mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-01-13 09:39:11 +01:00
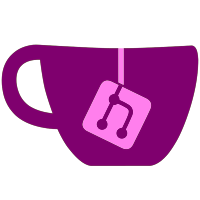
From wxWidgets master 81570ae070b35c9d52de47b1f14897f3ff1a66c7. include/wx/defs.h -- __w64 warning disable patch by comex brought forward. include/wx/msw/window.h -- added GetContentScaleFactor() which was not implemented on Windows but is necessary for wxBitmap scaling on Mac OS X so it needs to work to avoid #ifdef-ing the code. src/gtk/window.cpp -- Modified DoSetClientSize() to direct call wxWindowGTK::DoSetSize() instead of using public wxWindowBase::SetSize() which now prevents derived classes (like wxAuiToolbar) intercepting the call and breaking it. This matches Windows which does NOT need to call DoSetSize internally. End result is this fixes Dolphin's debug tools toolbars on Linux. src/osx/window_osx.cpp -- Same fix as for GTK since it has the same issue. src/msw/radiobox.cpp -- Hacked to fix display in HiDPI (was clipping off end of text). Updated CMakeLists for Linux and Mac OS X. Small code changes to Dolphin to fix debug error boxes, deprecation warnings, and retain previous UI behavior on Windows.
153 lines
3.8 KiB
C++
153 lines
3.8 KiB
C++
///////////////////////////////////////////////////////////////////////////////
|
|
// Name: wx/cmdargs.h
|
|
// Purpose: declaration of wxCmdLineArgsArray helper class
|
|
// Author: Vadim Zeitlin
|
|
// Created: 2007-11-12
|
|
// Copyright: (c) 2007 Vadim Zeitlin <vadim@wxwindows.org>
|
|
// Licence: wxWindows licence
|
|
///////////////////////////////////////////////////////////////////////////////
|
|
|
|
#ifndef _WX_CMDARGS_H_
|
|
#define _WX_CMDARGS_H_
|
|
|
|
#include "wx/arrstr.h"
|
|
|
|
// ----------------------------------------------------------------------------
|
|
// wxCmdLineArgsArray: helper class used by wxApp::argv
|
|
// ----------------------------------------------------------------------------
|
|
|
|
#if wxUSE_UNICODE
|
|
|
|
// this class is used instead of either "char **" or "wchar_t **" (neither of
|
|
// which would be backwards compatible with all the existing code) for argv
|
|
// field of wxApp
|
|
//
|
|
// as it's used for compatibility, it tries to look as much as traditional
|
|
// (char **) argv as possible, in particular it provides implicit conversions
|
|
// to "char **" and also array-like operator[]
|
|
class WXDLLIMPEXP_BASE wxCmdLineArgsArray
|
|
{
|
|
public:
|
|
wxCmdLineArgsArray() { m_argsA = NULL; m_argsW = NULL; }
|
|
|
|
template <typename T>
|
|
wxCmdLineArgsArray& operator=(T **argv)
|
|
{
|
|
FreeArgs();
|
|
|
|
m_args.clear();
|
|
|
|
if ( argv )
|
|
{
|
|
while ( *argv )
|
|
m_args.push_back(*argv++);
|
|
}
|
|
|
|
return *this;
|
|
}
|
|
|
|
operator char**() const
|
|
{
|
|
if ( !m_argsA )
|
|
{
|
|
const size_t count = m_args.size();
|
|
m_argsA = new char *[count];
|
|
for ( size_t n = 0; n < count; n++ )
|
|
m_argsA[n] = wxStrdup(m_args[n].ToAscii());
|
|
}
|
|
|
|
return m_argsA;
|
|
}
|
|
|
|
operator wchar_t**() const
|
|
{
|
|
if ( !m_argsW )
|
|
{
|
|
const size_t count = m_args.size();
|
|
m_argsW = new wchar_t *[count];
|
|
for ( size_t n = 0; n < count; n++ )
|
|
m_argsW[n] = wxStrdup(m_args[n].wc_str());
|
|
}
|
|
|
|
return m_argsW;
|
|
}
|
|
|
|
// existing code does checks like "if ( argv )" and we want it to continue
|
|
// to compile, so provide this conversion even if it is pretty dangerous
|
|
operator bool() const
|
|
{
|
|
return !m_args.empty();
|
|
}
|
|
|
|
// and the same for "if ( !argv )" checks
|
|
bool operator!() const
|
|
{
|
|
return m_args.empty();
|
|
}
|
|
|
|
wxString operator[](size_t n) const
|
|
{
|
|
return m_args[n];
|
|
}
|
|
|
|
// we must provide this overload for g++ 3.4 which can't choose between
|
|
// our operator[](size_t) and ::operator[](char**, int) otherwise
|
|
wxString operator[](int n) const
|
|
{
|
|
return m_args[n];
|
|
}
|
|
|
|
|
|
// convenience methods, i.e. not existing only for backwards compatibility
|
|
|
|
// do we have any arguments at all?
|
|
bool IsEmpty() const { return m_args.empty(); }
|
|
|
|
// access the arguments as a convenient array of wxStrings
|
|
const wxArrayString& GetArguments() const { return m_args; }
|
|
|
|
~wxCmdLineArgsArray()
|
|
{
|
|
FreeArgs();
|
|
}
|
|
|
|
private:
|
|
template <typename T>
|
|
void Free(T**& args)
|
|
{
|
|
if ( !args )
|
|
return;
|
|
|
|
const size_t count = m_args.size();
|
|
for ( size_t n = 0; n < count; n++ )
|
|
free(args[n]);
|
|
|
|
delete [] args;
|
|
args = NULL;
|
|
}
|
|
|
|
void FreeArgs()
|
|
{
|
|
Free(m_argsA);
|
|
Free(m_argsW);
|
|
}
|
|
|
|
wxArrayString m_args;
|
|
mutable char **m_argsA;
|
|
mutable wchar_t **m_argsW;
|
|
|
|
wxDECLARE_NO_COPY_CLASS(wxCmdLineArgsArray);
|
|
};
|
|
|
|
// provide global operator overload for compatibility with the existing code
|
|
// doing things like "if ( condition && argv )"
|
|
inline bool operator&&(bool cond, const wxCmdLineArgsArray& array)
|
|
{
|
|
return cond && !array.IsEmpty();
|
|
}
|
|
|
|
#endif // wxUSE_UNICODE
|
|
|
|
#endif // _WX_CMDARGS_H_
|
|
|