mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-05-28 23:08:46 +02:00
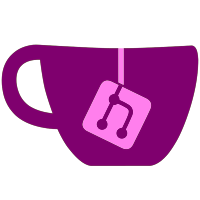
Skyline has been licensed with GPLv3 but as we want to look into optional ads in the future, and that would require linking AdMob SDKs which are proprietary. So, we decided to move to LGPLv3 so we could keep that option open as a revenue stream. We've gathered consent from all contributors for the license change: * PixelyIon - https://github.com/PixelyIon (Commit Author) * Zephyren25 - https://github.com/zephyren25 * ByLaws - https://github.com/bylaws * IvarWithoutBones - https://github.com/IvarWithoutBones * Cyuubi - https://github.com/Cyuubi * greggamesplayer - https://github.com/greggameplayer
91 lines
3.6 KiB
C++
91 lines
3.6 KiB
C++
// SPDX-License-Identifier: LGPL-3.0-or-later
|
|
// Copyright © 2020 Skyline Team and Contributors (https://github.com/skyline-emu/)
|
|
|
|
#pragma once
|
|
|
|
#include <common.h>
|
|
#include <jni.h>
|
|
|
|
namespace skyline {
|
|
/**
|
|
* @brief The JvmManager class is used to simplify transactions with the Java component
|
|
*/
|
|
class JvmManager {
|
|
public:
|
|
JavaVM *vm{}; //!< A pointer to the Java VM
|
|
jobject instance; //!< A reference to the activity
|
|
jclass instanceClass; //!< The class of the activity
|
|
|
|
/**
|
|
* @param env A pointer to the JNI environment
|
|
* @param instance A reference to the activity
|
|
*/
|
|
JvmManager(JNIEnv *env, jobject instance);
|
|
|
|
/**
|
|
* @brief Attach the current thread to the Java VM
|
|
*/
|
|
void AttachThread();
|
|
|
|
/**
|
|
* @brief Detach the current thread to the Java VM
|
|
*/
|
|
void DetachThread();
|
|
|
|
/**
|
|
* @brief Returns a pointer to the JNI environment for the current thread
|
|
*/
|
|
JNIEnv *GetEnv();
|
|
|
|
/**
|
|
* @brief Retrieves a specific field of the given type from the activity
|
|
* @tparam objectType The type of the object in the field
|
|
* @param key The name of the field in the activity class
|
|
* @return The contents of the field as objectType
|
|
*/
|
|
template<typename objectType>
|
|
inline objectType GetField(const char *key) {
|
|
JNIEnv *env = GetEnv();
|
|
if constexpr(std::is_same<objectType, jboolean>())
|
|
return env->GetBooleanField(instance, env->GetFieldID(instanceClass, key, "Z"));
|
|
else if constexpr(std::is_same<objectType, jbyte>())
|
|
return env->GetByteField(instance, env->GetFieldID(instanceClass, key, "B"));
|
|
else if constexpr(std::is_same<objectType, jchar>())
|
|
return env->GetCharField(instance, env->GetFieldID(instanceClass, key, "C"));
|
|
else if constexpr(std::is_same<objectType, jshort>())
|
|
return env->GetShortField(instance, env->GetFieldID(instanceClass, key, "S"));
|
|
else if constexpr(std::is_same<objectType, jint>())
|
|
return env->GetIntField(instance, env->GetFieldID(instanceClass, key, "I"));
|
|
else if constexpr(std::is_same<objectType, jlong>())
|
|
return env->GetLongField(instance, env->GetFieldID(instanceClass, key, "J"));
|
|
else if constexpr(std::is_same<objectType, jfloat>())
|
|
return env->GetFloatField(instance, env->GetFieldID(instanceClass, key, "F"));
|
|
else if constexpr(std::is_same<objectType, jdouble>())
|
|
return env->GetDoubleField(instance, env->GetFieldID(instanceClass, key, "D"));
|
|
}
|
|
|
|
/**
|
|
* @brief Retrieves a specific field from the activity as a jobject
|
|
* @param key The name of the field in the activity class
|
|
* @param signature The signature of the field
|
|
* @return A jobject of the contents of the field
|
|
*/
|
|
jobject GetField(const char *key, const char *signature);
|
|
|
|
/**
|
|
* @brief Checks if a specific field from the activity is null or not
|
|
* @param key The name of the field in the activity class
|
|
* @param signature The signature of the field
|
|
* @return If the field is null or not
|
|
*/
|
|
bool CheckNull(const char *key, const char *signature);
|
|
|
|
/**
|
|
* @brief Checks if a specific jobject is null or not
|
|
* @param object The jobject to check
|
|
* @return If the object is null or not
|
|
*/
|
|
bool CheckNull(jobject &object);
|
|
};
|
|
}
|