mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-01-15 18:49:11 +01:00
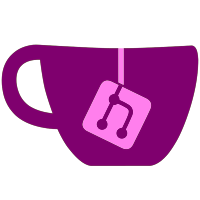
Instead of comparing the game ID, revision, disc number and name, we can compare a hash of important parts of the disc including all the aforementioned data but also additional data such as the FST. The primary reason why I'm making this change is to let us catch more desyncs before they happen, but this should also fix https://bugs.dolphin-emu.org/issues/12115. As a bonus, the UI can now distinguish the case where a client doesn't have the game at all from the case where a client has the wrong version of the game.
84 lines
1.7 KiB
C++
84 lines
1.7 KiB
C++
// Copyright 2017 Dolphin Emulator Project
|
|
// Licensed under GPLv2+
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <QDialog>
|
|
|
|
class GameListModel;
|
|
class QCheckBox;
|
|
class QComboBox;
|
|
class QDialogButtonBox;
|
|
class QLabel;
|
|
class QLineEdit;
|
|
class QListWidget;
|
|
class QGridLayout;
|
|
class QPushButton;
|
|
class QSpinBox;
|
|
class QTabWidget;
|
|
|
|
namespace UICommon
|
|
{
|
|
class GameFile;
|
|
}
|
|
|
|
class NetPlaySetupDialog : public QDialog
|
|
{
|
|
Q_OBJECT
|
|
public:
|
|
explicit NetPlaySetupDialog(QWidget* parent);
|
|
|
|
void accept() override;
|
|
void show();
|
|
|
|
signals:
|
|
bool Join();
|
|
bool Host(const UICommon::GameFile& game);
|
|
|
|
private:
|
|
void CreateMainLayout();
|
|
void ConnectWidgets();
|
|
void PopulateGameList();
|
|
void ResetTraversalHost();
|
|
|
|
void SaveSettings();
|
|
|
|
void OnConnectionTypeChanged(int index);
|
|
|
|
// Main Widget
|
|
QDialogButtonBox* m_button_box;
|
|
QComboBox* m_connection_type;
|
|
QLineEdit* m_nickname_edit;
|
|
QGridLayout* m_main_layout;
|
|
QTabWidget* m_tab_widget;
|
|
QPushButton* m_reset_traversal_button;
|
|
|
|
// Connection Widget
|
|
QLabel* m_ip_label;
|
|
QLineEdit* m_ip_edit;
|
|
QLabel* m_connect_port_label;
|
|
QSpinBox* m_connect_port_box;
|
|
QPushButton* m_connect_button;
|
|
|
|
// Host Widget
|
|
QLabel* m_host_port_label;
|
|
QSpinBox* m_host_port_box;
|
|
QListWidget* m_host_games;
|
|
QPushButton* m_host_button;
|
|
QCheckBox* m_host_force_port_check;
|
|
QSpinBox* m_host_force_port_box;
|
|
QCheckBox* m_host_chunked_upload_limit_check;
|
|
QSpinBox* m_host_chunked_upload_limit_box;
|
|
QCheckBox* m_host_server_browser;
|
|
QLineEdit* m_host_server_name;
|
|
QLineEdit* m_host_server_password;
|
|
QComboBox* m_host_server_region;
|
|
|
|
#ifdef USE_UPNP
|
|
QCheckBox* m_host_upnp;
|
|
#endif
|
|
|
|
GameListModel* m_game_list_model;
|
|
};
|