mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-11-06 00:45:09 +01:00
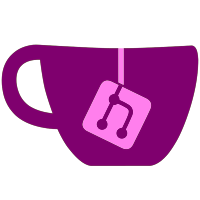
Extends the profile picture stub into a full-fledged implementation with the ability for users to set their profile picture in settings while having the Skyline icon as the default profile picture.
181 lines
5.0 KiB
Groovy
181 lines
5.0 KiB
Groovy
plugins {
|
|
id 'com.android.application'
|
|
id 'kotlin-android'
|
|
id 'kotlin-kapt'
|
|
id 'dagger.hilt.android.plugin'
|
|
id 'idea'
|
|
id 'org.jetbrains.kotlin.plugin.serialization' version "$kotlin_version"
|
|
}
|
|
|
|
idea.module {
|
|
// These are not viable to index on most systems so exclude them to prevent IDE crashes
|
|
excludeDirs.add(file("libraries/boost"))
|
|
excludeDirs.add(file("libraries/llvm"))
|
|
}
|
|
|
|
android {
|
|
namespace 'emu.skyline'
|
|
|
|
var isBuildSigned = (System.getenv("CI") == "true") && (System.getenv("IS_SKYLINE_SIGNED") == "true")
|
|
|
|
compileSdkVersion 33
|
|
buildToolsVersion '33.0.0'
|
|
defaultConfig {
|
|
applicationId "skyline.emu"
|
|
|
|
minSdkVersion 29
|
|
targetSdkVersion 33
|
|
|
|
versionCode 3
|
|
versionName "0.0.3"
|
|
|
|
ndk {
|
|
abiFilters "arm64-v8a"
|
|
}
|
|
|
|
if (isBuildSigned)
|
|
manifestPlaceholders = [shouldSaveUserData: "true"]
|
|
else
|
|
manifestPlaceholders = [shouldSaveUserData: "false"]
|
|
}
|
|
|
|
/* JVM Bytecode Options */
|
|
def javaVersion = JavaVersion.VERSION_1_8
|
|
compileOptions {
|
|
sourceCompatibility = javaVersion
|
|
targetCompatibility = javaVersion
|
|
}
|
|
kotlinOptions {
|
|
jvmTarget = javaVersion.toString()
|
|
freeCompilerArgs += "-opt-in=kotlin.RequiresOptIn"
|
|
}
|
|
|
|
signingConfigs {
|
|
ci {
|
|
storeFile file(System.getenv("SIGNING_STORE_PATH") ?: "${System.getenv("user.home")}/keystore.jks")
|
|
storePassword System.getenv("SIGNING_STORE_PASSWORD")
|
|
keyAlias System.getenv("SIGNING_KEY_ALIAS")
|
|
keyPassword System.getenv("SIGNING_KEY_PASSWORD")
|
|
}
|
|
}
|
|
|
|
buildTypes {
|
|
release {
|
|
debuggable true
|
|
externalNativeBuild {
|
|
cmake {
|
|
arguments "-DCMAKE_BUILD_TYPE=RELEASE"
|
|
}
|
|
}
|
|
minifyEnabled true
|
|
shrinkResources true
|
|
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
|
|
signingConfig = isBuildSigned ? signingConfigs.ci : signingConfigs.debug
|
|
}
|
|
|
|
reldebug {
|
|
debuggable true
|
|
externalNativeBuild {
|
|
cmake {
|
|
arguments "-DCMAKE_BUILD_TYPE=RELWITHDEBINFO"
|
|
}
|
|
}
|
|
minifyEnabled false
|
|
shrinkResources false
|
|
signingConfig = isBuildSigned ? signingConfigs.ci : signingConfigs.debug
|
|
}
|
|
|
|
debug {
|
|
debuggable true
|
|
minifyEnabled false
|
|
shrinkResources false
|
|
signingConfig = isBuildSigned ? signingConfigs.ci : signingConfigs.debug
|
|
}
|
|
}
|
|
|
|
flavorDimensions += "version"
|
|
productFlavors {
|
|
full {
|
|
dimension = "version"
|
|
manifestPlaceholders = [appLabel: "Skyline"]
|
|
}
|
|
|
|
dev {
|
|
dimension = "version"
|
|
applicationIdSuffix = ".dev"
|
|
versionNameSuffix = "-dev"
|
|
manifestPlaceholders = [appLabel: "Skyline Dev"]
|
|
}
|
|
}
|
|
|
|
buildFeatures {
|
|
viewBinding true
|
|
}
|
|
|
|
/* Linting */
|
|
lint {
|
|
disable 'IconLocation'
|
|
}
|
|
|
|
/* NDK and CMake */
|
|
ndkVersion '25.0.8775105'
|
|
externalNativeBuild {
|
|
cmake {
|
|
version '3.22.1+'
|
|
path "CMakeLists.txt"
|
|
}
|
|
}
|
|
|
|
/* Android Assets */
|
|
androidResources {
|
|
ignoreAssetsPattern '*.md'
|
|
}
|
|
|
|
/* Vulkan Validation Layers */
|
|
sourceSets {
|
|
reldebug {
|
|
jniLibs {
|
|
srcDir "libraries/vklayers"
|
|
}
|
|
}
|
|
|
|
debug {
|
|
jniLibs {
|
|
srcDir "libraries/vklayers"
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
dependencies {
|
|
/* Google */
|
|
implementation 'androidx.core:core-ktx:1.8.0'
|
|
implementation 'androidx.appcompat:appcompat:1.5.0'
|
|
implementation 'androidx.constraintlayout:constraintlayout:2.1.4'
|
|
implementation 'androidx.preference:preference-ktx:1.2.0'
|
|
implementation 'androidx.activity:activity-ktx:1.6.1'
|
|
implementation 'com.google.android.material:material:1.6.1'
|
|
implementation 'androidx.documentfile:documentfile:1.0.1'
|
|
implementation 'androidx.swiperefreshlayout:swiperefreshlayout:1.1.0'
|
|
implementation "androidx.lifecycle:lifecycle-viewmodel-ktx:$lifecycle_version"
|
|
implementation "androidx.lifecycle:lifecycle-livedata-ktx:$lifecycle_version"
|
|
implementation 'androidx.fragment:fragment-ktx:1.5.2'
|
|
implementation "com.google.dagger:hilt-android:$hilt_version"
|
|
kapt "com.google.dagger:hilt-android-compiler:$hilt_version"
|
|
implementation 'com.google.android.flexbox:flexbox:3.0.0'
|
|
|
|
/* Kotlin */
|
|
implementation "org.jetbrains.kotlin:kotlin-reflect:$kotlin_version"
|
|
implementation "org.jetbrains.kotlinx:kotlinx-serialization-json:$serialization_version"
|
|
|
|
/* JetBrains */
|
|
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk8:$kotlin_version"
|
|
|
|
/* Other Java */
|
|
implementation 'info.debatty:java-string-similarity:2.0.0'
|
|
}
|
|
|
|
kapt {
|
|
correctErrorTypes true
|
|
}
|