mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-11-17 00:49:16 +01:00
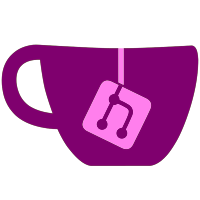
This commit adds Vulkan-Hpp as a library to the project. The headers are from a modified version of `VulkanHppGenerator`. They are broken into multiple files to avoid exceeding the Intellisense file size limit of Android Studio.
186 lines
9.7 KiB
C++
186 lines
9.7 KiB
C++
// Copyright (c) 2015-2019 The Khronos Group Inc.
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
//
|
|
// ---- Exceptions to the Apache 2.0 License: ----
|
|
//
|
|
// As an exception, if you use this Software to generate code and portions of
|
|
// this Software are embedded into the generated code as a result, you may
|
|
// redistribute such product without providing attribution as would otherwise
|
|
// be required by Sections 4(a), 4(b) and 4(d) of the License.
|
|
//
|
|
// In addition, if you combine or link code generated by this Software with
|
|
// software that is licensed under the GPLv2 or the LGPL v2.0 or 2.1
|
|
// ("`Combined Software`") and if a court of competent jurisdiction determines
|
|
// that the patent provision (Section 3), the indemnity provision (Section 9)
|
|
// or other Section of the License conflicts with the conditions of the
|
|
// applicable GPL or LGPL license, you may retroactively and prospectively
|
|
// choose to deem waived or otherwise exclude such Section(s) of the License,
|
|
// but only in their entirety and only with respect to the Combined Software.
|
|
//
|
|
|
|
// This header is generated from the Khronos Vulkan XML API Registry.
|
|
|
|
#pragma once
|
|
|
|
#include "../structs.hpp"
|
|
|
|
namespace VULKAN_HPP_NAMESPACE
|
|
{
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result createInstance( const VULKAN_HPP_NAMESPACE::InstanceCreateInfo* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::Instance* pInstance, Dispatch const &d) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateInstance( reinterpret_cast<const VkInstanceCreateInfo*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkInstance*>( pInstance ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::Instance>::type createInstance( const InstanceCreateInfo & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d )
|
|
{
|
|
VULKAN_HPP_NAMESPACE::Instance instance;
|
|
Result result = static_cast<Result>( d.vkCreateInstance( reinterpret_cast<const VkInstanceCreateInfo*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkInstance*>( &instance ) ) );
|
|
return createResultValue( result, instance, VULKAN_HPP_NAMESPACE_STRING"::createInstance" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<Instance,Dispatch>>::type createInstanceUnique( const InstanceCreateInfo & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d )
|
|
{
|
|
VULKAN_HPP_NAMESPACE::Instance instance;
|
|
Result result = static_cast<Result>( d.vkCreateInstance( reinterpret_cast<const VkInstanceCreateInfo*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkInstance*>( &instance ) ) );
|
|
|
|
ObjectDestroy<NoParent,Dispatch> deleter( allocator, d );
|
|
return createResultValue<Instance,Dispatch>( result, instance, VULKAN_HPP_NAMESPACE_STRING"::createInstanceUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result enumerateInstanceExtensionProperties( const char* pLayerName, uint32_t* pPropertyCount, VULKAN_HPP_NAMESPACE::ExtensionProperties* pProperties, Dispatch const &d) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkEnumerateInstanceExtensionProperties( pLayerName, pPropertyCount, reinterpret_cast<VkExtensionProperties*>( pProperties ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Allocator, typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<std::vector<ExtensionProperties,Allocator>>::type enumerateInstanceExtensionProperties( Optional<const std::string> layerName, Dispatch const &d )
|
|
{
|
|
std::vector<ExtensionProperties,Allocator> properties;
|
|
uint32_t propertyCount;
|
|
Result result;
|
|
do
|
|
{
|
|
result = static_cast<Result>( d.vkEnumerateInstanceExtensionProperties( layerName ? layerName->c_str() : nullptr, &propertyCount, nullptr ) );
|
|
if ( ( result == Result::eSuccess ) && propertyCount )
|
|
{
|
|
properties.resize( propertyCount );
|
|
result = static_cast<Result>( d.vkEnumerateInstanceExtensionProperties( layerName ? layerName->c_str() : nullptr, &propertyCount, reinterpret_cast<VkExtensionProperties*>( properties.data() ) ) );
|
|
}
|
|
} while ( result == Result::eIncomplete );
|
|
if ( result == Result::eSuccess )
|
|
{
|
|
VULKAN_HPP_ASSERT( propertyCount <= properties.size() );
|
|
properties.resize( propertyCount );
|
|
}
|
|
return createResultValue( result, properties, VULKAN_HPP_NAMESPACE_STRING"::enumerateInstanceExtensionProperties" );
|
|
}
|
|
template<typename Allocator, typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<std::vector<ExtensionProperties,Allocator>>::type enumerateInstanceExtensionProperties( Optional<const std::string> layerName, Allocator const& vectorAllocator, Dispatch const &d )
|
|
{
|
|
std::vector<ExtensionProperties,Allocator> properties( vectorAllocator );
|
|
uint32_t propertyCount;
|
|
Result result;
|
|
do
|
|
{
|
|
result = static_cast<Result>( d.vkEnumerateInstanceExtensionProperties( layerName ? layerName->c_str() : nullptr, &propertyCount, nullptr ) );
|
|
if ( ( result == Result::eSuccess ) && propertyCount )
|
|
{
|
|
properties.resize( propertyCount );
|
|
result = static_cast<Result>( d.vkEnumerateInstanceExtensionProperties( layerName ? layerName->c_str() : nullptr, &propertyCount, reinterpret_cast<VkExtensionProperties*>( properties.data() ) ) );
|
|
}
|
|
} while ( result == Result::eIncomplete );
|
|
if ( result == Result::eSuccess )
|
|
{
|
|
VULKAN_HPP_ASSERT( propertyCount <= properties.size() );
|
|
properties.resize( propertyCount );
|
|
}
|
|
return createResultValue( result, properties, VULKAN_HPP_NAMESPACE_STRING"::enumerateInstanceExtensionProperties" );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result enumerateInstanceLayerProperties( uint32_t* pPropertyCount, VULKAN_HPP_NAMESPACE::LayerProperties* pProperties, Dispatch const &d) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkEnumerateInstanceLayerProperties( pPropertyCount, reinterpret_cast<VkLayerProperties*>( pProperties ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Allocator, typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<std::vector<LayerProperties,Allocator>>::type enumerateInstanceLayerProperties(Dispatch const &d )
|
|
{
|
|
std::vector<LayerProperties,Allocator> properties;
|
|
uint32_t propertyCount;
|
|
Result result;
|
|
do
|
|
{
|
|
result = static_cast<Result>( d.vkEnumerateInstanceLayerProperties( &propertyCount, nullptr ) );
|
|
if ( ( result == Result::eSuccess ) && propertyCount )
|
|
{
|
|
properties.resize( propertyCount );
|
|
result = static_cast<Result>( d.vkEnumerateInstanceLayerProperties( &propertyCount, reinterpret_cast<VkLayerProperties*>( properties.data() ) ) );
|
|
}
|
|
} while ( result == Result::eIncomplete );
|
|
if ( result == Result::eSuccess )
|
|
{
|
|
VULKAN_HPP_ASSERT( propertyCount <= properties.size() );
|
|
properties.resize( propertyCount );
|
|
}
|
|
return createResultValue( result, properties, VULKAN_HPP_NAMESPACE_STRING"::enumerateInstanceLayerProperties" );
|
|
}
|
|
template<typename Allocator, typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<std::vector<LayerProperties,Allocator>>::type enumerateInstanceLayerProperties(Allocator const& vectorAllocator, Dispatch const &d )
|
|
{
|
|
std::vector<LayerProperties,Allocator> properties( vectorAllocator );
|
|
uint32_t propertyCount;
|
|
Result result;
|
|
do
|
|
{
|
|
result = static_cast<Result>( d.vkEnumerateInstanceLayerProperties( &propertyCount, nullptr ) );
|
|
if ( ( result == Result::eSuccess ) && propertyCount )
|
|
{
|
|
properties.resize( propertyCount );
|
|
result = static_cast<Result>( d.vkEnumerateInstanceLayerProperties( &propertyCount, reinterpret_cast<VkLayerProperties*>( properties.data() ) ) );
|
|
}
|
|
} while ( result == Result::eIncomplete );
|
|
if ( result == Result::eSuccess )
|
|
{
|
|
VULKAN_HPP_ASSERT( propertyCount <= properties.size() );
|
|
properties.resize( propertyCount );
|
|
}
|
|
return createResultValue( result, properties, VULKAN_HPP_NAMESPACE_STRING"::enumerateInstanceLayerProperties" );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result enumerateInstanceVersion( uint32_t* pApiVersion, Dispatch const &d) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkEnumerateInstanceVersion( pApiVersion ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<uint32_t>::type enumerateInstanceVersion(Dispatch const &d )
|
|
{
|
|
uint32_t apiVersion;
|
|
Result result = static_cast<Result>( d.vkEnumerateInstanceVersion( &apiVersion ) );
|
|
return createResultValue( result, apiVersion, VULKAN_HPP_NAMESPACE_STRING"::enumerateInstanceVersion" );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
} // namespace VULKAN_HPP_NAMESPACE
|