mirror of
https://github.com/Barubary/dsdecmp.git
synced 2024-11-16 23:59:25 +01:00
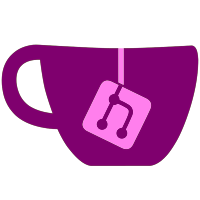
To keep the base program mobile, all native NDS formats do not require plugins. The 8-bit Huffman format cannot be used to compress at the moment; thanks to CUE a bug has been found where the offset-field of a node overflows, corrupting the compressed file.
59 lines
1.4 KiB
C#
59 lines
1.4 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
|
|
namespace DSDecmp.Formats.Nitro
|
|
{
|
|
public class CompositeGBAFormat : CompositeFormat
|
|
{
|
|
public CompositeGBAFormat()
|
|
: base(new Huffman4(), new Huffman8(), new LZ10()) { }
|
|
|
|
public override string ShortFormatString
|
|
{
|
|
get { return "GBA"; }
|
|
}
|
|
|
|
public override string Description
|
|
{
|
|
get { return "All formats natively supported by the GBA."; }
|
|
}
|
|
|
|
public override bool SupportsCompression
|
|
{
|
|
get { return true; }
|
|
}
|
|
|
|
public override string CompressionFlag
|
|
{
|
|
get { return "gba*"; }
|
|
}
|
|
}
|
|
|
|
public class CompositeNDSFormat : CompositeFormat
|
|
{
|
|
public CompositeNDSFormat()
|
|
: base(new Huffman4(), new Huffman8(), new LZ10(), new LZ11()) { }
|
|
|
|
public override string ShortFormatString
|
|
{
|
|
get { return "NDS"; }
|
|
}
|
|
|
|
public override string Description
|
|
{
|
|
get { return "All formats natively supported by the NDS."; }
|
|
}
|
|
|
|
public override bool SupportsCompression
|
|
{
|
|
get { return true; }
|
|
}
|
|
|
|
public override string CompressionFlag
|
|
{
|
|
get { return "nds*"; }
|
|
}
|
|
}
|
|
}
|