mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-06-09 06:18:44 +02:00
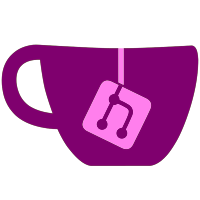
We've moved to using a beta AGP as `7.0.2` is breaks `clangd` and other C++ features on Beta/Canary Android Studio. NDK was additionally updated with `mbedtls` to fix warnings caused by it alongside some other minor fixes to code for newer versions of libcxx. The new AGP has a bug where it does not look for executables specified in `android_gradle_build.json` in `PATH` that includes `ninja` which is provided by the `ninja-build` package on the system rather than Android SDK's CMake on GitHub Actions (Ubuntu 20.04). This has been fixed by symlinking `/usr/bin/ninja` to the project root which is searched in for the `ninja` executable.
37 lines
1.4 KiB
C++
37 lines
1.4 KiB
C++
// SPDX-License-Identifier: MPL-2.0
|
|
// Copyright © 2020 Skyline Team and Contributors (https://github.com/skyline-emu/)
|
|
|
|
#include "nacp.h"
|
|
|
|
namespace skyline::vfs {
|
|
NACP::NACP(const std::shared_ptr<vfs::Backing> &backing) {
|
|
nacpContents = backing->Read<NacpData>();
|
|
|
|
u32 counter{};
|
|
for (auto &entry : nacpContents.titleEntries) {
|
|
if (entry.applicationName.front() != '\0') {
|
|
supportedTitleLanguages |= (1 << counter);
|
|
}
|
|
counter++;
|
|
}
|
|
}
|
|
|
|
language::ApplicationLanguage NACP::GetFirstSupportedTitleLanguage() {
|
|
return static_cast<language::ApplicationLanguage>(std::countr_zero(supportedTitleLanguages));
|
|
}
|
|
|
|
language::ApplicationLanguage NACP::GetFirstSupportedLanguage() {
|
|
return static_cast<language::ApplicationLanguage>(std::countr_zero(nacpContents.supportedLanguageFlag));
|
|
}
|
|
|
|
std::string NACP::GetApplicationName(language::ApplicationLanguage language) {
|
|
auto applicationName{span(nacpContents.titleEntries.at(static_cast<size_t>(language)).applicationName)};
|
|
return std::string(applicationName.as_string(true));
|
|
}
|
|
|
|
std::string NACP::GetApplicationPublisher(language::ApplicationLanguage language) {
|
|
auto applicationPublisher{span(nacpContents.titleEntries.at(static_cast<size_t>(language)).applicationPublisher)};
|
|
return std::string(applicationPublisher.as_string(true));
|
|
}
|
|
}
|