
|
|
dict(CFBundleTypeExtensions =
|
|
('ciso', 'dol', 'elf', 'gcm', 'gcz', 'iso', 'wad'),
|
|
CFBundleTypeIconFile = 'Dolphin.icns',
|
|
CFBundleTypeName = 'Nintendo GC/Wii file',
|
|
CFBundleTypeRole = 'Viewer')]
|
|
)))
|
|
|
|
env.Command('dummy', '#' + env['prefix'],
|
|
"find $SOURCES -name .svn -exec rm -rf {} +")
|
|
else:
|
|
files += [ 'X11Utils.cpp' ]
|
|
libs += [ 'SDL' ]
|
|
if env['HAVE_WX']:
|
|
exe = env['binary_dir'] + '/dolphin-emu'
|
|
else:
|
|
exe = env['binary_dir'] + '/dolphin-emu-nogui'
|
|
env.InstallAs(env['data_dir'] + '/sys', '#Data/Sys')
|
|
env.InstallAs(env['data_dir'] + '/user', '#Data/User')
|
|
|
|
libs = wxlibs + libs + env['LIBS']
|
|
linkflags = ldflags + env['LINKFLAGS']
|
|
|
|
env.Program(exe, files, LIBS = libs, LINKFLAGS = linkflags)
|