mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-06-01 21:08:42 +02:00
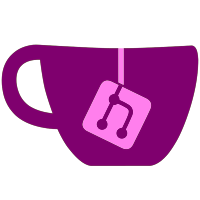
* Fix `AddClearColorSubpass` bug where it would not generate a `VkCmdNextSubpass` when an attachment clear was utilized * Fix `AddSubpass` bug where the Depth Stencil texture would not be synced * Respect `VkCommandPool` external synchronization requirements by making it thread-local with a custom RAII wrapper * Fix linear RT width calculation as it's provided in terms of bytes rather than format units * Fix `AllocateStagingBuffer` bug where it would not supply `eTransferDst` as a usage flag * Fix `AllocateMappedImage` where `VkMemoryPropertyFlags` were not respected resulting in non-`eHostVisible` memory being utilized * Change feature requirement in `AndroidManifest.xml` to Vulkan 1.1 from OGL 3.1 as this was incorrect
77 lines
3.4 KiB
C++
77 lines
3.4 KiB
C++
// SPDX-License-Identifier: MPL-2.0
|
|
// Copyright © 2020 Skyline Team and Contributors (https://github.com/skyline-emu/)
|
|
|
|
#include <kernel/types/KProcess.h>
|
|
#include <services/hosbinder/IHOSBinderDriver.h>
|
|
#include "ISelfController.h"
|
|
|
|
namespace skyline::service::am {
|
|
ISelfController::ISelfController(const DeviceState &state, ServiceManager &manager) : libraryAppletLaunchableEvent(std::make_shared<type::KEvent>(state, false)), accumulatedSuspendedTickChangedEvent(std::make_shared<type::KEvent>(state, false)), hosbinder(manager.CreateOrGetService<hosbinder::IHOSBinderDriver>("dispdrv")), BaseService(state, manager) {}
|
|
|
|
Result ISelfController::Exit(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response) {
|
|
if (state.thread->id)
|
|
state.process->Kill(false);
|
|
std::longjmp(state.thread->originalCtx, true);
|
|
return {};
|
|
}
|
|
|
|
Result ISelfController::LockExit(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response) {
|
|
return {};
|
|
}
|
|
|
|
Result ISelfController::UnlockExit(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response) {
|
|
return {};
|
|
}
|
|
|
|
Result ISelfController::GetLibraryAppletLaunchableEvent(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response) {
|
|
libraryAppletLaunchableEvent->Signal();
|
|
|
|
KHandle handle{state.process->InsertItem(libraryAppletLaunchableEvent)};
|
|
state.logger->Debug("Library Applet Launchable Event Handle: 0x{:X}", handle);
|
|
|
|
response.copyHandles.push_back(handle);
|
|
return {};
|
|
}
|
|
|
|
Result ISelfController::SetOperationModeChangedNotification(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response) {
|
|
return {};
|
|
}
|
|
|
|
Result ISelfController::SetPerformanceModeChangedNotification(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response) {
|
|
return {};
|
|
}
|
|
|
|
Result ISelfController::SetFocusHandlingMode(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response) {
|
|
return {};
|
|
}
|
|
|
|
Result ISelfController::SetRestartMessageEnabled(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response) {
|
|
return {};
|
|
}
|
|
|
|
Result ISelfController::SetOutOfFocusSuspendingEnabled(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response) {
|
|
return {};
|
|
}
|
|
|
|
Result ISelfController::CreateManagedDisplayLayer(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response) {
|
|
auto layerId{hosbinder->CreateLayer(hosbinder::DisplayId::Default)};
|
|
state.logger->Debug("Creating Managed Layer #{} on 'Default' Display", layerId);
|
|
response.Push(layerId);
|
|
return {};
|
|
}
|
|
|
|
Result ISelfController::GetAccumulatedSuspendedTickValue(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response) {
|
|
// TODO: Properly handle this after we implement game suspending
|
|
response.Push<u64>(0);
|
|
return {};
|
|
}
|
|
|
|
Result ISelfController::GetAccumulatedSuspendedTickChangedEvent(type::KSession &session, ipc::IpcRequest &request, ipc::IpcResponse &response) {
|
|
auto handle{state.process->InsertItem(accumulatedSuspendedTickChangedEvent)};
|
|
state.logger->Debug("Accumulated Suspended Tick Event Handle: 0x{:X}", handle);
|
|
|
|
response.copyHandles.push_back(handle);
|
|
return {};
|
|
}
|
|
}
|