mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-06-02 01:48:45 +02:00
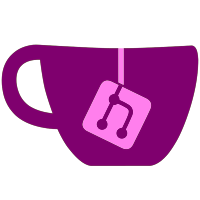
This commit contains the C++ side of the initial Input implementation, this is based on the work done in the `hid` branch in `bylaws/skyline`. Co-authored-by: ◱ PixelyIon <pixelyion@protonmail.com>
59 lines
2.6 KiB
C++
59 lines
2.6 KiB
C++
// SPDX-License-Identifier: MPL-2.0
|
|
// Copyright © 2020 Skyline Team and Contributors (https://github.com/skyline-emu/)
|
|
|
|
#pragma once
|
|
|
|
#include <services/base_service.h>
|
|
#include <services/serviceman.h>
|
|
#include "IAppletResource.h"
|
|
|
|
namespace skyline::service::hid {
|
|
/**
|
|
* @brief IHidServer or hid service is used to access input devices (https://switchbrew.org/wiki/HID_services#hid)
|
|
*/
|
|
class IHidServer : public BaseService {
|
|
public:
|
|
IHidServer(const DeviceState &state, ServiceManager &manager);
|
|
|
|
/**
|
|
* @brief This returns an IAppletResource (https://switchbrew.org/wiki/HID_services#CreateAppletResource)
|
|
*/
|
|
void CreateAppletResource(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response);
|
|
|
|
/**
|
|
* @brief This sets the style of controllers supported (https://switchbrew.org/wiki/HID_services#SetSupportedNpadStyleSet)
|
|
*/
|
|
void SetSupportedNpadStyleSet(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response);
|
|
|
|
/**
|
|
* @brief This sets the NpadIds which are supported (https://switchbrew.org/wiki/HID_services#SetSupportedNpadIdType)
|
|
*/
|
|
void SetSupportedNpadIdType(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response);
|
|
|
|
/**
|
|
* @brief This requests the activation of a controller. This is stubbed as we don't have to activate anything.
|
|
*/
|
|
void ActivateNpad(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response);
|
|
|
|
/**
|
|
* @brief Sets the Joy-Con hold mode (https://switchbrew.org/wiki/HID_services#SetNpadJoyHoldType)
|
|
*/
|
|
void SetNpadJoyHoldType(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response);
|
|
|
|
/**
|
|
* @brief Sets the Joy-Con assignment mode to Single by default (https://switchbrew.org/wiki/HID_services#SetNpadJoyAssignmentModeSingleByDefault)
|
|
*/
|
|
void SetNpadJoyAssignmentModeSingleByDefault(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response);
|
|
|
|
/**
|
|
* @brief Sets the Joy-Con assignment mode to Single (https://switchbrew.org/wiki/HID_services#SetNpadJoyAssignmentModeSingle)
|
|
*/
|
|
void SetNpadJoyAssignmentModeSingle(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response);
|
|
|
|
/**
|
|
* @brief Sets the Joy-Con assignment mode to Dual (https://switchbrew.org/wiki/HID_services#SetNpadJoyAssignmentModeDual)
|
|
*/
|
|
void SetNpadJoyAssignmentModeDual(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response);
|
|
};
|
|
}
|