mirror of
https://github.com/skyline-emu/skyline.git
synced 2024-05-28 22:38:47 +02:00
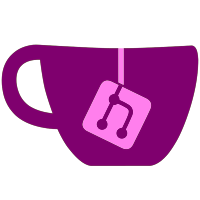
Skyline has been licensed with GPLv3 but as we want to look into optional ads in the future, and that would require linking AdMob SDKs which are proprietary. So, we decided to move to LGPLv3 so we could keep that option open as a revenue stream. We've gathered consent from all contributors for the license change: * PixelyIon - https://github.com/PixelyIon (Commit Author) * Zephyren25 - https://github.com/zephyren25 * ByLaws - https://github.com/bylaws * IvarWithoutBones - https://github.com/IvarWithoutBones * Cyuubi - https://github.com/Cyuubi * greggamesplayer - https://github.com/greggameplayer
41 lines
1.2 KiB
C++
41 lines
1.2 KiB
C++
// SPDX-License-Identifier: LGPL-3.0-or-later
|
|
// Copyright © 2020 Skyline Team and Contributors (https://github.com/skyline-emu/)
|
|
|
|
#pragma once
|
|
|
|
#include <unistd.h>
|
|
#include <os.h>
|
|
#include <kernel/types/KProcess.h>
|
|
|
|
namespace skyline::loader {
|
|
class Loader {
|
|
protected:
|
|
const int romFd; //!< An FD to the ROM file
|
|
|
|
/**
|
|
* @brief Read the file at a particular offset
|
|
* @tparam T The type of object to write to
|
|
* @param output The object to write to
|
|
* @param offset The offset to read the file at
|
|
* @param size The amount to read in bytes
|
|
*/
|
|
template<typename T>
|
|
inline void ReadOffset(T *output, u64 offset, size_t size) {
|
|
pread64(romFd, output, size, offset);
|
|
}
|
|
|
|
public:
|
|
/**
|
|
* @param filePath The path to the ROM file
|
|
*/
|
|
Loader(const int romFd) : romFd(romFd) {}
|
|
|
|
/**
|
|
* This loads in the data of the main process
|
|
* @param process The process to load in the data
|
|
* @param state The state of the device
|
|
*/
|
|
virtual void LoadProcessData(const std::shared_ptr<kernel::type::KProcess> process, const DeviceState &state) = 0;
|
|
};
|
|
}
|