mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-06-02 18:28:44 +02:00
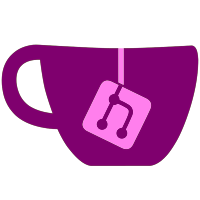
What was added: * Framebuffer * NativeActivity * NV Services * IOCTL Handler * NV Devices: * * /dev/nvmap - 0xC0080101, 0xC0080103, 0xC0200104, 0xC0180105, 0xC00C0109, 0xC008010E * * /dev/nvhost-as-gpu * * /dev/nvhost-channel - 0x40044801, 0xC0104809, 0xC010480B, 0xC018480C, 0x4004480D, 0xC020481A, 0x40084714 * * /dev/nvhost-ctrl * * /dev/nvhost-ctrl-gpu - 0x80044701, 0x80284702, 0xC0184706, 0xC0B04705, 0x80084714 * SVCs: * * SetMemoryAttribute * * CreateTransferMemory * * ResetSignal * * GetSystemTick * Addition of Compact Logger What was fixed: * SVCs: * * SetHeapSize * * SetMemoryAttribute * * QueryMemory * A release build would not set CMAKE_BUILD_TYPE to "RELEASE" * The logger code was simplified
84 lines
3.2 KiB
C++
84 lines
3.2 KiB
C++
#include "common.h"
|
|
#include "nce.h"
|
|
#include "gpu.h"
|
|
#include <tinyxml2.h>
|
|
|
|
namespace skyline {
|
|
Settings::Settings(const std::string &prefXml) {
|
|
tinyxml2::XMLDocument pref;
|
|
if (pref.LoadFile(prefXml.c_str()))
|
|
throw exception("TinyXML2 Error: " + std::string(pref.ErrorStr()));
|
|
tinyxml2::XMLElement *elem = pref.LastChild()->FirstChild()->ToElement();
|
|
while (elem) {
|
|
switch (elem->Value()[0]) {
|
|
case 's':
|
|
stringMap[elem->FindAttribute("name")->Value()] = elem->GetText();
|
|
break;
|
|
case 'b':
|
|
boolMap[elem->FindAttribute("name")->Value()] = elem->FindAttribute("value")->BoolValue();
|
|
break;
|
|
case 'i':
|
|
intMap[elem->FindAttribute("name")->Value()] = elem->FindAttribute("value")->IntValue();
|
|
break;
|
|
default:
|
|
syslog(LOG_ALERT, "Settings type is missing: %s for %s", elem->Value(), elem->FindAttribute("name")->Value());
|
|
break;
|
|
};
|
|
if (elem->NextSibling())
|
|
elem = elem->NextSibling()->ToElement();
|
|
else
|
|
break;
|
|
}
|
|
pref.Clear();
|
|
}
|
|
|
|
std::string Settings::GetString(const std::string &key) {
|
|
return stringMap.at(key);
|
|
}
|
|
|
|
bool Settings::GetBool(const std::string &key) {
|
|
return boolMap.at(key);
|
|
}
|
|
|
|
int Settings::GetInt(const std::string &key) {
|
|
return intMap.at(key);
|
|
}
|
|
|
|
void Settings::List(const std::shared_ptr<Logger> &logger) {
|
|
for (auto &iter : stringMap)
|
|
logger->Info("Key: {}, Value: {}, Type: String", iter.first, GetString(iter.first));
|
|
for (auto &iter : boolMap)
|
|
logger->Info("Key: {}, Value: {}, Type: Bool", iter.first, GetBool(iter.first));
|
|
}
|
|
|
|
Logger::Logger(const std::string &logPath, LogLevel configLevel) : configLevel(configLevel) {
|
|
logFile.open(logPath, std::ios::app);
|
|
WriteHeader("Logging started");
|
|
}
|
|
|
|
Logger::~Logger() {
|
|
WriteHeader("Logging ended");
|
|
}
|
|
|
|
void Logger::WriteHeader(const std::string &str) {
|
|
syslog(LOG_ALERT, "%s", str.c_str());
|
|
logFile << "0|" << str << "\n";
|
|
logFile.flush();
|
|
}
|
|
|
|
void Logger::Write(const LogLevel level, std::string str) {
|
|
syslog(levelSyslog[static_cast<u8>(level)], "%s", str.c_str());
|
|
for (auto &character : str)
|
|
if (character == '\n')
|
|
character = '\\';
|
|
logFile << "1|" << levelStr[static_cast<u8>(level)] << "|" << str << "\n";
|
|
logFile.flush();
|
|
}
|
|
|
|
DeviceState::DeviceState(kernel::OS *os, std::shared_ptr<kernel::type::KProcess> &thisProcess, std::shared_ptr<kernel::type::KThread> &thisThread, ANativeWindow *window, std::shared_ptr<Settings> settings, std::shared_ptr<Logger> logger) : os(os), settings(std::move(settings)), logger(std::move(logger)), thisProcess(thisProcess), thisThread(thisThread) {
|
|
// We assign these later as they may use the state in their constructor and we don't want null pointers
|
|
nce = std::move(std::make_shared<NCE>(*this));
|
|
gpu = std::move(std::make_shared<gpu::GPU>(*this, window));
|
|
}
|
|
}
|