mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-06-01 20:58:44 +02:00
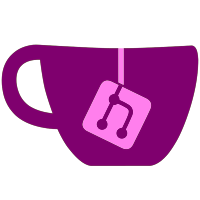
We've moved from using an AAR for Mbed TLS to a submodule as the AAR was packaged manually and used from a local repository which ended up being very hacky and resulted in Linter errors, it could also not be updated with ease as it would need to be repackaged. All of these issues have been solved by moving to a git submodule tied to the official Mbed TLS GitHub repository.
134 lines
3.6 KiB
Groovy
134 lines
3.6 KiB
Groovy
plugins {
|
|
id 'com.android.application'
|
|
id 'kotlin-android'
|
|
id 'kotlin-kapt'
|
|
id 'dagger.hilt.android.plugin'
|
|
}
|
|
|
|
android {
|
|
compileSdkVersion 30
|
|
buildToolsVersion '30.0.3'
|
|
defaultConfig {
|
|
applicationId "skyline.emu"
|
|
|
|
minSdkVersion 29
|
|
targetSdkVersion 30
|
|
|
|
versionCode 3
|
|
versionName "0.0.3"
|
|
|
|
ndk {
|
|
abiFilters "arm64-v8a"
|
|
}
|
|
}
|
|
|
|
/* JVM Bytecode Options */
|
|
def javaVersion = JavaVersion.VERSION_1_8
|
|
compileOptions {
|
|
sourceCompatibility = javaVersion
|
|
targetCompatibility = javaVersion
|
|
}
|
|
kotlinOptions {
|
|
jvmTarget = javaVersion.toString()
|
|
}
|
|
|
|
buildTypes {
|
|
release {
|
|
debuggable true
|
|
externalNativeBuild {
|
|
cmake {
|
|
arguments "-DCMAKE_BUILD_TYPE=RELEASE"
|
|
}
|
|
}
|
|
minifyEnabled true
|
|
shrinkResources true
|
|
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
|
|
signingConfig signingConfigs.debug
|
|
}
|
|
|
|
debug {
|
|
debuggable true
|
|
minifyEnabled false
|
|
shrinkResources false
|
|
}
|
|
}
|
|
buildFeatures {
|
|
prefab true
|
|
viewBinding true
|
|
}
|
|
|
|
/* Linting */
|
|
lintOptions {
|
|
disable 'IconLocation'
|
|
}
|
|
|
|
/* NDK */
|
|
ndkVersion '22.1.7171670'
|
|
externalNativeBuild {
|
|
cmake {
|
|
version '3.18.1+'
|
|
path "CMakeLists.txt"
|
|
}
|
|
}
|
|
|
|
/* Vulkan Validation Layers */
|
|
sourceSets {
|
|
debug {
|
|
main {
|
|
jniLibs {
|
|
srcDir "$buildDir/generated/vulkan_layers"
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
/* Android Assets */
|
|
aaptOptions {
|
|
ignoreAssetsPattern "*.md"
|
|
}
|
|
}
|
|
|
|
/**
|
|
* We just want VK_LAYER_KHRONOS_validation in the APK while NDK contains several other legacy layers
|
|
* This task copies shared objects associated with that layer into a folder from where JNI can use it
|
|
*/
|
|
task setupValidationLayer(type: Copy) {
|
|
doFirst {
|
|
def folder = new File("$buildDir/generated/vulkan_layers")
|
|
if (!folder.exists()) {
|
|
folder.mkdirs() // We need to recursively create all directories as the copy requires the output directory to exist
|
|
}
|
|
}
|
|
from("${android.ndkDirectory}/sources/third_party/vulkan/src/build-android/jniLibs") {
|
|
include "*/libVkLayer_khronos_validation.so"
|
|
}
|
|
into "$buildDir/generated/vulkan_layers"
|
|
}
|
|
|
|
afterEvaluate {
|
|
preDebugBuild.dependsOn setupValidationLayer
|
|
}
|
|
|
|
dependencies {
|
|
/* Google */
|
|
implementation "androidx.core:core-ktx:1.6.0"
|
|
implementation 'androidx.appcompat:appcompat:1.3.0'
|
|
implementation 'androidx.constraintlayout:constraintlayout:2.0.4'
|
|
implementation 'androidx.preference:preference-ktx:1.1.1'
|
|
implementation 'com.google.android.material:material:1.4.0'
|
|
implementation 'androidx.documentfile:documentfile:1.0.1'
|
|
implementation 'androidx.swiperefreshlayout:swiperefreshlayout:1.1.0'
|
|
implementation "androidx.lifecycle:lifecycle-viewmodel-ktx:$lifecycle_version"
|
|
implementation "androidx.lifecycle:lifecycle-livedata-ktx:$lifecycle_version"
|
|
implementation 'androidx.fragment:fragment-ktx:1.3.5'
|
|
implementation "com.google.dagger:hilt-android:$hilt_version"
|
|
kapt "com.google.dagger:hilt-android-compiler:$hilt_version"
|
|
implementation 'com.google.android:flexbox:2.0.1'
|
|
|
|
/* Kotlin */
|
|
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk8:$kotlin_version"
|
|
|
|
/* Other Java */
|
|
implementation 'info.debatty:java-string-similarity:2.0.0'
|
|
}
|