mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-01-11 16:49:12 +01:00
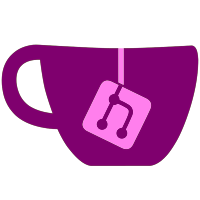
the intent is to replace the haphazard scheduling and finger-crossing associated with saving/loading with the correct and minimal necessary wait for each thread to reach a known safe location before commencing the savestate operation, and for any already-paused components to not need to be resumed to do so.
58 lines
1.2 KiB
C++
58 lines
1.2 KiB
C++
|
|
#ifndef SW_VIDEO_BACKEND_H_
|
|
#define SW_VIDEO_BACKEND_H_
|
|
|
|
#include "VideoBackendBase.h"
|
|
|
|
namespace SW
|
|
{
|
|
|
|
class VideoSoftware : public VideoBackend
|
|
{
|
|
bool Initialize(void *&);
|
|
void Shutdown();
|
|
|
|
std::string GetName();
|
|
|
|
void EmuStateChange(EMUSTATE_CHANGE newState);
|
|
|
|
void RunLoop(bool enable);
|
|
|
|
void ShowConfig(void* parent);
|
|
|
|
void Video_Prepare();
|
|
|
|
void Video_EnterLoop();
|
|
void Video_ExitLoop();
|
|
void Video_BeginField(u32, FieldType, u32, u32);
|
|
void Video_EndField();
|
|
u32 Video_AccessEFB(EFBAccessType, u32, u32, u32);
|
|
|
|
void Video_AddMessage(const char* pstr, unsigned int milliseconds);
|
|
void Video_ClearMessages();
|
|
bool Video_Screenshot(const char* filename);
|
|
|
|
void Video_SetRendering(bool bEnabled);
|
|
|
|
void Video_GatherPipeBursted();
|
|
bool Video_IsHiWatermarkActive();
|
|
bool Video_IsPossibleWaitingSetDrawDone();
|
|
void Video_AbortFrame();
|
|
|
|
readFn16 Video_CPRead16();
|
|
writeFn16 Video_CPWrite16();
|
|
readFn16 Video_PERead16();
|
|
writeFn16 Video_PEWrite16();
|
|
writeFn32 Video_PEWrite32();
|
|
|
|
void UpdateFPSDisplay(const char*);
|
|
unsigned int PeekMessages();
|
|
|
|
void PauseAndLock(bool doLock, bool unpauseOnUnlock=true);
|
|
void DoState(PointerWrap &p);
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|