mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-06-02 02:58:46 +02:00
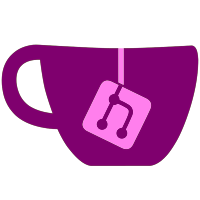
This commit adds Vulkan-Hpp as a library to the project. The headers are from a modified version of `VulkanHppGenerator`. They are broken into multiple files to avoid exceeding the Intellisense file size limit of Android Studio.
779 lines
22 KiB
C++
779 lines
22 KiB
C++
// Copyright (c) 2015-2019 The Khronos Group Inc.
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
//
|
|
// ---- Exceptions to the Apache 2.0 License: ----
|
|
//
|
|
// As an exception, if you use this Software to generate code and portions of
|
|
// this Software are embedded into the generated code as a result, you may
|
|
// redistribute such product without providing attribution as would otherwise
|
|
// be required by Sections 4(a), 4(b) and 4(d) of the License.
|
|
//
|
|
// In addition, if you combine or link code generated by this Software with
|
|
// software that is licensed under the GPLv2 or the LGPL v2.0 or 2.1
|
|
// ("`Combined Software`") and if a court of competent jurisdiction determines
|
|
// that the patent provision (Section 3), the indemnity provision (Section 9)
|
|
// or other Section of the License conflicts with the conditions of the
|
|
// applicable GPL or LGPL license, you may retroactively and prospectively
|
|
// choose to deem waived or otherwise exclude such Section(s) of the License,
|
|
// but only in their entirety and only with respect to the Combined Software.
|
|
//
|
|
|
|
// This header is generated from the Khronos Vulkan XML API Registry.
|
|
|
|
#pragma once
|
|
|
|
#include <algorithm>
|
|
#include <array>
|
|
#include <cstddef>
|
|
#include <cstdint>
|
|
#include <cstring>
|
|
#include <initializer_list>
|
|
#include <string>
|
|
#include <system_error>
|
|
#include <tuple>
|
|
#include <type_traits>
|
|
#include <vulkan/vulkan.h>
|
|
|
|
#if defined(VULKAN_HPP_DISABLE_ENHANCED_MODE)
|
|
# if !defined(VULKAN_HPP_NO_SMART_HANDLE)
|
|
# define VULKAN_HPP_NO_SMART_HANDLE
|
|
# endif
|
|
#else
|
|
# include <memory>
|
|
# include <vector>
|
|
#endif
|
|
|
|
#if !defined(VULKAN_HPP_ASSERT)
|
|
# include <cassert>
|
|
# define VULKAN_HPP_ASSERT assert
|
|
#endif
|
|
|
|
#if !defined(VULKAN_HPP_ENABLE_DYNAMIC_LOADER_TOOL)
|
|
# define VULKAN_HPP_ENABLE_DYNAMIC_LOADER_TOOL 1
|
|
#endif
|
|
|
|
#if VULKAN_HPP_ENABLE_DYNAMIC_LOADER_TOOL == 1
|
|
# if defined(__linux__) || defined(__APPLE__)
|
|
# include <dlfcn.h>
|
|
# endif
|
|
|
|
# if defined(_WIN32)
|
|
# include <windows.h>
|
|
# endif
|
|
#endif
|
|
|
|
#if 201711 <= __cpp_impl_three_way_comparison
|
|
# define VULKAN_HPP_HAS_SPACESHIP_OPERATOR
|
|
#endif
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
# include <compare>
|
|
#endif
|
|
|
|
static_assert( VK_HEADER_VERSION == 121 , "Wrong VK_HEADER_VERSION!" );
|
|
|
|
// 32-bit vulkan is not typesafe for handles, so don't allow copy constructors on this platform by default.
|
|
// To enable this feature on 32-bit platforms please define VULKAN_HPP_TYPESAFE_CONVERSION
|
|
#if defined(__LP64__) || defined(_WIN64) || (defined(__x86_64__) && !defined(__ILP32__) ) || defined(_M_X64) || defined(__ia64) || defined (_M_IA64) || defined(__aarch64__) || defined(__powerpc64__)
|
|
# if !defined( VULKAN_HPP_TYPESAFE_CONVERSION )
|
|
# define VULKAN_HPP_TYPESAFE_CONVERSION
|
|
# endif
|
|
#endif
|
|
|
|
// <tuple> includes <sys/sysmacros.h> through some other header
|
|
// this results in major(x) being resolved to gnu_dev_major(x)
|
|
// which is an expression in a constructor initializer list.
|
|
#if defined(major)
|
|
#undef major
|
|
#endif
|
|
#if defined(minor)
|
|
#undef minor
|
|
#endif
|
|
|
|
// Windows defines MemoryBarrier which is deprecated and collides
|
|
// with the VULKAN_HPP_NAMESPACE::MemoryBarrier struct.
|
|
#if defined(MemoryBarrier)
|
|
#undef MemoryBarrier
|
|
#endif
|
|
|
|
#if !defined(VULKAN_HPP_HAS_UNRESTRICTED_UNIONS)
|
|
# if defined(__clang__)
|
|
# if __has_feature(cxx_unrestricted_unions)
|
|
# define VULKAN_HPP_HAS_UNRESTRICTED_UNIONS
|
|
# endif
|
|
# elif defined(__GNUC__)
|
|
# define GCC_VERSION (__GNUC__ * 10000 + __GNUC_MINOR__ * 100 + __GNUC_PATCHLEVEL__)
|
|
# if 40600 <= GCC_VERSION
|
|
# define VULKAN_HPP_HAS_UNRESTRICTED_UNIONS
|
|
# endif
|
|
# elif defined(_MSC_VER)
|
|
# if 1900 <= _MSC_VER
|
|
# define VULKAN_HPP_HAS_UNRESTRICTED_UNIONS
|
|
# endif
|
|
# endif
|
|
#endif
|
|
|
|
#if !defined(VULKAN_HPP_INLINE)
|
|
# if defined(__clang__)
|
|
# if __has_attribute(always_inline)
|
|
# define VULKAN_HPP_INLINE __attribute__((always_inline)) __inline__
|
|
# else
|
|
# define VULKAN_HPP_INLINE inline
|
|
# endif
|
|
# elif defined(__GNUC__)
|
|
# define VULKAN_HPP_INLINE __attribute__((always_inline)) __inline__
|
|
# elif defined(_MSC_VER)
|
|
# define VULKAN_HPP_INLINE inline
|
|
# else
|
|
# define VULKAN_HPP_INLINE inline
|
|
# endif
|
|
#endif
|
|
|
|
#if defined(VULKAN_HPP_TYPESAFE_CONVERSION)
|
|
# define VULKAN_HPP_TYPESAFE_EXPLICIT
|
|
#else
|
|
# define VULKAN_HPP_TYPESAFE_EXPLICIT explicit
|
|
#endif
|
|
|
|
#if defined(__cpp_constexpr)
|
|
# define VULKAN_HPP_CONSTEXPR constexpr
|
|
# if __cpp_constexpr >= 201304
|
|
# define VULKAN_HPP_CONSTEXPR_14 constexpr
|
|
# else
|
|
# define VULKAN_HPP_CONSTEXPR_14
|
|
# endif
|
|
# define VULKAN_HPP_CONST_OR_CONSTEXPR constexpr
|
|
#else
|
|
# define VULKAN_HPP_CONSTEXPR
|
|
# define VULKAN_HPP_CONSTEXPR_14
|
|
# define VULKAN_HPP_CONST_OR_CONSTEXPR const
|
|
#endif
|
|
|
|
#if !defined(VULKAN_HPP_NOEXCEPT)
|
|
# if defined(_MSC_VER) && (_MSC_VER <= 1800)
|
|
# define VULKAN_HPP_NOEXCEPT
|
|
# else
|
|
# define VULKAN_HPP_NOEXCEPT noexcept
|
|
# define VULKAN_HPP_HAS_NOEXCEPT 1
|
|
# endif
|
|
#endif
|
|
|
|
#if !defined(VULKAN_HPP_NAMESPACE)
|
|
#define VULKAN_HPP_NAMESPACE vk
|
|
#endif
|
|
|
|
#define VULKAN_HPP_STRINGIFY2(text) #text
|
|
#define VULKAN_HPP_STRINGIFY(text) VULKAN_HPP_STRINGIFY2(text)
|
|
#define VULKAN_HPP_NAMESPACE_STRING VULKAN_HPP_STRINGIFY(VULKAN_HPP_NAMESPACE)
|
|
|
|
namespace VULKAN_HPP_NAMESPACE
|
|
{
|
|
#if !defined(VULKAN_HPP_DISABLE_ENHANCED_MODE)
|
|
template <typename T>
|
|
class ArrayProxy
|
|
{
|
|
public:
|
|
VULKAN_HPP_CONSTEXPR ArrayProxy(std::nullptr_t) VULKAN_HPP_NOEXCEPT
|
|
: m_count(0)
|
|
, m_ptr(nullptr)
|
|
{}
|
|
|
|
ArrayProxy(typename std::remove_reference<T>::type & ptr) VULKAN_HPP_NOEXCEPT
|
|
: m_count(1)
|
|
, m_ptr(&ptr)
|
|
{}
|
|
|
|
ArrayProxy(uint32_t count, T * ptr) VULKAN_HPP_NOEXCEPT
|
|
: m_count(count)
|
|
, m_ptr(ptr)
|
|
{}
|
|
|
|
template <size_t N>
|
|
ArrayProxy(std::array<typename std::remove_const<T>::type, N> & data) VULKAN_HPP_NOEXCEPT
|
|
: m_count(N)
|
|
, m_ptr(data.data())
|
|
{}
|
|
|
|
template <size_t N>
|
|
ArrayProxy(std::array<typename std::remove_const<T>::type, N> const& data) VULKAN_HPP_NOEXCEPT
|
|
: m_count(N)
|
|
, m_ptr(data.data())
|
|
{}
|
|
|
|
template <class Allocator = std::allocator<typename std::remove_const<T>::type>>
|
|
ArrayProxy(std::vector<typename std::remove_const<T>::type, Allocator> & data) VULKAN_HPP_NOEXCEPT
|
|
: m_count(static_cast<uint32_t>(data.size()))
|
|
, m_ptr(data.data())
|
|
{}
|
|
|
|
template <class Allocator = std::allocator<typename std::remove_const<T>::type>>
|
|
ArrayProxy(std::vector<typename std::remove_const<T>::type, Allocator> const& data) VULKAN_HPP_NOEXCEPT
|
|
: m_count(static_cast<uint32_t>(data.size()))
|
|
, m_ptr(data.data())
|
|
{}
|
|
|
|
ArrayProxy(std::initializer_list<typename std::remove_reference<T>::type> const& data) VULKAN_HPP_NOEXCEPT
|
|
: m_count(static_cast<uint32_t>(data.end() - data.begin()))
|
|
, m_ptr(data.begin())
|
|
{}
|
|
|
|
const T * begin() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_ptr;
|
|
}
|
|
|
|
const T * end() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_ptr + m_count;
|
|
}
|
|
|
|
const T & front() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
VULKAN_HPP_ASSERT(m_count && m_ptr);
|
|
return *m_ptr;
|
|
}
|
|
|
|
const T & back() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
VULKAN_HPP_ASSERT(m_count && m_ptr);
|
|
return *(m_ptr + m_count - 1);
|
|
}
|
|
|
|
bool empty() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return (m_count == 0);
|
|
}
|
|
|
|
uint32_t size() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_count;
|
|
}
|
|
|
|
T * data() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_ptr;
|
|
}
|
|
|
|
private:
|
|
uint32_t m_count;
|
|
T * m_ptr;
|
|
};
|
|
#endif
|
|
|
|
template <typename FlagBitsType> struct FlagTraits
|
|
{
|
|
enum { allFlags = 0 };
|
|
};
|
|
|
|
template <typename BitType>
|
|
class Flags
|
|
{
|
|
public:
|
|
using MaskType = typename std::underlying_type<BitType>::type;
|
|
|
|
// constructors
|
|
VULKAN_HPP_CONSTEXPR Flags() VULKAN_HPP_NOEXCEPT
|
|
: m_mask(0)
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR Flags(BitType bit) VULKAN_HPP_NOEXCEPT
|
|
: m_mask(static_cast<MaskType>(bit))
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR Flags(Flags<BitType> const& rhs) VULKAN_HPP_NOEXCEPT
|
|
: m_mask(rhs.m_mask)
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR explicit Flags(MaskType flags) VULKAN_HPP_NOEXCEPT
|
|
: m_mask(flags)
|
|
{}
|
|
|
|
// relational operators
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>(Flags<BitType> const&) const = default;
|
|
#else
|
|
VULKAN_HPP_CONSTEXPR bool operator<(Flags<BitType> const& rhs) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_mask < rhs.m_mask;
|
|
}
|
|
|
|
VULKAN_HPP_CONSTEXPR bool operator<=(Flags<BitType> const& rhs) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_mask <= rhs.m_mask;
|
|
}
|
|
|
|
VULKAN_HPP_CONSTEXPR bool operator>(Flags<BitType> const& rhs) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_mask > rhs.m_mask;
|
|
}
|
|
|
|
VULKAN_HPP_CONSTEXPR bool operator>=(Flags<BitType> const& rhs) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_mask >= rhs.m_mask;
|
|
}
|
|
|
|
VULKAN_HPP_CONSTEXPR bool operator==(Flags<BitType> const& rhs) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_mask == rhs.m_mask;
|
|
}
|
|
|
|
VULKAN_HPP_CONSTEXPR bool operator!=(Flags<BitType> const& rhs) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_mask != rhs.m_mask;
|
|
}
|
|
#endif
|
|
|
|
// logical operator
|
|
VULKAN_HPP_CONSTEXPR bool operator!() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !m_mask;
|
|
}
|
|
|
|
// bitwise operators
|
|
VULKAN_HPP_CONSTEXPR Flags<BitType> operator&(Flags<BitType> const& rhs) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return Flags<BitType>(m_mask & rhs.m_mask);
|
|
}
|
|
|
|
VULKAN_HPP_CONSTEXPR Flags<BitType> operator|(Flags<BitType> const& rhs) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return Flags<BitType>(m_mask | rhs.m_mask);
|
|
}
|
|
|
|
VULKAN_HPP_CONSTEXPR Flags<BitType> operator^(Flags<BitType> const& rhs) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return Flags<BitType>(m_mask ^ rhs.m_mask);
|
|
}
|
|
|
|
VULKAN_HPP_CONSTEXPR Flags<BitType> operator~() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return Flags<BitType>(m_mask ^ FlagTraits<BitType>::allFlags);
|
|
}
|
|
|
|
// assignment operators
|
|
Flags<BitType> & operator=(Flags<BitType> const& rhs) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
m_mask = rhs.m_mask;
|
|
return *this;
|
|
}
|
|
|
|
Flags<BitType> & operator|=(Flags<BitType> const& rhs) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
m_mask |= rhs.m_mask;
|
|
return *this;
|
|
}
|
|
|
|
Flags<BitType> & operator&=(Flags<BitType> const& rhs) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
m_mask &= rhs.m_mask;
|
|
return *this;
|
|
}
|
|
|
|
Flags<BitType> & operator^=(Flags<BitType> const& rhs) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
m_mask ^= rhs.m_mask;
|
|
return *this;
|
|
}
|
|
|
|
// cast operators
|
|
explicit VULKAN_HPP_CONSTEXPR operator bool() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !!m_mask;
|
|
}
|
|
|
|
explicit VULKAN_HPP_CONSTEXPR operator MaskType() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_mask;
|
|
}
|
|
|
|
private:
|
|
MaskType m_mask;
|
|
};
|
|
|
|
#if !defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
// relational operators only needed for pre C++20
|
|
template <typename BitType>
|
|
VULKAN_HPP_CONSTEXPR bool operator<(BitType bit, Flags<BitType> const& flags) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return flags > bit;
|
|
}
|
|
|
|
template <typename BitType>
|
|
VULKAN_HPP_CONSTEXPR bool operator<=(BitType bit, Flags<BitType> const& flags) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return flags >= bit;
|
|
}
|
|
|
|
template <typename BitType>
|
|
VULKAN_HPP_CONSTEXPR bool operator>(BitType bit, Flags<BitType> const& flags) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return flags < bit;
|
|
}
|
|
|
|
template <typename BitType>
|
|
VULKAN_HPP_CONSTEXPR bool operator>=(BitType bit, Flags<BitType> const& flags) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return flags <= bit;
|
|
}
|
|
|
|
template <typename BitType>
|
|
VULKAN_HPP_CONSTEXPR bool operator==(BitType bit, Flags<BitType> const& flags) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return flags == bit;
|
|
}
|
|
|
|
template <typename BitType>
|
|
VULKAN_HPP_CONSTEXPR bool operator!=(BitType bit, Flags<BitType> const& flags) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return flags != bit;
|
|
}
|
|
#endif
|
|
|
|
// bitwise operators
|
|
template <typename BitType>
|
|
VULKAN_HPP_CONSTEXPR Flags<BitType> operator&(BitType bit, Flags<BitType> const& flags) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return flags & bit;
|
|
}
|
|
|
|
template <typename BitType>
|
|
VULKAN_HPP_CONSTEXPR Flags<BitType> operator|(BitType bit, Flags<BitType> const& flags) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return flags | bit;
|
|
}
|
|
|
|
template <typename BitType>
|
|
VULKAN_HPP_CONSTEXPR Flags<BitType> operator^(BitType bit, Flags<BitType> const& flags) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return flags ^ bit;
|
|
}
|
|
|
|
template <typename RefType>
|
|
class Optional
|
|
{
|
|
public:
|
|
Optional(RefType & reference) VULKAN_HPP_NOEXCEPT { m_ptr = &reference; }
|
|
Optional(RefType * ptr) VULKAN_HPP_NOEXCEPT { m_ptr = ptr; }
|
|
Optional(std::nullptr_t) VULKAN_HPP_NOEXCEPT { m_ptr = nullptr; }
|
|
|
|
operator RefType*() const VULKAN_HPP_NOEXCEPT { return m_ptr; }
|
|
RefType const* operator->() const VULKAN_HPP_NOEXCEPT { return m_ptr; }
|
|
explicit operator bool() const VULKAN_HPP_NOEXCEPT { return !!m_ptr; }
|
|
|
|
private:
|
|
RefType *m_ptr;
|
|
};
|
|
|
|
template <typename X, typename Y> struct isStructureChainValid { enum { value = false }; };
|
|
|
|
template <typename P, typename T>
|
|
struct TypeList
|
|
{
|
|
using list = P;
|
|
using last = T;
|
|
};
|
|
|
|
template <typename List, typename X>
|
|
struct extendCheck
|
|
{
|
|
static const bool valid = isStructureChainValid<typename List::last, X>::value || extendCheck<typename List::list,X>::valid;
|
|
};
|
|
|
|
template <typename T, typename X>
|
|
struct extendCheck<TypeList<void,T>,X>
|
|
{
|
|
static const bool valid = isStructureChainValid<T, X>::value;
|
|
};
|
|
|
|
template <typename X>
|
|
struct extendCheck<void,X>
|
|
{
|
|
static const bool valid = true;
|
|
};
|
|
|
|
template<typename Type, class...>
|
|
struct isPartOfStructureChain
|
|
{
|
|
static const bool valid = false;
|
|
};
|
|
|
|
template<typename Type, typename Head, typename... Tail>
|
|
struct isPartOfStructureChain<Type, Head, Tail...>
|
|
{
|
|
static const bool valid = std::is_same<Type, Head>::value || isPartOfStructureChain<Type, Tail...>::valid;
|
|
};
|
|
|
|
template <class Element>
|
|
class StructureChainElement
|
|
{
|
|
public:
|
|
explicit operator Element&() VULKAN_HPP_NOEXCEPT { return value; }
|
|
explicit operator const Element&() const VULKAN_HPP_NOEXCEPT { return value; }
|
|
private:
|
|
Element value;
|
|
};
|
|
|
|
template<typename ...StructureElements>
|
|
class StructureChain : private StructureChainElement<StructureElements>...
|
|
{
|
|
public:
|
|
StructureChain() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
link<void, StructureElements...>();
|
|
}
|
|
|
|
StructureChain(StructureChain const &rhs) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
linkAndCopy<void, StructureElements...>(rhs);
|
|
}
|
|
|
|
StructureChain(StructureElements const &... elems) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
linkAndCopyElements<void, StructureElements...>(elems...);
|
|
}
|
|
|
|
StructureChain& operator=(StructureChain const &rhs) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
linkAndCopy<void, StructureElements...>(rhs);
|
|
return *this;
|
|
}
|
|
|
|
template<typename ClassType> ClassType& get() VULKAN_HPP_NOEXCEPT { return static_cast<ClassType&>(*this);}
|
|
|
|
template<typename ClassType> const ClassType& get() const VULKAN_HPP_NOEXCEPT { return static_cast<const ClassType&>(*this);}
|
|
|
|
template<typename ClassTypeA, typename ClassTypeB, typename ...ClassTypes>
|
|
std::tuple<ClassTypeA&, ClassTypeB&, ClassTypes&...> get()
|
|
{
|
|
return std::tie(get<ClassTypeA>(), get<ClassTypeB>(), get<ClassTypes>()...);
|
|
}
|
|
|
|
template<typename ClassTypeA, typename ClassTypeB, typename ...ClassTypes>
|
|
std::tuple<const ClassTypeA&, const ClassTypeB&, const ClassTypes&...> get() const
|
|
{
|
|
return std::tie(get<ClassTypeA>(), get<ClassTypeB>(), get<ClassTypes>()...);
|
|
}
|
|
|
|
template<typename ClassType>
|
|
void unlink() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
static_assert(isPartOfStructureChain<ClassType, StructureElements...>::valid, "Can't unlink Structure that's not part of this StructureChain!");
|
|
static_assert(!std::is_same<ClassType, typename std::tuple_element<0, std::tuple<StructureElements...>>::type>::value, "It's not allowed to unlink the first element!");
|
|
VkBaseOutStructure * ptr = reinterpret_cast<VkBaseOutStructure*>(&get<ClassType>());
|
|
VULKAN_HPP_ASSERT(ptr != nullptr);
|
|
VkBaseOutStructure ** ppNext = &(reinterpret_cast<VkBaseOutStructure*>(this)->pNext);
|
|
VULKAN_HPP_ASSERT(*ppNext != nullptr);
|
|
while (*ppNext != ptr)
|
|
{
|
|
ppNext = &(*ppNext)->pNext;
|
|
VULKAN_HPP_ASSERT(*ppNext != nullptr); // fires, if the ClassType member has already been unlinked !
|
|
}
|
|
VULKAN_HPP_ASSERT(*ppNext == ptr);
|
|
*ppNext = (*ppNext)->pNext;
|
|
}
|
|
|
|
template <typename ClassType>
|
|
void relink() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
static_assert(isPartOfStructureChain<ClassType, StructureElements...>::valid, "Can't relink Structure that's not part of this StructureChain!");
|
|
static_assert(!std::is_same<ClassType, typename std::tuple_element<0, std::tuple<StructureElements...>>::type>::value, "It's not allowed to have the first element unlinked!");
|
|
VkBaseOutStructure * ptr = reinterpret_cast<VkBaseOutStructure*>(&get<ClassType>());
|
|
VULKAN_HPP_ASSERT(ptr != nullptr);
|
|
VkBaseOutStructure ** ppNext = &(reinterpret_cast<VkBaseOutStructure*>(this)->pNext);
|
|
VULKAN_HPP_ASSERT(*ppNext != nullptr);
|
|
#if !defined(NDEBUG)
|
|
while (*ppNext)
|
|
{
|
|
VULKAN_HPP_ASSERT(*ppNext != ptr); // fires, if the ClassType member has not been unlinked before
|
|
ppNext = &(*ppNext)->pNext;
|
|
}
|
|
ppNext = &(reinterpret_cast<VkBaseOutStructure*>(this)->pNext);
|
|
#endif
|
|
ptr->pNext = *ppNext;
|
|
*ppNext = ptr;
|
|
}
|
|
|
|
private:
|
|
template<typename List, typename X>
|
|
void link() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
static_assert(extendCheck<List, X>::valid, "The structure chain is not valid!");
|
|
}
|
|
|
|
template<typename List, typename X, typename Y, typename ...Z>
|
|
void link() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
static_assert(extendCheck<List,X>::valid, "The structure chain is not valid!");
|
|
X& x = static_cast<X&>(*this);
|
|
Y& y = static_cast<Y&>(*this);
|
|
x.pNext = &y;
|
|
link<TypeList<List, X>, Y, Z...>();
|
|
}
|
|
|
|
template<typename List, typename X>
|
|
void linkAndCopy(StructureChain const &rhs) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
static_assert(extendCheck<List, X>::valid, "The structure chain is not valid!");
|
|
static_cast<X&>(*this) = static_cast<X const &>(rhs);
|
|
}
|
|
|
|
template<typename List, typename X, typename Y, typename ...Z>
|
|
void linkAndCopy(StructureChain const &rhs) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
static_assert(extendCheck<List, X>::valid, "The structure chain is not valid!");
|
|
X& x = static_cast<X&>(*this);
|
|
Y& y = static_cast<Y&>(*this);
|
|
x = static_cast<X const &>(rhs);
|
|
x.pNext = &y;
|
|
linkAndCopy<TypeList<List, X>, Y, Z...>(rhs);
|
|
}
|
|
|
|
template<typename List, typename X>
|
|
void linkAndCopyElements(X const &xelem) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
static_assert(extendCheck<List, X>::valid, "The structure chain is not valid!");
|
|
static_cast<X&>(*this) = xelem;
|
|
}
|
|
|
|
template<typename List, typename X, typename Y, typename ...Z>
|
|
void linkAndCopyElements(X const &xelem, Y const &yelem, Z const &... zelem) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
static_assert(extendCheck<List, X>::valid, "The structure chain is not valid!");
|
|
X& x = static_cast<X&>(*this);
|
|
Y& y = static_cast<Y&>(*this);
|
|
x = xelem;
|
|
x.pNext = &y;
|
|
linkAndCopyElements<TypeList<List, X>, Y, Z...>(yelem, zelem...);
|
|
}
|
|
};
|
|
|
|
#if !defined(VULKAN_HPP_NO_SMART_HANDLE)
|
|
template <typename Type, typename Dispatch> class UniqueHandleTraits;
|
|
|
|
template <typename Type, typename Dispatch>
|
|
class UniqueHandle : public UniqueHandleTraits<Type,Dispatch>::deleter
|
|
{
|
|
private:
|
|
using Deleter = typename UniqueHandleTraits<Type,Dispatch>::deleter;
|
|
|
|
public:
|
|
using element_type = Type;
|
|
|
|
UniqueHandle()
|
|
: Deleter()
|
|
, m_value()
|
|
{}
|
|
|
|
explicit UniqueHandle( Type const& value, Deleter const& deleter = Deleter() ) VULKAN_HPP_NOEXCEPT
|
|
: Deleter( deleter)
|
|
, m_value( value )
|
|
{}
|
|
|
|
UniqueHandle( UniqueHandle const& ) = delete;
|
|
|
|
UniqueHandle( UniqueHandle && other ) VULKAN_HPP_NOEXCEPT
|
|
: Deleter( std::move( static_cast<Deleter&>( other ) ) )
|
|
, m_value( other.release() )
|
|
{}
|
|
|
|
~UniqueHandle() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
if ( m_value ) this->destroy( m_value );
|
|
}
|
|
|
|
UniqueHandle & operator=( UniqueHandle const& ) = delete;
|
|
|
|
UniqueHandle & operator=( UniqueHandle && other ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
reset( other.release() );
|
|
*static_cast<Deleter*>(this) = std::move( static_cast<Deleter&>(other) );
|
|
return *this;
|
|
}
|
|
|
|
explicit operator bool() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_value.operator bool();
|
|
}
|
|
|
|
Type const* operator->() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return &m_value;
|
|
}
|
|
|
|
Type * operator->() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return &m_value;
|
|
}
|
|
|
|
Type const& operator*() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_value;
|
|
}
|
|
|
|
Type & operator*() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_value;
|
|
}
|
|
|
|
const Type & get() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_value;
|
|
}
|
|
|
|
Type & get() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return m_value;
|
|
}
|
|
|
|
void reset( Type const& value = Type() ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
if ( m_value != value )
|
|
{
|
|
if ( m_value ) this->destroy( m_value );
|
|
m_value = value;
|
|
}
|
|
}
|
|
|
|
Type release() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
Type value = m_value;
|
|
m_value = nullptr;
|
|
return value;
|
|
}
|
|
|
|
void swap( UniqueHandle<Type,Dispatch> & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
std::swap(m_value, rhs.m_value);
|
|
std::swap(static_cast<Deleter&>(*this), static_cast<Deleter&>(rhs));
|
|
}
|
|
|
|
private:
|
|
Type m_value;
|
|
};
|
|
|
|
template <typename UniqueType>
|
|
VULKAN_HPP_INLINE std::vector<typename UniqueType::element_type> uniqueToRaw(std::vector<UniqueType> const& handles)
|
|
{
|
|
std::vector<typename UniqueType::element_type> newBuffer(handles.size());
|
|
std::transform(handles.begin(), handles.end(), newBuffer.begin(), [](UniqueType const& handle) { return handle.get(); });
|
|
return newBuffer;
|
|
}
|
|
|
|
template <typename Type, typename Dispatch>
|
|
VULKAN_HPP_INLINE void swap( UniqueHandle<Type,Dispatch> & lhs, UniqueHandle<Type,Dispatch> & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
lhs.swap( rhs );
|
|
}
|
|
#endif
|
|
} // namespace VULKAN_HPP_NAMESPACE
|