mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-06-29 09:46:04 +02:00
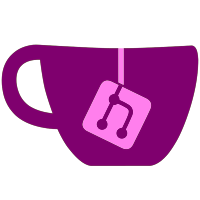
This commit adds Vulkan-Hpp as a library to the project. The headers are from a modified version of `VulkanHppGenerator`. They are broken into multiple files to avoid exceeding the Intellisense file size limit of Android Studio.
395 lines
12 KiB
C++
395 lines
12 KiB
C++
// Copyright (c) 2015-2019 The Khronos Group Inc.
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
//
|
|
// ---- Exceptions to the Apache 2.0 License: ----
|
|
//
|
|
// As an exception, if you use this Software to generate code and portions of
|
|
// this Software are embedded into the generated code as a result, you may
|
|
// redistribute such product without providing attribution as would otherwise
|
|
// be required by Sections 4(a), 4(b) and 4(d) of the License.
|
|
//
|
|
// In addition, if you combine or link code generated by this Software with
|
|
// software that is licensed under the GPLv2 or the LGPL v2.0 or 2.1
|
|
// ("`Combined Software`") and if a court of competent jurisdiction determines
|
|
// that the patent provision (Section 3), the indemnity provision (Section 9)
|
|
// or other Section of the License conflicts with the conditions of the
|
|
// applicable GPL or LGPL license, you may retroactively and prospectively
|
|
// choose to deem waived or otherwise exclude such Section(s) of the License,
|
|
// but only in their entirety and only with respect to the Combined Software.
|
|
//
|
|
|
|
// This header is generated from the Khronos Vulkan XML API Registry.
|
|
|
|
#pragma once
|
|
|
|
#include "../handles.hpp"
|
|
#include "VkAcquire.hpp"
|
|
#include "VkAcceleration.hpp"
|
|
#include "VkApplication.hpp"
|
|
#include "VkAllocation.hpp"
|
|
#include "VkBind.hpp"
|
|
#include "VkAndroid.hpp"
|
|
#include "VkBase.hpp"
|
|
#include "VkAttachment.hpp"
|
|
#include "VkBuffer.hpp"
|
|
#include "VkCalibrated.hpp"
|
|
#include "VkCheckpoint.hpp"
|
|
#include "VkClear.hpp"
|
|
|
|
namespace VULKAN_HPP_NAMESPACE
|
|
{
|
|
union ClearColorValue
|
|
{
|
|
ClearColorValue( VULKAN_HPP_NAMESPACE::ClearColorValue const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( static_cast<void*>(this), &rhs, sizeof( VULKAN_HPP_NAMESPACE::ClearColorValue ) );
|
|
}
|
|
|
|
ClearColorValue( const std::array<float,4>& float32_ = {} )
|
|
{
|
|
memcpy( float32, float32_.data(), 4 * sizeof( float ) );
|
|
}
|
|
|
|
ClearColorValue( const std::array<int32_t,4>& int32_ )
|
|
{
|
|
memcpy( int32, int32_.data(), 4 * sizeof( int32_t ) );
|
|
}
|
|
|
|
ClearColorValue( const std::array<uint32_t,4>& uint32_ )
|
|
{
|
|
memcpy( uint32, uint32_.data(), 4 * sizeof( uint32_t ) );
|
|
}
|
|
|
|
ClearColorValue & setFloat32( std::array<float,4> float32_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( float32, float32_.data(), 4 * sizeof( float ) );
|
|
return *this;
|
|
}
|
|
|
|
ClearColorValue & setInt32( std::array<int32_t,4> int32_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( int32, int32_.data(), 4 * sizeof( int32_t ) );
|
|
return *this;
|
|
}
|
|
|
|
ClearColorValue & setUint32( std::array<uint32_t,4> uint32_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( uint32, uint32_.data(), 4 * sizeof( uint32_t ) );
|
|
return *this;
|
|
}
|
|
|
|
VULKAN_HPP_NAMESPACE::ClearColorValue & operator=( VULKAN_HPP_NAMESPACE::ClearColorValue const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( static_cast<void*>(this), &rhs, sizeof( VULKAN_HPP_NAMESPACE::ClearColorValue ) );
|
|
return *this;
|
|
}
|
|
|
|
operator VkClearColorValue const&() const
|
|
{
|
|
return *reinterpret_cast<const VkClearColorValue*>(this);
|
|
}
|
|
|
|
operator VkClearColorValue &()
|
|
{
|
|
return *reinterpret_cast<VkClearColorValue*>(this);
|
|
}
|
|
|
|
float float32[4];
|
|
int32_t int32[4];
|
|
uint32_t uint32[4];
|
|
};
|
|
|
|
struct ClearDepthStencilValue
|
|
{
|
|
VULKAN_HPP_CONSTEXPR ClearDepthStencilValue( float depth_ = {},
|
|
uint32_t stencil_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: depth( depth_ )
|
|
, stencil( stencil_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR ClearDepthStencilValue( ClearDepthStencilValue const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: depth( rhs.depth )
|
|
, stencil( rhs.stencil )
|
|
{}
|
|
|
|
ClearDepthStencilValue & operator=( ClearDepthStencilValue const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( static_cast<void*>(this), &rhs, sizeof( ClearDepthStencilValue ) );
|
|
return *this;
|
|
}
|
|
|
|
ClearDepthStencilValue( VkClearDepthStencilValue const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
ClearDepthStencilValue& operator=( VkClearDepthStencilValue const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::ClearDepthStencilValue const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
ClearDepthStencilValue & setDepth( float depth_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
depth = depth_;
|
|
return *this;
|
|
}
|
|
|
|
ClearDepthStencilValue & setStencil( uint32_t stencil_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
stencil = stencil_;
|
|
return *this;
|
|
}
|
|
|
|
operator VkClearDepthStencilValue const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkClearDepthStencilValue*>( this );
|
|
}
|
|
|
|
operator VkClearDepthStencilValue &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkClearDepthStencilValue*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( ClearDepthStencilValue const& ) const = default;
|
|
#else
|
|
bool operator==( ClearDepthStencilValue const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( depth == rhs.depth )
|
|
&& ( stencil == rhs.stencil );
|
|
}
|
|
|
|
bool operator!=( ClearDepthStencilValue const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
float depth = {};
|
|
uint32_t stencil = {};
|
|
};
|
|
static_assert( sizeof( ClearDepthStencilValue ) == sizeof( VkClearDepthStencilValue ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<ClearDepthStencilValue>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
union ClearValue
|
|
{
|
|
ClearValue( VULKAN_HPP_NAMESPACE::ClearValue const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( static_cast<void*>(this), &rhs, sizeof( VULKAN_HPP_NAMESPACE::ClearValue ) );
|
|
}
|
|
|
|
ClearValue( VULKAN_HPP_NAMESPACE::ClearColorValue color_ = {} )
|
|
{
|
|
color = color_;
|
|
}
|
|
|
|
ClearValue( VULKAN_HPP_NAMESPACE::ClearDepthStencilValue depthStencil_ )
|
|
{
|
|
depthStencil = depthStencil_;
|
|
}
|
|
|
|
ClearValue & setColor( VULKAN_HPP_NAMESPACE::ClearColorValue color_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
color = color_;
|
|
return *this;
|
|
}
|
|
|
|
ClearValue & setDepthStencil( VULKAN_HPP_NAMESPACE::ClearDepthStencilValue depthStencil_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
depthStencil = depthStencil_;
|
|
return *this;
|
|
}
|
|
|
|
VULKAN_HPP_NAMESPACE::ClearValue & operator=( VULKAN_HPP_NAMESPACE::ClearValue const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( static_cast<void*>(this), &rhs, sizeof( VULKAN_HPP_NAMESPACE::ClearValue ) );
|
|
return *this;
|
|
}
|
|
|
|
operator VkClearValue const&() const
|
|
{
|
|
return *reinterpret_cast<const VkClearValue*>(this);
|
|
}
|
|
|
|
operator VkClearValue &()
|
|
{
|
|
return *reinterpret_cast<VkClearValue*>(this);
|
|
}
|
|
|
|
#ifdef VULKAN_HPP_HAS_UNRESTRICTED_UNIONS
|
|
VULKAN_HPP_NAMESPACE::ClearColorValue color;
|
|
VULKAN_HPP_NAMESPACE::ClearDepthStencilValue depthStencil;
|
|
#else
|
|
VkClearColorValue color;
|
|
VkClearDepthStencilValue depthStencil;
|
|
#endif /*VULKAN_HPP_HAS_UNRESTRICTED_UNIONS*/
|
|
};
|
|
|
|
struct ClearAttachment
|
|
{
|
|
ClearAttachment( VULKAN_HPP_NAMESPACE::ImageAspectFlags aspectMask_ = {},
|
|
uint32_t colorAttachment_ = {},
|
|
VULKAN_HPP_NAMESPACE::ClearValue clearValue_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: aspectMask( aspectMask_ )
|
|
, colorAttachment( colorAttachment_ )
|
|
, clearValue( clearValue_ )
|
|
{}
|
|
|
|
ClearAttachment( ClearAttachment const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: aspectMask( rhs.aspectMask )
|
|
, colorAttachment( rhs.colorAttachment )
|
|
, clearValue( rhs.clearValue )
|
|
{}
|
|
|
|
ClearAttachment & operator=( ClearAttachment const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( static_cast<void*>(this), &rhs, sizeof( ClearAttachment ) );
|
|
return *this;
|
|
}
|
|
|
|
ClearAttachment( VkClearAttachment const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
ClearAttachment& operator=( VkClearAttachment const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::ClearAttachment const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
ClearAttachment & setAspectMask( VULKAN_HPP_NAMESPACE::ImageAspectFlags aspectMask_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
aspectMask = aspectMask_;
|
|
return *this;
|
|
}
|
|
|
|
ClearAttachment & setColorAttachment( uint32_t colorAttachment_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
colorAttachment = colorAttachment_;
|
|
return *this;
|
|
}
|
|
|
|
ClearAttachment & setClearValue( VULKAN_HPP_NAMESPACE::ClearValue clearValue_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
clearValue = clearValue_;
|
|
return *this;
|
|
}
|
|
|
|
operator VkClearAttachment const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkClearAttachment*>( this );
|
|
}
|
|
|
|
operator VkClearAttachment &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkClearAttachment*>( this );
|
|
}
|
|
|
|
public:
|
|
VULKAN_HPP_NAMESPACE::ImageAspectFlags aspectMask = {};
|
|
uint32_t colorAttachment = {};
|
|
VULKAN_HPP_NAMESPACE::ClearValue clearValue = {};
|
|
};
|
|
static_assert( sizeof( ClearAttachment ) == sizeof( VkClearAttachment ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<ClearAttachment>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
struct ClearRect
|
|
{
|
|
VULKAN_HPP_CONSTEXPR ClearRect( VULKAN_HPP_NAMESPACE::Rect2D rect_ = {},
|
|
uint32_t baseArrayLayer_ = {},
|
|
uint32_t layerCount_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: rect( rect_ )
|
|
, baseArrayLayer( baseArrayLayer_ )
|
|
, layerCount( layerCount_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR ClearRect( ClearRect const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: rect( rhs.rect )
|
|
, baseArrayLayer( rhs.baseArrayLayer )
|
|
, layerCount( rhs.layerCount )
|
|
{}
|
|
|
|
ClearRect & operator=( ClearRect const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( static_cast<void*>(this), &rhs, sizeof( ClearRect ) );
|
|
return *this;
|
|
}
|
|
|
|
ClearRect( VkClearRect const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
ClearRect& operator=( VkClearRect const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::ClearRect const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
ClearRect & setRect( VULKAN_HPP_NAMESPACE::Rect2D rect_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
rect = rect_;
|
|
return *this;
|
|
}
|
|
|
|
ClearRect & setBaseArrayLayer( uint32_t baseArrayLayer_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
baseArrayLayer = baseArrayLayer_;
|
|
return *this;
|
|
}
|
|
|
|
ClearRect & setLayerCount( uint32_t layerCount_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
layerCount = layerCount_;
|
|
return *this;
|
|
}
|
|
|
|
operator VkClearRect const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkClearRect*>( this );
|
|
}
|
|
|
|
operator VkClearRect &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkClearRect*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( ClearRect const& ) const = default;
|
|
#else
|
|
bool operator==( ClearRect const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( rect == rhs.rect )
|
|
&& ( baseArrayLayer == rhs.baseArrayLayer )
|
|
&& ( layerCount == rhs.layerCount );
|
|
}
|
|
|
|
bool operator!=( ClearRect const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
VULKAN_HPP_NAMESPACE::Rect2D rect = {};
|
|
uint32_t baseArrayLayer = {};
|
|
uint32_t layerCount = {};
|
|
};
|
|
static_assert( sizeof( ClearRect ) == sizeof( VkClearRect ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<ClearRect>::value, "struct wrapper is not a standard layout!" );
|
|
} // namespace VULKAN_HPP_NAMESPACE
|