mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-06-02 02:38:43 +02:00
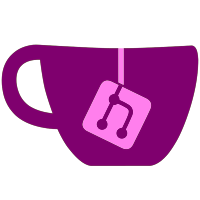
This commit adds Vulkan-Hpp as a library to the project. The headers are from a modified version of `VulkanHppGenerator`. They are broken into multiple files to avoid exceeding the Intellisense file size limit of Android Studio.
747 lines
55 KiB
C++
747 lines
55 KiB
C++
// Copyright (c) 2015-2019 The Khronos Group Inc.
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
//
|
|
// ---- Exceptions to the Apache 2.0 License: ----
|
|
//
|
|
// As an exception, if you use this Software to generate code and portions of
|
|
// this Software are embedded into the generated code as a result, you may
|
|
// redistribute such product without providing attribution as would otherwise
|
|
// be required by Sections 4(a), 4(b) and 4(d) of the License.
|
|
//
|
|
// In addition, if you combine or link code generated by this Software with
|
|
// software that is licensed under the GPLv2 or the LGPL v2.0 or 2.1
|
|
// ("`Combined Software`") and if a court of competent jurisdiction determines
|
|
// that the patent provision (Section 3), the indemnity provision (Section 9)
|
|
// or other Section of the License conflicts with the conditions of the
|
|
// applicable GPL or LGPL license, you may retroactively and prospectively
|
|
// choose to deem waived or otherwise exclude such Section(s) of the License,
|
|
// but only in their entirety and only with respect to the Combined Software.
|
|
//
|
|
|
|
// This header is generated from the Khronos Vulkan XML API Registry.
|
|
|
|
#pragma once
|
|
|
|
#include "../structs.hpp"
|
|
|
|
namespace VULKAN_HPP_NAMESPACE
|
|
{
|
|
|
|
#ifdef VK_USE_PLATFORM_ANDROID_KHR
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createAndroidSurfaceKHR( const VULKAN_HPP_NAMESPACE::AndroidSurfaceCreateInfoKHR* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::SurfaceKHR* pSurface, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateAndroidSurfaceKHR( m_instance, reinterpret_cast<const VkAndroidSurfaceCreateInfoKHR*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkSurfaceKHR*>( pSurface ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::SurfaceKHR>::type Instance::createAndroidSurfaceKHR( const AndroidSurfaceCreateInfoKHR & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateAndroidSurfaceKHR( m_instance, reinterpret_cast<const VkAndroidSurfaceCreateInfoKHR*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
return createResultValue( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createAndroidSurfaceKHR" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<SurfaceKHR,Dispatch>>::type Instance::createAndroidSurfaceKHRUnique( const AndroidSurfaceCreateInfoKHR & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateAndroidSurfaceKHR( m_instance, reinterpret_cast<const VkAndroidSurfaceCreateInfoKHR*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<SurfaceKHR,Dispatch>( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createAndroidSurfaceKHRUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
#endif /*VK_USE_PLATFORM_ANDROID_KHR*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createDebugReportCallbackEXT( const VULKAN_HPP_NAMESPACE::DebugReportCallbackCreateInfoEXT* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::DebugReportCallbackEXT* pCallback, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateDebugReportCallbackEXT( m_instance, reinterpret_cast<const VkDebugReportCallbackCreateInfoEXT*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkDebugReportCallbackEXT*>( pCallback ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::DebugReportCallbackEXT>::type Instance::createDebugReportCallbackEXT( const DebugReportCallbackCreateInfoEXT & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::DebugReportCallbackEXT callback;
|
|
Result result = static_cast<Result>( d.vkCreateDebugReportCallbackEXT( m_instance, reinterpret_cast<const VkDebugReportCallbackCreateInfoEXT*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkDebugReportCallbackEXT*>( &callback ) ) );
|
|
return createResultValue( result, callback, VULKAN_HPP_NAMESPACE_STRING"::Instance::createDebugReportCallbackEXT" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<DebugReportCallbackEXT,Dispatch>>::type Instance::createDebugReportCallbackEXTUnique( const DebugReportCallbackCreateInfoEXT & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::DebugReportCallbackEXT callback;
|
|
Result result = static_cast<Result>( d.vkCreateDebugReportCallbackEXT( m_instance, reinterpret_cast<const VkDebugReportCallbackCreateInfoEXT*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkDebugReportCallbackEXT*>( &callback ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<DebugReportCallbackEXT,Dispatch>( result, callback, VULKAN_HPP_NAMESPACE_STRING"::Instance::createDebugReportCallbackEXTUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createDebugUtilsMessengerEXT( const VULKAN_HPP_NAMESPACE::DebugUtilsMessengerCreateInfoEXT* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::DebugUtilsMessengerEXT* pMessenger, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateDebugUtilsMessengerEXT( m_instance, reinterpret_cast<const VkDebugUtilsMessengerCreateInfoEXT*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkDebugUtilsMessengerEXT*>( pMessenger ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::DebugUtilsMessengerEXT>::type Instance::createDebugUtilsMessengerEXT( const DebugUtilsMessengerCreateInfoEXT & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::DebugUtilsMessengerEXT messenger;
|
|
Result result = static_cast<Result>( d.vkCreateDebugUtilsMessengerEXT( m_instance, reinterpret_cast<const VkDebugUtilsMessengerCreateInfoEXT*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkDebugUtilsMessengerEXT*>( &messenger ) ) );
|
|
return createResultValue( result, messenger, VULKAN_HPP_NAMESPACE_STRING"::Instance::createDebugUtilsMessengerEXT" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<DebugUtilsMessengerEXT,Dispatch>>::type Instance::createDebugUtilsMessengerEXTUnique( const DebugUtilsMessengerCreateInfoEXT & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::DebugUtilsMessengerEXT messenger;
|
|
Result result = static_cast<Result>( d.vkCreateDebugUtilsMessengerEXT( m_instance, reinterpret_cast<const VkDebugUtilsMessengerCreateInfoEXT*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkDebugUtilsMessengerEXT*>( &messenger ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<DebugUtilsMessengerEXT,Dispatch>( result, messenger, VULKAN_HPP_NAMESPACE_STRING"::Instance::createDebugUtilsMessengerEXTUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createDisplayPlaneSurfaceKHR( const VULKAN_HPP_NAMESPACE::DisplaySurfaceCreateInfoKHR* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::SurfaceKHR* pSurface, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateDisplayPlaneSurfaceKHR( m_instance, reinterpret_cast<const VkDisplaySurfaceCreateInfoKHR*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkSurfaceKHR*>( pSurface ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::SurfaceKHR>::type Instance::createDisplayPlaneSurfaceKHR( const DisplaySurfaceCreateInfoKHR & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateDisplayPlaneSurfaceKHR( m_instance, reinterpret_cast<const VkDisplaySurfaceCreateInfoKHR*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
return createResultValue( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createDisplayPlaneSurfaceKHR" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<SurfaceKHR,Dispatch>>::type Instance::createDisplayPlaneSurfaceKHRUnique( const DisplaySurfaceCreateInfoKHR & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateDisplayPlaneSurfaceKHR( m_instance, reinterpret_cast<const VkDisplaySurfaceCreateInfoKHR*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<SurfaceKHR,Dispatch>( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createDisplayPlaneSurfaceKHRUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createHeadlessSurfaceEXT( const VULKAN_HPP_NAMESPACE::HeadlessSurfaceCreateInfoEXT* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::SurfaceKHR* pSurface, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateHeadlessSurfaceEXT( m_instance, reinterpret_cast<const VkHeadlessSurfaceCreateInfoEXT*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkSurfaceKHR*>( pSurface ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::SurfaceKHR>::type Instance::createHeadlessSurfaceEXT( const HeadlessSurfaceCreateInfoEXT & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateHeadlessSurfaceEXT( m_instance, reinterpret_cast<const VkHeadlessSurfaceCreateInfoEXT*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
return createResultValue( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createHeadlessSurfaceEXT" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<SurfaceKHR,Dispatch>>::type Instance::createHeadlessSurfaceEXTUnique( const HeadlessSurfaceCreateInfoEXT & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateHeadlessSurfaceEXT( m_instance, reinterpret_cast<const VkHeadlessSurfaceCreateInfoEXT*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<SurfaceKHR,Dispatch>( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createHeadlessSurfaceEXTUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
#ifdef VK_USE_PLATFORM_IOS_MVK
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createIOSSurfaceMVK( const VULKAN_HPP_NAMESPACE::IOSSurfaceCreateInfoMVK* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::SurfaceKHR* pSurface, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateIOSSurfaceMVK( m_instance, reinterpret_cast<const VkIOSSurfaceCreateInfoMVK*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkSurfaceKHR*>( pSurface ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::SurfaceKHR>::type Instance::createIOSSurfaceMVK( const IOSSurfaceCreateInfoMVK & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateIOSSurfaceMVK( m_instance, reinterpret_cast<const VkIOSSurfaceCreateInfoMVK*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
return createResultValue( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createIOSSurfaceMVK" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<SurfaceKHR,Dispatch>>::type Instance::createIOSSurfaceMVKUnique( const IOSSurfaceCreateInfoMVK & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateIOSSurfaceMVK( m_instance, reinterpret_cast<const VkIOSSurfaceCreateInfoMVK*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<SurfaceKHR,Dispatch>( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createIOSSurfaceMVKUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
#endif /*VK_USE_PLATFORM_IOS_MVK*/
|
|
|
|
#ifdef VK_USE_PLATFORM_FUCHSIA
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createImagePipeSurfaceFUCHSIA( const VULKAN_HPP_NAMESPACE::ImagePipeSurfaceCreateInfoFUCHSIA* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::SurfaceKHR* pSurface, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateImagePipeSurfaceFUCHSIA( m_instance, reinterpret_cast<const VkImagePipeSurfaceCreateInfoFUCHSIA*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkSurfaceKHR*>( pSurface ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::SurfaceKHR>::type Instance::createImagePipeSurfaceFUCHSIA( const ImagePipeSurfaceCreateInfoFUCHSIA & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateImagePipeSurfaceFUCHSIA( m_instance, reinterpret_cast<const VkImagePipeSurfaceCreateInfoFUCHSIA*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
return createResultValue( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createImagePipeSurfaceFUCHSIA" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<SurfaceKHR,Dispatch>>::type Instance::createImagePipeSurfaceFUCHSIAUnique( const ImagePipeSurfaceCreateInfoFUCHSIA & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateImagePipeSurfaceFUCHSIA( m_instance, reinterpret_cast<const VkImagePipeSurfaceCreateInfoFUCHSIA*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<SurfaceKHR,Dispatch>( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createImagePipeSurfaceFUCHSIAUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
#endif /*VK_USE_PLATFORM_FUCHSIA*/
|
|
|
|
#ifdef VK_USE_PLATFORM_MACOS_MVK
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createMacOSSurfaceMVK( const VULKAN_HPP_NAMESPACE::MacOSSurfaceCreateInfoMVK* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::SurfaceKHR* pSurface, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateMacOSSurfaceMVK( m_instance, reinterpret_cast<const VkMacOSSurfaceCreateInfoMVK*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkSurfaceKHR*>( pSurface ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::SurfaceKHR>::type Instance::createMacOSSurfaceMVK( const MacOSSurfaceCreateInfoMVK & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateMacOSSurfaceMVK( m_instance, reinterpret_cast<const VkMacOSSurfaceCreateInfoMVK*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
return createResultValue( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createMacOSSurfaceMVK" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<SurfaceKHR,Dispatch>>::type Instance::createMacOSSurfaceMVKUnique( const MacOSSurfaceCreateInfoMVK & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateMacOSSurfaceMVK( m_instance, reinterpret_cast<const VkMacOSSurfaceCreateInfoMVK*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<SurfaceKHR,Dispatch>( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createMacOSSurfaceMVKUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
#endif /*VK_USE_PLATFORM_MACOS_MVK*/
|
|
|
|
#ifdef VK_USE_PLATFORM_METAL_EXT
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createMetalSurfaceEXT( const VULKAN_HPP_NAMESPACE::MetalSurfaceCreateInfoEXT* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::SurfaceKHR* pSurface, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateMetalSurfaceEXT( m_instance, reinterpret_cast<const VkMetalSurfaceCreateInfoEXT*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkSurfaceKHR*>( pSurface ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::SurfaceKHR>::type Instance::createMetalSurfaceEXT( const MetalSurfaceCreateInfoEXT & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateMetalSurfaceEXT( m_instance, reinterpret_cast<const VkMetalSurfaceCreateInfoEXT*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
return createResultValue( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createMetalSurfaceEXT" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<SurfaceKHR,Dispatch>>::type Instance::createMetalSurfaceEXTUnique( const MetalSurfaceCreateInfoEXT & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateMetalSurfaceEXT( m_instance, reinterpret_cast<const VkMetalSurfaceCreateInfoEXT*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<SurfaceKHR,Dispatch>( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createMetalSurfaceEXTUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
#endif /*VK_USE_PLATFORM_METAL_EXT*/
|
|
|
|
#ifdef VK_USE_PLATFORM_GGP
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createStreamDescriptorSurfaceGGP( const VULKAN_HPP_NAMESPACE::StreamDescriptorSurfaceCreateInfoGGP* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::SurfaceKHR* pSurface, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateStreamDescriptorSurfaceGGP( m_instance, reinterpret_cast<const VkStreamDescriptorSurfaceCreateInfoGGP*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkSurfaceKHR*>( pSurface ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::SurfaceKHR>::type Instance::createStreamDescriptorSurfaceGGP( const StreamDescriptorSurfaceCreateInfoGGP & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateStreamDescriptorSurfaceGGP( m_instance, reinterpret_cast<const VkStreamDescriptorSurfaceCreateInfoGGP*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
return createResultValue( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createStreamDescriptorSurfaceGGP" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<SurfaceKHR,Dispatch>>::type Instance::createStreamDescriptorSurfaceGGPUnique( const StreamDescriptorSurfaceCreateInfoGGP & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateStreamDescriptorSurfaceGGP( m_instance, reinterpret_cast<const VkStreamDescriptorSurfaceCreateInfoGGP*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<SurfaceKHR,Dispatch>( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createStreamDescriptorSurfaceGGPUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
#endif /*VK_USE_PLATFORM_GGP*/
|
|
|
|
#ifdef VK_USE_PLATFORM_VI_NN
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createViSurfaceNN( const VULKAN_HPP_NAMESPACE::ViSurfaceCreateInfoNN* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::SurfaceKHR* pSurface, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateViSurfaceNN( m_instance, reinterpret_cast<const VkViSurfaceCreateInfoNN*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkSurfaceKHR*>( pSurface ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::SurfaceKHR>::type Instance::createViSurfaceNN( const ViSurfaceCreateInfoNN & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateViSurfaceNN( m_instance, reinterpret_cast<const VkViSurfaceCreateInfoNN*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
return createResultValue( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createViSurfaceNN" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<SurfaceKHR,Dispatch>>::type Instance::createViSurfaceNNUnique( const ViSurfaceCreateInfoNN & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateViSurfaceNN( m_instance, reinterpret_cast<const VkViSurfaceCreateInfoNN*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<SurfaceKHR,Dispatch>( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createViSurfaceNNUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
#endif /*VK_USE_PLATFORM_VI_NN*/
|
|
|
|
#ifdef VK_USE_PLATFORM_WAYLAND_KHR
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createWaylandSurfaceKHR( const VULKAN_HPP_NAMESPACE::WaylandSurfaceCreateInfoKHR* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::SurfaceKHR* pSurface, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateWaylandSurfaceKHR( m_instance, reinterpret_cast<const VkWaylandSurfaceCreateInfoKHR*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkSurfaceKHR*>( pSurface ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::SurfaceKHR>::type Instance::createWaylandSurfaceKHR( const WaylandSurfaceCreateInfoKHR & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateWaylandSurfaceKHR( m_instance, reinterpret_cast<const VkWaylandSurfaceCreateInfoKHR*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
return createResultValue( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createWaylandSurfaceKHR" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<SurfaceKHR,Dispatch>>::type Instance::createWaylandSurfaceKHRUnique( const WaylandSurfaceCreateInfoKHR & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateWaylandSurfaceKHR( m_instance, reinterpret_cast<const VkWaylandSurfaceCreateInfoKHR*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<SurfaceKHR,Dispatch>( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createWaylandSurfaceKHRUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
#endif /*VK_USE_PLATFORM_WAYLAND_KHR*/
|
|
|
|
#ifdef VK_USE_PLATFORM_WIN32_KHR
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createWin32SurfaceKHR( const VULKAN_HPP_NAMESPACE::Win32SurfaceCreateInfoKHR* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::SurfaceKHR* pSurface, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateWin32SurfaceKHR( m_instance, reinterpret_cast<const VkWin32SurfaceCreateInfoKHR*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkSurfaceKHR*>( pSurface ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::SurfaceKHR>::type Instance::createWin32SurfaceKHR( const Win32SurfaceCreateInfoKHR & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateWin32SurfaceKHR( m_instance, reinterpret_cast<const VkWin32SurfaceCreateInfoKHR*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
return createResultValue( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createWin32SurfaceKHR" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<SurfaceKHR,Dispatch>>::type Instance::createWin32SurfaceKHRUnique( const Win32SurfaceCreateInfoKHR & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateWin32SurfaceKHR( m_instance, reinterpret_cast<const VkWin32SurfaceCreateInfoKHR*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<SurfaceKHR,Dispatch>( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createWin32SurfaceKHRUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
#endif /*VK_USE_PLATFORM_WIN32_KHR*/
|
|
|
|
#ifdef VK_USE_PLATFORM_XCB_KHR
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createXcbSurfaceKHR( const VULKAN_HPP_NAMESPACE::XcbSurfaceCreateInfoKHR* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::SurfaceKHR* pSurface, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateXcbSurfaceKHR( m_instance, reinterpret_cast<const VkXcbSurfaceCreateInfoKHR*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkSurfaceKHR*>( pSurface ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::SurfaceKHR>::type Instance::createXcbSurfaceKHR( const XcbSurfaceCreateInfoKHR & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateXcbSurfaceKHR( m_instance, reinterpret_cast<const VkXcbSurfaceCreateInfoKHR*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
return createResultValue( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createXcbSurfaceKHR" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<SurfaceKHR,Dispatch>>::type Instance::createXcbSurfaceKHRUnique( const XcbSurfaceCreateInfoKHR & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateXcbSurfaceKHR( m_instance, reinterpret_cast<const VkXcbSurfaceCreateInfoKHR*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<SurfaceKHR,Dispatch>( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createXcbSurfaceKHRUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
#endif /*VK_USE_PLATFORM_XCB_KHR*/
|
|
|
|
#ifdef VK_USE_PLATFORM_XLIB_KHR
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::createXlibSurfaceKHR( const VULKAN_HPP_NAMESPACE::XlibSurfaceCreateInfoKHR* pCreateInfo, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, VULKAN_HPP_NAMESPACE::SurfaceKHR* pSurface, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkCreateXlibSurfaceKHR( m_instance, reinterpret_cast<const VkXlibSurfaceCreateInfoKHR*>( pCreateInfo ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ), reinterpret_cast<VkSurfaceKHR*>( pSurface ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<VULKAN_HPP_NAMESPACE::SurfaceKHR>::type Instance::createXlibSurfaceKHR( const XlibSurfaceCreateInfoKHR & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateXlibSurfaceKHR( m_instance, reinterpret_cast<const VkXlibSurfaceCreateInfoKHR*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
return createResultValue( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createXlibSurfaceKHR" );
|
|
}
|
|
#ifndef VULKAN_HPP_NO_SMART_HANDLE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<UniqueHandle<SurfaceKHR,Dispatch>>::type Instance::createXlibSurfaceKHRUnique( const XlibSurfaceCreateInfoKHR & createInfo, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const
|
|
{
|
|
VULKAN_HPP_NAMESPACE::SurfaceKHR surface;
|
|
Result result = static_cast<Result>( d.vkCreateXlibSurfaceKHR( m_instance, reinterpret_cast<const VkXlibSurfaceCreateInfoKHR*>( &createInfo ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ), reinterpret_cast<VkSurfaceKHR*>( &surface ) ) );
|
|
|
|
ObjectDestroy<Instance,Dispatch> deleter( *this, allocator, d );
|
|
return createResultValue<SurfaceKHR,Dispatch>( result, surface, VULKAN_HPP_NAMESPACE_STRING"::Instance::createXlibSurfaceKHRUnique", deleter );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_SMART_HANDLE*/
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
#endif /*VK_USE_PLATFORM_XLIB_KHR*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::debugReportMessageEXT( VULKAN_HPP_NAMESPACE::DebugReportFlagsEXT flags, VULKAN_HPP_NAMESPACE::DebugReportObjectTypeEXT objectType, uint64_t object, size_t location, int32_t messageCode, const char* pLayerPrefix, const char* pMessage, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDebugReportMessageEXT( m_instance, static_cast<VkDebugReportFlagsEXT>( flags ), static_cast<VkDebugReportObjectTypeEXT>( objectType ), object, location, messageCode, pLayerPrefix, pMessage );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::debugReportMessageEXT( VULKAN_HPP_NAMESPACE::DebugReportFlagsEXT flags, VULKAN_HPP_NAMESPACE::DebugReportObjectTypeEXT objectType, uint64_t object, size_t location, int32_t messageCode, const std::string & layerPrefix, const std::string & message, Dispatch const &d ) const
|
|
{
|
|
#ifdef VULKAN_HPP_NO_EXCEPTIONS
|
|
VULKAN_HPP_ASSERT( layerPrefix.size() == message.size() );
|
|
#else
|
|
if ( layerPrefix.size() != message.size() )
|
|
{
|
|
throw LogicError( VULKAN_HPP_NAMESPACE_STRING "::VkInstance::debugReportMessageEXT: layerPrefix.size() != message.size()" );
|
|
}
|
|
#endif /*VULKAN_HPP_NO_EXCEPTIONS*/
|
|
d.vkDebugReportMessageEXT( m_instance, static_cast<VkDebugReportFlagsEXT>( flags ), static_cast<VkDebugReportObjectTypeEXT>( objectType ), object, location, messageCode, layerPrefix.c_str(), message.c_str() );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::destroyDebugReportCallbackEXT( VULKAN_HPP_NAMESPACE::DebugReportCallbackEXT callback, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDestroyDebugReportCallbackEXT( m_instance, static_cast<VkDebugReportCallbackEXT>( callback ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::destroyDebugReportCallbackEXT( VULKAN_HPP_NAMESPACE::DebugReportCallbackEXT callback, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDestroyDebugReportCallbackEXT( m_instance, static_cast<VkDebugReportCallbackEXT>( callback ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ) );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::destroy( VULKAN_HPP_NAMESPACE::DebugReportCallbackEXT callback, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDestroyDebugReportCallbackEXT( m_instance, static_cast<VkDebugReportCallbackEXT>( callback ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::destroy( VULKAN_HPP_NAMESPACE::DebugReportCallbackEXT callback, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDestroyDebugReportCallbackEXT( m_instance, static_cast<VkDebugReportCallbackEXT>( callback ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ) );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::destroyDebugUtilsMessengerEXT( VULKAN_HPP_NAMESPACE::DebugUtilsMessengerEXT messenger, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDestroyDebugUtilsMessengerEXT( m_instance, static_cast<VkDebugUtilsMessengerEXT>( messenger ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::destroyDebugUtilsMessengerEXT( VULKAN_HPP_NAMESPACE::DebugUtilsMessengerEXT messenger, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDestroyDebugUtilsMessengerEXT( m_instance, static_cast<VkDebugUtilsMessengerEXT>( messenger ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ) );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::destroy( VULKAN_HPP_NAMESPACE::DebugUtilsMessengerEXT messenger, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDestroyDebugUtilsMessengerEXT( m_instance, static_cast<VkDebugUtilsMessengerEXT>( messenger ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::destroy( VULKAN_HPP_NAMESPACE::DebugUtilsMessengerEXT messenger, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDestroyDebugUtilsMessengerEXT( m_instance, static_cast<VkDebugUtilsMessengerEXT>( messenger ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ) );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::destroy( const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDestroyInstance( m_instance, reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::destroy( Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDestroyInstance( m_instance, reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ) );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::destroySurfaceKHR( VULKAN_HPP_NAMESPACE::SurfaceKHR surface, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDestroySurfaceKHR( m_instance, static_cast<VkSurfaceKHR>( surface ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::destroySurfaceKHR( VULKAN_HPP_NAMESPACE::SurfaceKHR surface, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDestroySurfaceKHR( m_instance, static_cast<VkSurfaceKHR>( surface ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ) );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::destroy( VULKAN_HPP_NAMESPACE::SurfaceKHR surface, const VULKAN_HPP_NAMESPACE::AllocationCallbacks* pAllocator, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDestroySurfaceKHR( m_instance, static_cast<VkSurfaceKHR>( surface ), reinterpret_cast<const VkAllocationCallbacks*>( pAllocator ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::destroy( VULKAN_HPP_NAMESPACE::SurfaceKHR surface, Optional<const AllocationCallbacks> allocator, Dispatch const &d ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkDestroySurfaceKHR( m_instance, static_cast<VkSurfaceKHR>( surface ), reinterpret_cast<const VkAllocationCallbacks*>( static_cast<const AllocationCallbacks*>( allocator ) ) );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::enumeratePhysicalDeviceGroups( uint32_t* pPhysicalDeviceGroupCount, VULKAN_HPP_NAMESPACE::PhysicalDeviceGroupProperties* pPhysicalDeviceGroupProperties, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkEnumeratePhysicalDeviceGroups( m_instance, pPhysicalDeviceGroupCount, reinterpret_cast<VkPhysicalDeviceGroupProperties*>( pPhysicalDeviceGroupProperties ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Allocator, typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<std::vector<PhysicalDeviceGroupProperties,Allocator>>::type Instance::enumeratePhysicalDeviceGroups(Dispatch const &d ) const
|
|
{
|
|
std::vector<PhysicalDeviceGroupProperties,Allocator> physicalDeviceGroupProperties;
|
|
uint32_t physicalDeviceGroupCount;
|
|
Result result;
|
|
do
|
|
{
|
|
result = static_cast<Result>( d.vkEnumeratePhysicalDeviceGroups( m_instance, &physicalDeviceGroupCount, nullptr ) );
|
|
if ( ( result == Result::eSuccess ) && physicalDeviceGroupCount )
|
|
{
|
|
physicalDeviceGroupProperties.resize( physicalDeviceGroupCount );
|
|
result = static_cast<Result>( d.vkEnumeratePhysicalDeviceGroups( m_instance, &physicalDeviceGroupCount, reinterpret_cast<VkPhysicalDeviceGroupProperties*>( physicalDeviceGroupProperties.data() ) ) );
|
|
}
|
|
} while ( result == Result::eIncomplete );
|
|
if ( result == Result::eSuccess )
|
|
{
|
|
VULKAN_HPP_ASSERT( physicalDeviceGroupCount <= physicalDeviceGroupProperties.size() );
|
|
physicalDeviceGroupProperties.resize( physicalDeviceGroupCount );
|
|
}
|
|
return createResultValue( result, physicalDeviceGroupProperties, VULKAN_HPP_NAMESPACE_STRING"::Instance::enumeratePhysicalDeviceGroups" );
|
|
}
|
|
template<typename Allocator, typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<std::vector<PhysicalDeviceGroupProperties,Allocator>>::type Instance::enumeratePhysicalDeviceGroups(Allocator const& vectorAllocator, Dispatch const &d ) const
|
|
{
|
|
std::vector<PhysicalDeviceGroupProperties,Allocator> physicalDeviceGroupProperties( vectorAllocator );
|
|
uint32_t physicalDeviceGroupCount;
|
|
Result result;
|
|
do
|
|
{
|
|
result = static_cast<Result>( d.vkEnumeratePhysicalDeviceGroups( m_instance, &physicalDeviceGroupCount, nullptr ) );
|
|
if ( ( result == Result::eSuccess ) && physicalDeviceGroupCount )
|
|
{
|
|
physicalDeviceGroupProperties.resize( physicalDeviceGroupCount );
|
|
result = static_cast<Result>( d.vkEnumeratePhysicalDeviceGroups( m_instance, &physicalDeviceGroupCount, reinterpret_cast<VkPhysicalDeviceGroupProperties*>( physicalDeviceGroupProperties.data() ) ) );
|
|
}
|
|
} while ( result == Result::eIncomplete );
|
|
if ( result == Result::eSuccess )
|
|
{
|
|
VULKAN_HPP_ASSERT( physicalDeviceGroupCount <= physicalDeviceGroupProperties.size() );
|
|
physicalDeviceGroupProperties.resize( physicalDeviceGroupCount );
|
|
}
|
|
return createResultValue( result, physicalDeviceGroupProperties, VULKAN_HPP_NAMESPACE_STRING"::Instance::enumeratePhysicalDeviceGroups" );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::enumeratePhysicalDeviceGroupsKHR( uint32_t* pPhysicalDeviceGroupCount, VULKAN_HPP_NAMESPACE::PhysicalDeviceGroupProperties* pPhysicalDeviceGroupProperties, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkEnumeratePhysicalDeviceGroups( m_instance, pPhysicalDeviceGroupCount, reinterpret_cast<VkPhysicalDeviceGroupProperties*>( pPhysicalDeviceGroupProperties ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Allocator, typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<std::vector<PhysicalDeviceGroupProperties,Allocator>>::type Instance::enumeratePhysicalDeviceGroupsKHR(Dispatch const &d ) const
|
|
{
|
|
std::vector<PhysicalDeviceGroupProperties,Allocator> physicalDeviceGroupProperties;
|
|
uint32_t physicalDeviceGroupCount;
|
|
Result result;
|
|
do
|
|
{
|
|
result = static_cast<Result>( d.vkEnumeratePhysicalDeviceGroups( m_instance, &physicalDeviceGroupCount, nullptr ) );
|
|
if ( ( result == Result::eSuccess ) && physicalDeviceGroupCount )
|
|
{
|
|
physicalDeviceGroupProperties.resize( physicalDeviceGroupCount );
|
|
result = static_cast<Result>( d.vkEnumeratePhysicalDeviceGroups( m_instance, &physicalDeviceGroupCount, reinterpret_cast<VkPhysicalDeviceGroupProperties*>( physicalDeviceGroupProperties.data() ) ) );
|
|
}
|
|
} while ( result == Result::eIncomplete );
|
|
if ( result == Result::eSuccess )
|
|
{
|
|
VULKAN_HPP_ASSERT( physicalDeviceGroupCount <= physicalDeviceGroupProperties.size() );
|
|
physicalDeviceGroupProperties.resize( physicalDeviceGroupCount );
|
|
}
|
|
return createResultValue( result, physicalDeviceGroupProperties, VULKAN_HPP_NAMESPACE_STRING"::Instance::enumeratePhysicalDeviceGroupsKHR" );
|
|
}
|
|
template<typename Allocator, typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<std::vector<PhysicalDeviceGroupProperties,Allocator>>::type Instance::enumeratePhysicalDeviceGroupsKHR(Allocator const& vectorAllocator, Dispatch const &d ) const
|
|
{
|
|
std::vector<PhysicalDeviceGroupProperties,Allocator> physicalDeviceGroupProperties( vectorAllocator );
|
|
uint32_t physicalDeviceGroupCount;
|
|
Result result;
|
|
do
|
|
{
|
|
result = static_cast<Result>( d.vkEnumeratePhysicalDeviceGroups( m_instance, &physicalDeviceGroupCount, nullptr ) );
|
|
if ( ( result == Result::eSuccess ) && physicalDeviceGroupCount )
|
|
{
|
|
physicalDeviceGroupProperties.resize( physicalDeviceGroupCount );
|
|
result = static_cast<Result>( d.vkEnumeratePhysicalDeviceGroups( m_instance, &physicalDeviceGroupCount, reinterpret_cast<VkPhysicalDeviceGroupProperties*>( physicalDeviceGroupProperties.data() ) ) );
|
|
}
|
|
} while ( result == Result::eIncomplete );
|
|
if ( result == Result::eSuccess )
|
|
{
|
|
VULKAN_HPP_ASSERT( physicalDeviceGroupCount <= physicalDeviceGroupProperties.size() );
|
|
physicalDeviceGroupProperties.resize( physicalDeviceGroupCount );
|
|
}
|
|
return createResultValue( result, physicalDeviceGroupProperties, VULKAN_HPP_NAMESPACE_STRING"::Instance::enumeratePhysicalDeviceGroupsKHR" );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE Result Instance::enumeratePhysicalDevices( uint32_t* pPhysicalDeviceCount, VULKAN_HPP_NAMESPACE::PhysicalDevice* pPhysicalDevices, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return static_cast<Result>( d.vkEnumeratePhysicalDevices( m_instance, pPhysicalDeviceCount, reinterpret_cast<VkPhysicalDevice*>( pPhysicalDevices ) ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Allocator, typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<std::vector<PhysicalDevice,Allocator>>::type Instance::enumeratePhysicalDevices(Dispatch const &d ) const
|
|
{
|
|
std::vector<PhysicalDevice,Allocator> physicalDevices;
|
|
uint32_t physicalDeviceCount;
|
|
Result result;
|
|
do
|
|
{
|
|
result = static_cast<Result>( d.vkEnumeratePhysicalDevices( m_instance, &physicalDeviceCount, nullptr ) );
|
|
if ( ( result == Result::eSuccess ) && physicalDeviceCount )
|
|
{
|
|
physicalDevices.resize( physicalDeviceCount );
|
|
result = static_cast<Result>( d.vkEnumeratePhysicalDevices( m_instance, &physicalDeviceCount, reinterpret_cast<VkPhysicalDevice*>( physicalDevices.data() ) ) );
|
|
}
|
|
} while ( result == Result::eIncomplete );
|
|
if ( result == Result::eSuccess )
|
|
{
|
|
VULKAN_HPP_ASSERT( physicalDeviceCount <= physicalDevices.size() );
|
|
physicalDevices.resize( physicalDeviceCount );
|
|
}
|
|
return createResultValue( result, physicalDevices, VULKAN_HPP_NAMESPACE_STRING"::Instance::enumeratePhysicalDevices" );
|
|
}
|
|
template<typename Allocator, typename Dispatch>
|
|
VULKAN_HPP_INLINE typename ResultValueType<std::vector<PhysicalDevice,Allocator>>::type Instance::enumeratePhysicalDevices(Allocator const& vectorAllocator, Dispatch const &d ) const
|
|
{
|
|
std::vector<PhysicalDevice,Allocator> physicalDevices( vectorAllocator );
|
|
uint32_t physicalDeviceCount;
|
|
Result result;
|
|
do
|
|
{
|
|
result = static_cast<Result>( d.vkEnumeratePhysicalDevices( m_instance, &physicalDeviceCount, nullptr ) );
|
|
if ( ( result == Result::eSuccess ) && physicalDeviceCount )
|
|
{
|
|
physicalDevices.resize( physicalDeviceCount );
|
|
result = static_cast<Result>( d.vkEnumeratePhysicalDevices( m_instance, &physicalDeviceCount, reinterpret_cast<VkPhysicalDevice*>( physicalDevices.data() ) ) );
|
|
}
|
|
} while ( result == Result::eIncomplete );
|
|
if ( result == Result::eSuccess )
|
|
{
|
|
VULKAN_HPP_ASSERT( physicalDeviceCount <= physicalDevices.size() );
|
|
physicalDevices.resize( physicalDeviceCount );
|
|
}
|
|
return createResultValue( result, physicalDevices, VULKAN_HPP_NAMESPACE_STRING"::Instance::enumeratePhysicalDevices" );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE PFN_vkVoidFunction Instance::getProcAddr( const char* pName, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return d.vkGetInstanceProcAddr( m_instance, pName );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE PFN_vkVoidFunction Instance::getProcAddr( const std::string & name, Dispatch const &d ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return d.vkGetInstanceProcAddr( m_instance, name.c_str() );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::submitDebugUtilsMessageEXT( VULKAN_HPP_NAMESPACE::DebugUtilsMessageSeverityFlagBitsEXT messageSeverity, VULKAN_HPP_NAMESPACE::DebugUtilsMessageTypeFlagsEXT messageTypes, const VULKAN_HPP_NAMESPACE::DebugUtilsMessengerCallbackDataEXT* pCallbackData, Dispatch const &d) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkSubmitDebugUtilsMessageEXT( m_instance, static_cast<VkDebugUtilsMessageSeverityFlagBitsEXT>( messageSeverity ), static_cast<VkDebugUtilsMessageTypeFlagsEXT>( messageTypes ), reinterpret_cast<const VkDebugUtilsMessengerCallbackDataEXT*>( pCallbackData ) );
|
|
}
|
|
#ifndef VULKAN_HPP_DISABLE_ENHANCED_MODE
|
|
template<typename Dispatch>
|
|
VULKAN_HPP_INLINE void Instance::submitDebugUtilsMessageEXT( VULKAN_HPP_NAMESPACE::DebugUtilsMessageSeverityFlagBitsEXT messageSeverity, VULKAN_HPP_NAMESPACE::DebugUtilsMessageTypeFlagsEXT messageTypes, const DebugUtilsMessengerCallbackDataEXT & callbackData, Dispatch const &d ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
d.vkSubmitDebugUtilsMessageEXT( m_instance, static_cast<VkDebugUtilsMessageSeverityFlagBitsEXT>( messageSeverity ), static_cast<VkDebugUtilsMessageTypeFlagsEXT>( messageTypes ), reinterpret_cast<const VkDebugUtilsMessengerCallbackDataEXT*>( &callbackData ) );
|
|
}
|
|
#endif /*VULKAN_HPP_DISABLE_ENHANCED_MODE*/
|
|
} // namespace VULKAN_HPP_NAMESPACE
|