mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-11-17 20:29:17 +01:00
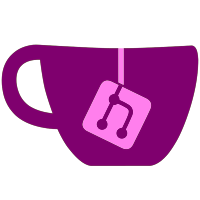
* Fix handling `SA_EXPOSE_TAGBITS` bit being set in Android 12 `sigaction` * Fix CMake bug using `CMAKE_INTERPROCEDURAL_OPTIMIZATION_RELEASE` when not supported causing `-fuse-ld=gold` to be emitted as a linker flag * Support using `VIBRATOR_MANAGER_SERVICE` rather than `VIBRATOR_SERVICE` on Android 12 * Optimize Imports for Kotlin code * Move away from deprecated APIs in Kotlin or explicitly mark where it's not possible * Update SDK, NDK and libraries * Enable Gradle Configuration Cache
39 lines
1.6 KiB
Kotlin
39 lines
1.6 KiB
Kotlin
package emu.skyline
|
|
|
|
import android.annotation.SuppressLint
|
|
import android.content.Context
|
|
import android.net.Uri
|
|
import androidx.documentfile.provider.DocumentFile
|
|
import dagger.hilt.android.qualifiers.ApplicationContext
|
|
import emu.skyline.loader.AppEntry
|
|
import emu.skyline.loader.RomFile
|
|
import emu.skyline.loader.RomFormat
|
|
import emu.skyline.loader.RomFormat.*
|
|
import javax.inject.Inject
|
|
import javax.inject.Singleton
|
|
|
|
@Singleton
|
|
class RomProvider @Inject constructor(@ApplicationContext private val context : Context) {
|
|
/**
|
|
* This adds all files in [directory] with [extension] as an entry using [RomFile] to load metadata
|
|
*/
|
|
@SuppressLint("DefaultLocale")
|
|
private fun addEntries(fileFormats : Map<String, RomFormat>, directory : DocumentFile, entries : HashMap<RomFormat, ArrayList<AppEntry>>, systemLanguage : Int) {
|
|
directory.listFiles().forEach { file ->
|
|
if (file.isDirectory) {
|
|
addEntries(fileFormats, file, entries, systemLanguage)
|
|
} else {
|
|
fileFormats[file.name?.substringAfterLast(".")?.lowercase()]?.let { romFormat->
|
|
entries.getOrPut(romFormat, { arrayListOf() }).add(RomFile(context, romFormat, file.uri, systemLanguage).appEntry)
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
fun loadRoms(searchLocation : Uri, systemLanguage : Int) = DocumentFile.fromTreeUri(context, searchLocation)!!.let { documentFile ->
|
|
hashMapOf<RomFormat, ArrayList<AppEntry>>().apply {
|
|
addEntries(mapOf("nro" to NRO, "nso" to NSO, "nca" to NCA, "nsp" to NSP, "xci" to XCI), documentFile, this, systemLanguage)
|
|
}
|
|
}
|
|
}
|