mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-02-28 23:13:42 +01:00
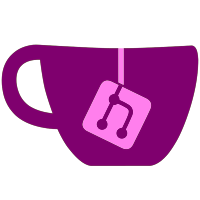
long become wxWidgets 2.9.2, which in turn is expected to be the last 2.9 release before the 3.0 stable release. Since the full wxWidgets distribution is rather large, I have imported only the parts that we use, on a subdirectory basis: art include/wx/*.* include/wx/aui include/wx/cocoa include/wx/generic include/wx/gtk include/wx/meta include/wx/msw include/wx/osx include/wx/persist include/wx/private include/wx/protocol include/wx/unix src/aui src/common src/generic src/gtk src/msw src/osx src/unix git-svn-id: https://dolphin-emu.googlecode.com/svn/trunk@7380 8ced0084-cf51-0410-be5f-012b33b47a6e
118 lines
3.3 KiB
C++
118 lines
3.3 KiB
C++
/////////////////////////////////////////////////////////////////////////////
|
|
// Name: src/common/sysopt.cpp
|
|
// Purpose: wxSystemOptions
|
|
// Author: Julian Smart
|
|
// Modified by:
|
|
// Created: 2001-07-10
|
|
// RCS-ID: $Id: sysopt.cpp 64651 2010-06-20 17:43:15Z VZ $
|
|
// Copyright: (c) Julian Smart
|
|
// Licence: wxWindows licence
|
|
/////////////////////////////////////////////////////////////////////////////
|
|
|
|
// ============================================================================
|
|
// declarations
|
|
// ============================================================================
|
|
|
|
// ---------------------------------------------------------------------------
|
|
// headers
|
|
// ---------------------------------------------------------------------------
|
|
|
|
// For compilers that support precompilation, includes "wx.h".
|
|
#include "wx/wxprec.h"
|
|
|
|
#if defined(__BORLANDC__)
|
|
#pragma hdrstop
|
|
#endif
|
|
|
|
#if wxUSE_SYSTEM_OPTIONS
|
|
|
|
#include "wx/sysopt.h"
|
|
|
|
#ifndef WX_PRECOMP
|
|
#include "wx/app.h"
|
|
#include "wx/list.h"
|
|
#include "wx/string.h"
|
|
#include "wx/arrstr.h"
|
|
#endif
|
|
|
|
// ----------------------------------------------------------------------------
|
|
// private globals
|
|
// ----------------------------------------------------------------------------
|
|
|
|
static wxArrayString gs_optionNames,
|
|
gs_optionValues;
|
|
|
|
// ============================================================================
|
|
// wxSystemOptions implementation
|
|
// ============================================================================
|
|
|
|
// Option functions (arbitrary name/value mapping)
|
|
void wxSystemOptions::SetOption(const wxString& name, const wxString& value)
|
|
{
|
|
int idx = gs_optionNames.Index(name, false);
|
|
if (idx == wxNOT_FOUND)
|
|
{
|
|
gs_optionNames.Add(name);
|
|
gs_optionValues.Add(value);
|
|
}
|
|
else
|
|
{
|
|
gs_optionNames[idx] = name;
|
|
gs_optionValues[idx] = value;
|
|
}
|
|
}
|
|
|
|
void wxSystemOptions::SetOption(const wxString& name, int value)
|
|
{
|
|
SetOption(name, wxString::Format(wxT("%d"), value));
|
|
}
|
|
|
|
wxString wxSystemOptions::GetOption(const wxString& name)
|
|
{
|
|
wxString val;
|
|
|
|
int idx = gs_optionNames.Index(name, false);
|
|
if ( idx != wxNOT_FOUND )
|
|
{
|
|
val = gs_optionValues[idx];
|
|
}
|
|
else // not set explicitely
|
|
{
|
|
// look in the environment: first for a variable named "wx_appname_name"
|
|
// which can be set to affect the behaviour or just this application
|
|
// and then for "wx_name" which can be set to change the option globally
|
|
wxString var(name);
|
|
var.Replace(wxT("."), wxT("_")); // '.'s not allowed in env var names
|
|
var.Replace(wxT("-"), wxT("_")); // and neither are '-'s
|
|
|
|
wxString appname;
|
|
if ( wxTheApp )
|
|
appname = wxTheApp->GetAppName();
|
|
|
|
if ( !appname.empty() )
|
|
val = wxGetenv(wxT("wx_") + appname + wxT('_') + var);
|
|
|
|
if ( val.empty() )
|
|
val = wxGetenv(wxT("wx_") + var);
|
|
}
|
|
|
|
return val;
|
|
}
|
|
|
|
int wxSystemOptions::GetOptionInt(const wxString& name)
|
|
{
|
|
#ifdef _PACC_VER
|
|
// work around the PalmOS pacc compiler bug
|
|
return wxAtoi (GetOption(name).data());
|
|
#else
|
|
return wxAtoi (GetOption(name));
|
|
#endif
|
|
}
|
|
|
|
bool wxSystemOptions::HasOption(const wxString& name)
|
|
{
|
|
return !GetOption(name).empty();
|
|
}
|
|
|
|
#endif // wxUSE_SYSTEM_OPTIONS
|