mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-06-29 09:46:04 +02:00
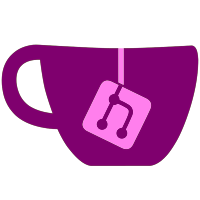
This commit adds Vulkan-Hpp as a library to the project. The headers are from a modified version of `VulkanHppGenerator`. They are broken into multiple files to avoid exceeding the Intellisense file size limit of Android Studio.
1264 lines
43 KiB
C++
1264 lines
43 KiB
C++
// Copyright (c) 2015-2019 The Khronos Group Inc.
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
//
|
|
// ---- Exceptions to the Apache 2.0 License: ----
|
|
//
|
|
// As an exception, if you use this Software to generate code and portions of
|
|
// this Software are embedded into the generated code as a result, you may
|
|
// redistribute such product without providing attribution as would otherwise
|
|
// be required by Sections 4(a), 4(b) and 4(d) of the License.
|
|
//
|
|
// In addition, if you combine or link code generated by this Software with
|
|
// software that is licensed under the GPLv2 or the LGPL v2.0 or 2.1
|
|
// ("`Combined Software`") and if a court of competent jurisdiction determines
|
|
// that the patent provision (Section 3), the indemnity provision (Section 9)
|
|
// or other Section of the License conflicts with the conditions of the
|
|
// applicable GPL or LGPL license, you may retroactively and prospectively
|
|
// choose to deem waived or otherwise exclude such Section(s) of the License,
|
|
// but only in their entirety and only with respect to the Combined Software.
|
|
//
|
|
|
|
// This header is generated from the Khronos Vulkan XML API Registry.
|
|
|
|
#pragma once
|
|
|
|
#include "../handles.hpp"
|
|
#include "VkAcquire.hpp"
|
|
#include "VkAcceleration.hpp"
|
|
#include "VkApplication.hpp"
|
|
#include "VkInitialize.hpp"
|
|
#include "VkAllocation.hpp"
|
|
#include "VkExternal.hpp"
|
|
#include "VkBind.hpp"
|
|
#include "VkCooperative.hpp"
|
|
#include "VkAndroid.hpp"
|
|
#include "VkImport.hpp"
|
|
#include "VkImage.hpp"
|
|
#include "VkDescriptor.hpp"
|
|
#include "VkBase.hpp"
|
|
#include "VkAttachment.hpp"
|
|
#include "VkBuffer.hpp"
|
|
#include "VkFramebuffer.hpp"
|
|
#include "VkCalibrated.hpp"
|
|
#include "VkDevice.hpp"
|
|
#include "VkCheckpoint.hpp"
|
|
#include "VkConformance.hpp"
|
|
#include "VkClear.hpp"
|
|
#include "VkCmd.hpp"
|
|
#include "VkExtension.hpp"
|
|
#include "VkCoarse.hpp"
|
|
#include "VkCommand.hpp"
|
|
#include "VkFormat.hpp"
|
|
#include "VkComponent.hpp"
|
|
#include "VkCopy.hpp"
|
|
#include "VkCompute.hpp"
|
|
#include "VkConditional.hpp"
|
|
#include "VkMapped.hpp"
|
|
#include "VkD3D.hpp"
|
|
#include "VkDebug.hpp"
|
|
#include "VkFence.hpp"
|
|
#include "VkDedicated.hpp"
|
|
#include "VkDraw.hpp"
|
|
#include "VkDispatch.hpp"
|
|
#include "VkDisplay.hpp"
|
|
#include "VkDrm.hpp"
|
|
#include "VkEvent.hpp"
|
|
#include "VkExport.hpp"
|
|
#include "VkExtent.hpp"
|
|
#include "VkFilter.hpp"
|
|
#include "VkGeometry.hpp"
|
|
#include "VkGraphics.hpp"
|
|
#include "VkHdr.hpp"
|
|
#include "VkHeadless.hpp"
|
|
#include "VkI.hpp"
|
|
#include "VkIndirect.hpp"
|
|
#include "VkInput.hpp"
|
|
#include "VkInstance.hpp"
|
|
#include "VkLayer.hpp"
|
|
#include "VkMac.hpp"
|
|
#include "VkMemory.hpp"
|
|
|
|
namespace VULKAN_HPP_NAMESPACE
|
|
{
|
|
struct MemoryAllocateFlagsInfo
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryAllocateFlagsInfo( VULKAN_HPP_NAMESPACE::MemoryAllocateFlags flags_ = {},
|
|
uint32_t deviceMask_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: flags( flags_ )
|
|
, deviceMask( deviceMask_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryAllocateFlagsInfo( MemoryAllocateFlagsInfo const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: pNext( rhs.pNext )
|
|
, flags( rhs.flags )
|
|
, deviceMask( rhs.deviceMask )
|
|
{}
|
|
|
|
MemoryAllocateFlagsInfo & operator=( MemoryAllocateFlagsInfo const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( &pNext, &rhs.pNext, sizeof( MemoryAllocateFlagsInfo ) - offsetof( MemoryAllocateFlagsInfo, pNext ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryAllocateFlagsInfo( VkMemoryAllocateFlagsInfo const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryAllocateFlagsInfo& operator=( VkMemoryAllocateFlagsInfo const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryAllocateFlagsInfo const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
MemoryAllocateFlagsInfo & setPNext( const void* pNext_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
pNext = pNext_;
|
|
return *this;
|
|
}
|
|
|
|
MemoryAllocateFlagsInfo & setFlags( VULKAN_HPP_NAMESPACE::MemoryAllocateFlags flags_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
flags = flags_;
|
|
return *this;
|
|
}
|
|
|
|
MemoryAllocateFlagsInfo & setDeviceMask( uint32_t deviceMask_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
deviceMask = deviceMask_;
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryAllocateFlagsInfo const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryAllocateFlagsInfo*>( this );
|
|
}
|
|
|
|
operator VkMemoryAllocateFlagsInfo &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryAllocateFlagsInfo*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryAllocateFlagsInfo const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryAllocateFlagsInfo const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( sType == rhs.sType )
|
|
&& ( pNext == rhs.pNext )
|
|
&& ( flags == rhs.flags )
|
|
&& ( deviceMask == rhs.deviceMask );
|
|
}
|
|
|
|
bool operator!=( MemoryAllocateFlagsInfo const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
const VULKAN_HPP_NAMESPACE::StructureType sType = StructureType::eMemoryAllocateFlagsInfo;
|
|
const void* pNext = {};
|
|
VULKAN_HPP_NAMESPACE::MemoryAllocateFlags flags = {};
|
|
uint32_t deviceMask = {};
|
|
};
|
|
static_assert( sizeof( MemoryAllocateFlagsInfo ) == sizeof( VkMemoryAllocateFlagsInfo ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryAllocateFlagsInfo>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
struct MemoryAllocateInfo
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryAllocateInfo( VULKAN_HPP_NAMESPACE::DeviceSize allocationSize_ = {},
|
|
uint32_t memoryTypeIndex_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: allocationSize( allocationSize_ )
|
|
, memoryTypeIndex( memoryTypeIndex_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryAllocateInfo( MemoryAllocateInfo const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: pNext( rhs.pNext )
|
|
, allocationSize( rhs.allocationSize )
|
|
, memoryTypeIndex( rhs.memoryTypeIndex )
|
|
{}
|
|
|
|
MemoryAllocateInfo & operator=( MemoryAllocateInfo const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( &pNext, &rhs.pNext, sizeof( MemoryAllocateInfo ) - offsetof( MemoryAllocateInfo, pNext ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryAllocateInfo( VkMemoryAllocateInfo const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryAllocateInfo& operator=( VkMemoryAllocateInfo const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryAllocateInfo const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
MemoryAllocateInfo & setPNext( const void* pNext_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
pNext = pNext_;
|
|
return *this;
|
|
}
|
|
|
|
MemoryAllocateInfo & setAllocationSize( VULKAN_HPP_NAMESPACE::DeviceSize allocationSize_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
allocationSize = allocationSize_;
|
|
return *this;
|
|
}
|
|
|
|
MemoryAllocateInfo & setMemoryTypeIndex( uint32_t memoryTypeIndex_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memoryTypeIndex = memoryTypeIndex_;
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryAllocateInfo const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryAllocateInfo*>( this );
|
|
}
|
|
|
|
operator VkMemoryAllocateInfo &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryAllocateInfo*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryAllocateInfo const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryAllocateInfo const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( sType == rhs.sType )
|
|
&& ( pNext == rhs.pNext )
|
|
&& ( allocationSize == rhs.allocationSize )
|
|
&& ( memoryTypeIndex == rhs.memoryTypeIndex );
|
|
}
|
|
|
|
bool operator!=( MemoryAllocateInfo const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
const VULKAN_HPP_NAMESPACE::StructureType sType = StructureType::eMemoryAllocateInfo;
|
|
const void* pNext = {};
|
|
VULKAN_HPP_NAMESPACE::DeviceSize allocationSize = {};
|
|
uint32_t memoryTypeIndex = {};
|
|
};
|
|
static_assert( sizeof( MemoryAllocateInfo ) == sizeof( VkMemoryAllocateInfo ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryAllocateInfo>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
struct MemoryBarrier
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryBarrier( VULKAN_HPP_NAMESPACE::AccessFlags srcAccessMask_ = {},
|
|
VULKAN_HPP_NAMESPACE::AccessFlags dstAccessMask_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: srcAccessMask( srcAccessMask_ )
|
|
, dstAccessMask( dstAccessMask_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryBarrier( MemoryBarrier const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: pNext( rhs.pNext )
|
|
, srcAccessMask( rhs.srcAccessMask )
|
|
, dstAccessMask( rhs.dstAccessMask )
|
|
{}
|
|
|
|
MemoryBarrier & operator=( MemoryBarrier const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( &pNext, &rhs.pNext, sizeof( MemoryBarrier ) - offsetof( MemoryBarrier, pNext ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryBarrier( VkMemoryBarrier const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryBarrier& operator=( VkMemoryBarrier const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryBarrier const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
MemoryBarrier & setPNext( const void* pNext_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
pNext = pNext_;
|
|
return *this;
|
|
}
|
|
|
|
MemoryBarrier & setSrcAccessMask( VULKAN_HPP_NAMESPACE::AccessFlags srcAccessMask_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
srcAccessMask = srcAccessMask_;
|
|
return *this;
|
|
}
|
|
|
|
MemoryBarrier & setDstAccessMask( VULKAN_HPP_NAMESPACE::AccessFlags dstAccessMask_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
dstAccessMask = dstAccessMask_;
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryBarrier const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryBarrier*>( this );
|
|
}
|
|
|
|
operator VkMemoryBarrier &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryBarrier*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryBarrier const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryBarrier const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( sType == rhs.sType )
|
|
&& ( pNext == rhs.pNext )
|
|
&& ( srcAccessMask == rhs.srcAccessMask )
|
|
&& ( dstAccessMask == rhs.dstAccessMask );
|
|
}
|
|
|
|
bool operator!=( MemoryBarrier const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
const VULKAN_HPP_NAMESPACE::StructureType sType = StructureType::eMemoryBarrier;
|
|
const void* pNext = {};
|
|
VULKAN_HPP_NAMESPACE::AccessFlags srcAccessMask = {};
|
|
VULKAN_HPP_NAMESPACE::AccessFlags dstAccessMask = {};
|
|
};
|
|
static_assert( sizeof( MemoryBarrier ) == sizeof( VkMemoryBarrier ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryBarrier>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
struct MemoryDedicatedAllocateInfo
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryDedicatedAllocateInfo( VULKAN_HPP_NAMESPACE::Image image_ = {},
|
|
VULKAN_HPP_NAMESPACE::Buffer buffer_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: image( image_ )
|
|
, buffer( buffer_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryDedicatedAllocateInfo( MemoryDedicatedAllocateInfo const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: pNext( rhs.pNext )
|
|
, image( rhs.image )
|
|
, buffer( rhs.buffer )
|
|
{}
|
|
|
|
MemoryDedicatedAllocateInfo & operator=( MemoryDedicatedAllocateInfo const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( &pNext, &rhs.pNext, sizeof( MemoryDedicatedAllocateInfo ) - offsetof( MemoryDedicatedAllocateInfo, pNext ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryDedicatedAllocateInfo( VkMemoryDedicatedAllocateInfo const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryDedicatedAllocateInfo& operator=( VkMemoryDedicatedAllocateInfo const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryDedicatedAllocateInfo const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
MemoryDedicatedAllocateInfo & setPNext( const void* pNext_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
pNext = pNext_;
|
|
return *this;
|
|
}
|
|
|
|
MemoryDedicatedAllocateInfo & setImage( VULKAN_HPP_NAMESPACE::Image image_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
image = image_;
|
|
return *this;
|
|
}
|
|
|
|
MemoryDedicatedAllocateInfo & setBuffer( VULKAN_HPP_NAMESPACE::Buffer buffer_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
buffer = buffer_;
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryDedicatedAllocateInfo const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryDedicatedAllocateInfo*>( this );
|
|
}
|
|
|
|
operator VkMemoryDedicatedAllocateInfo &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryDedicatedAllocateInfo*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryDedicatedAllocateInfo const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryDedicatedAllocateInfo const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( sType == rhs.sType )
|
|
&& ( pNext == rhs.pNext )
|
|
&& ( image == rhs.image )
|
|
&& ( buffer == rhs.buffer );
|
|
}
|
|
|
|
bool operator!=( MemoryDedicatedAllocateInfo const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
const VULKAN_HPP_NAMESPACE::StructureType sType = StructureType::eMemoryDedicatedAllocateInfo;
|
|
const void* pNext = {};
|
|
VULKAN_HPP_NAMESPACE::Image image = {};
|
|
VULKAN_HPP_NAMESPACE::Buffer buffer = {};
|
|
};
|
|
static_assert( sizeof( MemoryDedicatedAllocateInfo ) == sizeof( VkMemoryDedicatedAllocateInfo ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryDedicatedAllocateInfo>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
struct MemoryDedicatedRequirements
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryDedicatedRequirements( VULKAN_HPP_NAMESPACE::Bool32 prefersDedicatedAllocation_ = {},
|
|
VULKAN_HPP_NAMESPACE::Bool32 requiresDedicatedAllocation_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: prefersDedicatedAllocation( prefersDedicatedAllocation_ )
|
|
, requiresDedicatedAllocation( requiresDedicatedAllocation_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryDedicatedRequirements( MemoryDedicatedRequirements const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: pNext( rhs.pNext )
|
|
, prefersDedicatedAllocation( rhs.prefersDedicatedAllocation )
|
|
, requiresDedicatedAllocation( rhs.requiresDedicatedAllocation )
|
|
{}
|
|
|
|
MemoryDedicatedRequirements & operator=( MemoryDedicatedRequirements const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( &pNext, &rhs.pNext, sizeof( MemoryDedicatedRequirements ) - offsetof( MemoryDedicatedRequirements, pNext ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryDedicatedRequirements( VkMemoryDedicatedRequirements const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryDedicatedRequirements& operator=( VkMemoryDedicatedRequirements const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryDedicatedRequirements const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryDedicatedRequirements const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryDedicatedRequirements*>( this );
|
|
}
|
|
|
|
operator VkMemoryDedicatedRequirements &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryDedicatedRequirements*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryDedicatedRequirements const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryDedicatedRequirements const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( sType == rhs.sType )
|
|
&& ( pNext == rhs.pNext )
|
|
&& ( prefersDedicatedAllocation == rhs.prefersDedicatedAllocation )
|
|
&& ( requiresDedicatedAllocation == rhs.requiresDedicatedAllocation );
|
|
}
|
|
|
|
bool operator!=( MemoryDedicatedRequirements const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
const VULKAN_HPP_NAMESPACE::StructureType sType = StructureType::eMemoryDedicatedRequirements;
|
|
void* pNext = {};
|
|
VULKAN_HPP_NAMESPACE::Bool32 prefersDedicatedAllocation = {};
|
|
VULKAN_HPP_NAMESPACE::Bool32 requiresDedicatedAllocation = {};
|
|
};
|
|
static_assert( sizeof( MemoryDedicatedRequirements ) == sizeof( VkMemoryDedicatedRequirements ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryDedicatedRequirements>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
struct MemoryFdPropertiesKHR
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryFdPropertiesKHR( uint32_t memoryTypeBits_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: memoryTypeBits( memoryTypeBits_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryFdPropertiesKHR( MemoryFdPropertiesKHR const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: pNext( rhs.pNext )
|
|
, memoryTypeBits( rhs.memoryTypeBits )
|
|
{}
|
|
|
|
MemoryFdPropertiesKHR & operator=( MemoryFdPropertiesKHR const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( &pNext, &rhs.pNext, sizeof( MemoryFdPropertiesKHR ) - offsetof( MemoryFdPropertiesKHR, pNext ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryFdPropertiesKHR( VkMemoryFdPropertiesKHR const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryFdPropertiesKHR& operator=( VkMemoryFdPropertiesKHR const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryFdPropertiesKHR const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryFdPropertiesKHR const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryFdPropertiesKHR*>( this );
|
|
}
|
|
|
|
operator VkMemoryFdPropertiesKHR &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryFdPropertiesKHR*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryFdPropertiesKHR const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryFdPropertiesKHR const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( sType == rhs.sType )
|
|
&& ( pNext == rhs.pNext )
|
|
&& ( memoryTypeBits == rhs.memoryTypeBits );
|
|
}
|
|
|
|
bool operator!=( MemoryFdPropertiesKHR const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
const VULKAN_HPP_NAMESPACE::StructureType sType = StructureType::eMemoryFdPropertiesKHR;
|
|
void* pNext = {};
|
|
uint32_t memoryTypeBits = {};
|
|
};
|
|
static_assert( sizeof( MemoryFdPropertiesKHR ) == sizeof( VkMemoryFdPropertiesKHR ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryFdPropertiesKHR>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
#ifdef VK_USE_PLATFORM_ANDROID_KHR
|
|
struct MemoryGetAndroidHardwareBufferInfoANDROID
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryGetAndroidHardwareBufferInfoANDROID( VULKAN_HPP_NAMESPACE::DeviceMemory memory_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: memory( memory_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryGetAndroidHardwareBufferInfoANDROID( MemoryGetAndroidHardwareBufferInfoANDROID const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: pNext( rhs.pNext )
|
|
, memory( rhs.memory )
|
|
{}
|
|
|
|
MemoryGetAndroidHardwareBufferInfoANDROID & operator=( MemoryGetAndroidHardwareBufferInfoANDROID const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( &pNext, &rhs.pNext, sizeof( MemoryGetAndroidHardwareBufferInfoANDROID ) - offsetof( MemoryGetAndroidHardwareBufferInfoANDROID, pNext ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryGetAndroidHardwareBufferInfoANDROID( VkMemoryGetAndroidHardwareBufferInfoANDROID const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryGetAndroidHardwareBufferInfoANDROID& operator=( VkMemoryGetAndroidHardwareBufferInfoANDROID const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryGetAndroidHardwareBufferInfoANDROID const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
MemoryGetAndroidHardwareBufferInfoANDROID & setPNext( const void* pNext_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
pNext = pNext_;
|
|
return *this;
|
|
}
|
|
|
|
MemoryGetAndroidHardwareBufferInfoANDROID & setMemory( VULKAN_HPP_NAMESPACE::DeviceMemory memory_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memory = memory_;
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryGetAndroidHardwareBufferInfoANDROID const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryGetAndroidHardwareBufferInfoANDROID*>( this );
|
|
}
|
|
|
|
operator VkMemoryGetAndroidHardwareBufferInfoANDROID &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryGetAndroidHardwareBufferInfoANDROID*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryGetAndroidHardwareBufferInfoANDROID const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryGetAndroidHardwareBufferInfoANDROID const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( sType == rhs.sType )
|
|
&& ( pNext == rhs.pNext )
|
|
&& ( memory == rhs.memory );
|
|
}
|
|
|
|
bool operator!=( MemoryGetAndroidHardwareBufferInfoANDROID const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
const VULKAN_HPP_NAMESPACE::StructureType sType = StructureType::eMemoryGetAndroidHardwareBufferInfoANDROID;
|
|
const void* pNext = {};
|
|
VULKAN_HPP_NAMESPACE::DeviceMemory memory = {};
|
|
};
|
|
static_assert( sizeof( MemoryGetAndroidHardwareBufferInfoANDROID ) == sizeof( VkMemoryGetAndroidHardwareBufferInfoANDROID ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryGetAndroidHardwareBufferInfoANDROID>::value, "struct wrapper is not a standard layout!" );
|
|
#endif /*VK_USE_PLATFORM_ANDROID_KHR*/
|
|
|
|
struct MemoryGetFdInfoKHR
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryGetFdInfoKHR( VULKAN_HPP_NAMESPACE::DeviceMemory memory_ = {},
|
|
VULKAN_HPP_NAMESPACE::ExternalMemoryHandleTypeFlagBits handleType_ = VULKAN_HPP_NAMESPACE::ExternalMemoryHandleTypeFlagBits::eOpaqueFd ) VULKAN_HPP_NOEXCEPT
|
|
: memory( memory_ )
|
|
, handleType( handleType_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryGetFdInfoKHR( MemoryGetFdInfoKHR const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: pNext( rhs.pNext )
|
|
, memory( rhs.memory )
|
|
, handleType( rhs.handleType )
|
|
{}
|
|
|
|
MemoryGetFdInfoKHR & operator=( MemoryGetFdInfoKHR const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( &pNext, &rhs.pNext, sizeof( MemoryGetFdInfoKHR ) - offsetof( MemoryGetFdInfoKHR, pNext ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryGetFdInfoKHR( VkMemoryGetFdInfoKHR const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryGetFdInfoKHR& operator=( VkMemoryGetFdInfoKHR const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryGetFdInfoKHR const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
MemoryGetFdInfoKHR & setPNext( const void* pNext_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
pNext = pNext_;
|
|
return *this;
|
|
}
|
|
|
|
MemoryGetFdInfoKHR & setMemory( VULKAN_HPP_NAMESPACE::DeviceMemory memory_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memory = memory_;
|
|
return *this;
|
|
}
|
|
|
|
MemoryGetFdInfoKHR & setHandleType( VULKAN_HPP_NAMESPACE::ExternalMemoryHandleTypeFlagBits handleType_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
handleType = handleType_;
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryGetFdInfoKHR const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryGetFdInfoKHR*>( this );
|
|
}
|
|
|
|
operator VkMemoryGetFdInfoKHR &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryGetFdInfoKHR*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryGetFdInfoKHR const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryGetFdInfoKHR const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( sType == rhs.sType )
|
|
&& ( pNext == rhs.pNext )
|
|
&& ( memory == rhs.memory )
|
|
&& ( handleType == rhs.handleType );
|
|
}
|
|
|
|
bool operator!=( MemoryGetFdInfoKHR const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
const VULKAN_HPP_NAMESPACE::StructureType sType = StructureType::eMemoryGetFdInfoKHR;
|
|
const void* pNext = {};
|
|
VULKAN_HPP_NAMESPACE::DeviceMemory memory = {};
|
|
VULKAN_HPP_NAMESPACE::ExternalMemoryHandleTypeFlagBits handleType = VULKAN_HPP_NAMESPACE::ExternalMemoryHandleTypeFlagBits::eOpaqueFd;
|
|
};
|
|
static_assert( sizeof( MemoryGetFdInfoKHR ) == sizeof( VkMemoryGetFdInfoKHR ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryGetFdInfoKHR>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
#ifdef VK_USE_PLATFORM_WIN32_KHR
|
|
struct MemoryGetWin32HandleInfoKHR
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryGetWin32HandleInfoKHR( VULKAN_HPP_NAMESPACE::DeviceMemory memory_ = {},
|
|
VULKAN_HPP_NAMESPACE::ExternalMemoryHandleTypeFlagBits handleType_ = VULKAN_HPP_NAMESPACE::ExternalMemoryHandleTypeFlagBits::eOpaqueFd ) VULKAN_HPP_NOEXCEPT
|
|
: memory( memory_ )
|
|
, handleType( handleType_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryGetWin32HandleInfoKHR( MemoryGetWin32HandleInfoKHR const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: pNext( rhs.pNext )
|
|
, memory( rhs.memory )
|
|
, handleType( rhs.handleType )
|
|
{}
|
|
|
|
MemoryGetWin32HandleInfoKHR & operator=( MemoryGetWin32HandleInfoKHR const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( &pNext, &rhs.pNext, sizeof( MemoryGetWin32HandleInfoKHR ) - offsetof( MemoryGetWin32HandleInfoKHR, pNext ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryGetWin32HandleInfoKHR( VkMemoryGetWin32HandleInfoKHR const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryGetWin32HandleInfoKHR& operator=( VkMemoryGetWin32HandleInfoKHR const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryGetWin32HandleInfoKHR const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
MemoryGetWin32HandleInfoKHR & setPNext( const void* pNext_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
pNext = pNext_;
|
|
return *this;
|
|
}
|
|
|
|
MemoryGetWin32HandleInfoKHR & setMemory( VULKAN_HPP_NAMESPACE::DeviceMemory memory_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memory = memory_;
|
|
return *this;
|
|
}
|
|
|
|
MemoryGetWin32HandleInfoKHR & setHandleType( VULKAN_HPP_NAMESPACE::ExternalMemoryHandleTypeFlagBits handleType_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
handleType = handleType_;
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryGetWin32HandleInfoKHR const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryGetWin32HandleInfoKHR*>( this );
|
|
}
|
|
|
|
operator VkMemoryGetWin32HandleInfoKHR &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryGetWin32HandleInfoKHR*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryGetWin32HandleInfoKHR const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryGetWin32HandleInfoKHR const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( sType == rhs.sType )
|
|
&& ( pNext == rhs.pNext )
|
|
&& ( memory == rhs.memory )
|
|
&& ( handleType == rhs.handleType );
|
|
}
|
|
|
|
bool operator!=( MemoryGetWin32HandleInfoKHR const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
const VULKAN_HPP_NAMESPACE::StructureType sType = StructureType::eMemoryGetWin32HandleInfoKHR;
|
|
const void* pNext = {};
|
|
VULKAN_HPP_NAMESPACE::DeviceMemory memory = {};
|
|
VULKAN_HPP_NAMESPACE::ExternalMemoryHandleTypeFlagBits handleType = VULKAN_HPP_NAMESPACE::ExternalMemoryHandleTypeFlagBits::eOpaqueFd;
|
|
};
|
|
static_assert( sizeof( MemoryGetWin32HandleInfoKHR ) == sizeof( VkMemoryGetWin32HandleInfoKHR ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryGetWin32HandleInfoKHR>::value, "struct wrapper is not a standard layout!" );
|
|
#endif /*VK_USE_PLATFORM_WIN32_KHR*/
|
|
|
|
struct MemoryHeap
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryHeap( VULKAN_HPP_NAMESPACE::DeviceSize size_ = {},
|
|
VULKAN_HPP_NAMESPACE::MemoryHeapFlags flags_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: size( size_ )
|
|
, flags( flags_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryHeap( MemoryHeap const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: size( rhs.size )
|
|
, flags( rhs.flags )
|
|
{}
|
|
|
|
MemoryHeap & operator=( MemoryHeap const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( static_cast<void*>(this), &rhs, sizeof( MemoryHeap ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryHeap( VkMemoryHeap const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryHeap& operator=( VkMemoryHeap const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryHeap const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryHeap const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryHeap*>( this );
|
|
}
|
|
|
|
operator VkMemoryHeap &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryHeap*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryHeap const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryHeap const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( size == rhs.size )
|
|
&& ( flags == rhs.flags );
|
|
}
|
|
|
|
bool operator!=( MemoryHeap const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
VULKAN_HPP_NAMESPACE::DeviceSize size = {};
|
|
VULKAN_HPP_NAMESPACE::MemoryHeapFlags flags = {};
|
|
};
|
|
static_assert( sizeof( MemoryHeap ) == sizeof( VkMemoryHeap ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryHeap>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
struct MemoryHostPointerPropertiesEXT
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryHostPointerPropertiesEXT( uint32_t memoryTypeBits_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: memoryTypeBits( memoryTypeBits_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryHostPointerPropertiesEXT( MemoryHostPointerPropertiesEXT const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: pNext( rhs.pNext )
|
|
, memoryTypeBits( rhs.memoryTypeBits )
|
|
{}
|
|
|
|
MemoryHostPointerPropertiesEXT & operator=( MemoryHostPointerPropertiesEXT const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( &pNext, &rhs.pNext, sizeof( MemoryHostPointerPropertiesEXT ) - offsetof( MemoryHostPointerPropertiesEXT, pNext ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryHostPointerPropertiesEXT( VkMemoryHostPointerPropertiesEXT const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryHostPointerPropertiesEXT& operator=( VkMemoryHostPointerPropertiesEXT const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryHostPointerPropertiesEXT const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryHostPointerPropertiesEXT const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryHostPointerPropertiesEXT*>( this );
|
|
}
|
|
|
|
operator VkMemoryHostPointerPropertiesEXT &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryHostPointerPropertiesEXT*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryHostPointerPropertiesEXT const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryHostPointerPropertiesEXT const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( sType == rhs.sType )
|
|
&& ( pNext == rhs.pNext )
|
|
&& ( memoryTypeBits == rhs.memoryTypeBits );
|
|
}
|
|
|
|
bool operator!=( MemoryHostPointerPropertiesEXT const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
const VULKAN_HPP_NAMESPACE::StructureType sType = StructureType::eMemoryHostPointerPropertiesEXT;
|
|
void* pNext = {};
|
|
uint32_t memoryTypeBits = {};
|
|
};
|
|
static_assert( sizeof( MemoryHostPointerPropertiesEXT ) == sizeof( VkMemoryHostPointerPropertiesEXT ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryHostPointerPropertiesEXT>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
struct MemoryPriorityAllocateInfoEXT
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryPriorityAllocateInfoEXT( float priority_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: priority( priority_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryPriorityAllocateInfoEXT( MemoryPriorityAllocateInfoEXT const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: pNext( rhs.pNext )
|
|
, priority( rhs.priority )
|
|
{}
|
|
|
|
MemoryPriorityAllocateInfoEXT & operator=( MemoryPriorityAllocateInfoEXT const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( &pNext, &rhs.pNext, sizeof( MemoryPriorityAllocateInfoEXT ) - offsetof( MemoryPriorityAllocateInfoEXT, pNext ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryPriorityAllocateInfoEXT( VkMemoryPriorityAllocateInfoEXT const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryPriorityAllocateInfoEXT& operator=( VkMemoryPriorityAllocateInfoEXT const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryPriorityAllocateInfoEXT const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
MemoryPriorityAllocateInfoEXT & setPNext( const void* pNext_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
pNext = pNext_;
|
|
return *this;
|
|
}
|
|
|
|
MemoryPriorityAllocateInfoEXT & setPriority( float priority_ ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
priority = priority_;
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryPriorityAllocateInfoEXT const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryPriorityAllocateInfoEXT*>( this );
|
|
}
|
|
|
|
operator VkMemoryPriorityAllocateInfoEXT &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryPriorityAllocateInfoEXT*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryPriorityAllocateInfoEXT const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryPriorityAllocateInfoEXT const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( sType == rhs.sType )
|
|
&& ( pNext == rhs.pNext )
|
|
&& ( priority == rhs.priority );
|
|
}
|
|
|
|
bool operator!=( MemoryPriorityAllocateInfoEXT const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
const VULKAN_HPP_NAMESPACE::StructureType sType = StructureType::eMemoryPriorityAllocateInfoEXT;
|
|
const void* pNext = {};
|
|
float priority = {};
|
|
};
|
|
static_assert( sizeof( MemoryPriorityAllocateInfoEXT ) == sizeof( VkMemoryPriorityAllocateInfoEXT ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryPriorityAllocateInfoEXT>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
struct MemoryRequirements
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryRequirements( VULKAN_HPP_NAMESPACE::DeviceSize size_ = {},
|
|
VULKAN_HPP_NAMESPACE::DeviceSize alignment_ = {},
|
|
uint32_t memoryTypeBits_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: size( size_ )
|
|
, alignment( alignment_ )
|
|
, memoryTypeBits( memoryTypeBits_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryRequirements( MemoryRequirements const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: size( rhs.size )
|
|
, alignment( rhs.alignment )
|
|
, memoryTypeBits( rhs.memoryTypeBits )
|
|
{}
|
|
|
|
MemoryRequirements & operator=( MemoryRequirements const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( static_cast<void*>(this), &rhs, sizeof( MemoryRequirements ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryRequirements( VkMemoryRequirements const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryRequirements& operator=( VkMemoryRequirements const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryRequirements const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryRequirements const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryRequirements*>( this );
|
|
}
|
|
|
|
operator VkMemoryRequirements &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryRequirements*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryRequirements const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryRequirements const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( size == rhs.size )
|
|
&& ( alignment == rhs.alignment )
|
|
&& ( memoryTypeBits == rhs.memoryTypeBits );
|
|
}
|
|
|
|
bool operator!=( MemoryRequirements const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
VULKAN_HPP_NAMESPACE::DeviceSize size = {};
|
|
VULKAN_HPP_NAMESPACE::DeviceSize alignment = {};
|
|
uint32_t memoryTypeBits = {};
|
|
};
|
|
static_assert( sizeof( MemoryRequirements ) == sizeof( VkMemoryRequirements ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryRequirements>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
struct MemoryRequirements2
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryRequirements2( VULKAN_HPP_NAMESPACE::MemoryRequirements memoryRequirements_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: memoryRequirements( memoryRequirements_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryRequirements2( MemoryRequirements2 const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: pNext( rhs.pNext )
|
|
, memoryRequirements( rhs.memoryRequirements )
|
|
{}
|
|
|
|
MemoryRequirements2 & operator=( MemoryRequirements2 const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( &pNext, &rhs.pNext, sizeof( MemoryRequirements2 ) - offsetof( MemoryRequirements2, pNext ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryRequirements2( VkMemoryRequirements2 const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryRequirements2& operator=( VkMemoryRequirements2 const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryRequirements2 const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryRequirements2 const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryRequirements2*>( this );
|
|
}
|
|
|
|
operator VkMemoryRequirements2 &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryRequirements2*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryRequirements2 const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryRequirements2 const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( sType == rhs.sType )
|
|
&& ( pNext == rhs.pNext )
|
|
&& ( memoryRequirements == rhs.memoryRequirements );
|
|
}
|
|
|
|
bool operator!=( MemoryRequirements2 const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
const VULKAN_HPP_NAMESPACE::StructureType sType = StructureType::eMemoryRequirements2;
|
|
void* pNext = {};
|
|
VULKAN_HPP_NAMESPACE::MemoryRequirements memoryRequirements = {};
|
|
};
|
|
static_assert( sizeof( MemoryRequirements2 ) == sizeof( VkMemoryRequirements2 ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryRequirements2>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
struct MemoryType
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryType( VULKAN_HPP_NAMESPACE::MemoryPropertyFlags propertyFlags_ = {},
|
|
uint32_t heapIndex_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: propertyFlags( propertyFlags_ )
|
|
, heapIndex( heapIndex_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryType( MemoryType const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: propertyFlags( rhs.propertyFlags )
|
|
, heapIndex( rhs.heapIndex )
|
|
{}
|
|
|
|
MemoryType & operator=( MemoryType const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( static_cast<void*>(this), &rhs, sizeof( MemoryType ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryType( VkMemoryType const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryType& operator=( VkMemoryType const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryType const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryType const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryType*>( this );
|
|
}
|
|
|
|
operator VkMemoryType &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryType*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryType const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryType const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( propertyFlags == rhs.propertyFlags )
|
|
&& ( heapIndex == rhs.heapIndex );
|
|
}
|
|
|
|
bool operator!=( MemoryType const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
VULKAN_HPP_NAMESPACE::MemoryPropertyFlags propertyFlags = {};
|
|
uint32_t heapIndex = {};
|
|
};
|
|
static_assert( sizeof( MemoryType ) == sizeof( VkMemoryType ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryType>::value, "struct wrapper is not a standard layout!" );
|
|
|
|
#ifdef VK_USE_PLATFORM_WIN32_KHR
|
|
struct MemoryWin32HandlePropertiesKHR
|
|
{
|
|
VULKAN_HPP_CONSTEXPR MemoryWin32HandlePropertiesKHR( uint32_t memoryTypeBits_ = {} ) VULKAN_HPP_NOEXCEPT
|
|
: memoryTypeBits( memoryTypeBits_ )
|
|
{}
|
|
|
|
VULKAN_HPP_CONSTEXPR MemoryWin32HandlePropertiesKHR( MemoryWin32HandlePropertiesKHR const& rhs ) VULKAN_HPP_NOEXCEPT
|
|
: pNext( rhs.pNext )
|
|
, memoryTypeBits( rhs.memoryTypeBits )
|
|
{}
|
|
|
|
MemoryWin32HandlePropertiesKHR & operator=( MemoryWin32HandlePropertiesKHR const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
memcpy( &pNext, &rhs.pNext, sizeof( MemoryWin32HandlePropertiesKHR ) - offsetof( MemoryWin32HandlePropertiesKHR, pNext ) );
|
|
return *this;
|
|
}
|
|
|
|
MemoryWin32HandlePropertiesKHR( VkMemoryWin32HandlePropertiesKHR const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = rhs;
|
|
}
|
|
|
|
MemoryWin32HandlePropertiesKHR& operator=( VkMemoryWin32HandlePropertiesKHR const & rhs ) VULKAN_HPP_NOEXCEPT
|
|
{
|
|
*this = *reinterpret_cast<VULKAN_HPP_NAMESPACE::MemoryWin32HandlePropertiesKHR const *>(&rhs);
|
|
return *this;
|
|
}
|
|
|
|
operator VkMemoryWin32HandlePropertiesKHR const&() const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<const VkMemoryWin32HandlePropertiesKHR*>( this );
|
|
}
|
|
|
|
operator VkMemoryWin32HandlePropertiesKHR &() VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return *reinterpret_cast<VkMemoryWin32HandlePropertiesKHR*>( this );
|
|
}
|
|
|
|
#if defined(VULKAN_HPP_HAS_SPACESHIP_OPERATOR)
|
|
auto operator<=>( MemoryWin32HandlePropertiesKHR const& ) const = default;
|
|
#else
|
|
bool operator==( MemoryWin32HandlePropertiesKHR const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return ( sType == rhs.sType )
|
|
&& ( pNext == rhs.pNext )
|
|
&& ( memoryTypeBits == rhs.memoryTypeBits );
|
|
}
|
|
|
|
bool operator!=( MemoryWin32HandlePropertiesKHR const& rhs ) const VULKAN_HPP_NOEXCEPT
|
|
{
|
|
return !operator==( rhs );
|
|
}
|
|
#endif
|
|
|
|
public:
|
|
const VULKAN_HPP_NAMESPACE::StructureType sType = StructureType::eMemoryWin32HandlePropertiesKHR;
|
|
void* pNext = {};
|
|
uint32_t memoryTypeBits = {};
|
|
};
|
|
static_assert( sizeof( MemoryWin32HandlePropertiesKHR ) == sizeof( VkMemoryWin32HandlePropertiesKHR ), "struct and wrapper have different size!" );
|
|
static_assert( std::is_standard_layout<MemoryWin32HandlePropertiesKHR>::value, "struct wrapper is not a standard layout!" );
|
|
#endif /*VK_USE_PLATFORM_WIN32_KHR*/
|
|
} // namespace VULKAN_HPP_NAMESPACE
|