mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-06-01 15:39:02 +02:00
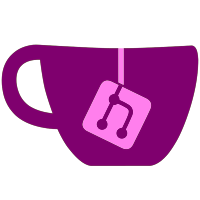
This commit adds the Services API and implements some services. It also changes the name of the application to Skyline and replaces the icon.
52 lines
1.1 KiB
C++
52 lines
1.1 KiB
C++
#pragma once
|
|
|
|
#include <cstdint>
|
|
#include "loader.h"
|
|
|
|
namespace skyline::loader {
|
|
class NroLoader : public Loader {
|
|
private:
|
|
/**
|
|
* @brief The structure of a single Segment descriptor in the NRO's header
|
|
*/
|
|
struct NroSegmentHeader {
|
|
u32 offset;
|
|
u32 size;
|
|
};
|
|
|
|
/**
|
|
* @brief A bit-field struct to read the header of an NRO directly
|
|
*/
|
|
struct NroHeader {
|
|
u32 : 32;
|
|
u32 mod_offset;
|
|
u64 : 64;
|
|
|
|
u32 magic;
|
|
u32 version;
|
|
u32 size;
|
|
u32 flags;
|
|
|
|
NroSegmentHeader text;
|
|
NroSegmentHeader ro;
|
|
NroSegmentHeader data;
|
|
|
|
u32 bssSize;
|
|
u32 : 32;
|
|
u64 build_id[4];
|
|
u64 : 64;
|
|
|
|
NroSegmentHeader api_info;
|
|
NroSegmentHeader dynstr;
|
|
NroSegmentHeader dynsym;
|
|
};
|
|
|
|
public:
|
|
/**
|
|
* @param filePath The path to the ROM file
|
|
* @param state The state of the device
|
|
*/
|
|
NroLoader(std::string filePath, const DeviceState &state);
|
|
};
|
|
}
|